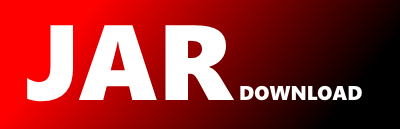
org.apache.river.lookup.util.ConsistentMapEntry Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.river.lookup.util;
import java.io.IOException;
import java.io.Serializable;
import java.util.Map;
import org.apache.river.api.io.AtomicSerial;
import org.apache.river.api.io.AtomicSerial.GetArg;
/**
* An implementation of the java.util.Map.Entry
interface that has
* a serialized form consistent in all virtual machines. ConsistentMapEntry
* instances are unmodifiable. The setValue
mutator method
* throws UnsupportedOperationException
. This class permits null
* for values and keys.
*
*
* Although instances of this class are unmodifiable, they are not necessarily
* immutable. If a client retrieves a mutable object (either a key or value) contained in a
* ConsistentMapEntry
and mutates that object, the client in effect
* mutates the state of the ConsistentMapEntry
. In this case, the
* serialized form of the ConsistentMapEntry
will most likely also
* have been mutated. A ConsistentMapEntry
that contains only immutable
* objects will maintain a consistent serialized form indefinitely. But a
* ConsistentMapEntry
that contains mutable objects will maintain a
* consistent serialized form only so long as the mutable objects are not
* mutated.
*
* @author Bill Venners
*/
@AtomicSerial
final class ConsistentMapEntry implements Map.Entry, Serializable {
private static final long serialVersionUID = -8633627011729114409L;
/**
* @serial An Object
key, or null
*/
private final K key;
/**
* @serial An Object
value, or null
*/
private final V value;
/**
* {@link AtomicSerial} constructor.
* @param arg
* @throws IOException
* @throws ClassCastException if types don't match
*/
public ConsistentMapEntry(GetArg arg) throws IOException {
this((K) arg.get("key", null), (V) arg.get("value", null));
}
/**
* Constructs a new ConsistentMapEntry
with passed
* key
and value
. null
is
* allowed in either (or both) parameters.
*
* @param key the key (null
key is OK)
* @param value the value (null
value is OK) associated with the key
*/
public ConsistentMapEntry(K key, V value) {
this.key = key;
this.value = value;
}
/**
* Returns the key.
*
* @return the key.
*/
@Override
public K getKey() {
return key;
}
/**
* Returns the value.
*
* @return the value.
*/
@Override
public V getValue() {
return value;
}
/**
* Replaces the value corresponding to this entry with the specified value. Because
* all instances of this class are unmodifiable, this method always throws
* UnsupportedOperationException
.
*
* @throws UnsupportedOperationException always
*/
@Override
public V setValue(V value) {
throw new UnsupportedOperationException();
}
/**
* Compares the specified object (the Object
passed
* in o
) with this ConsistentMapEntry
* object for equality. Returns true if the specified object
* is not null
, if the specified object's class is
* ConsistentMapEntry
, if the keys of this object and
* the specified object are either both null
or semantically
* equal, and the values of this object and the specified object are either
* both null
or semantically equal.
*
* @param o the object to compare against
* @return true
if the objects are the semantically equal,
* false
otherwise.
*/
@Override
public boolean equals(Object o) {
if (o == null) {
return false;
}
if (o == this) {
return true;
}
// TODO: ASK JOSH SHOULD EQUALS CHECK FOR INSTANCEOF OR EXACT CLASS?
if (o.getClass() != ConsistentMapEntry.class) {
return false;
}
ConsistentMapEntry unmod = (ConsistentMapEntry) o;
boolean keysEqual = equalsOrNull(key, unmod.key);
boolean valsEqual = equalsOrNull(value, unmod.value);
return keysEqual && valsEqual;
}
private static boolean equalsOrNull(Object o1, Object o2) {
return (o1 == null ? o2 == null : o1.equals(o2));
}
/**
* Returns the hash code value for this ConsistentMapEntry
object.
*
* @return the hashcode for this object
*/
@Override
public int hashCode() {
int keyHash = (key == null ? 0 : key.hashCode());
int valueHash = (value == null ? 0 : value.hashCode());
return keyHash ^ valueHash;
}
}