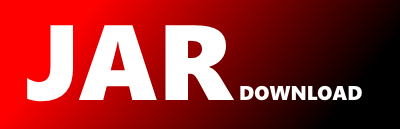
org.apache.river.proxy.ThrowThis Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.river.proxy;
import java.io.IOException;
import java.rmi.RemoteException;
import org.apache.river.api.io.AtomicException;
import org.apache.river.api.io.AtomicSerial;
import org.apache.river.api.io.AtomicSerial.GetArg;
import org.apache.river.api.io.Valid;
/**
* The semi-official way for remote methods on registration objects
* (e.g LeaseRenewalSet
,
* LookupDiscoveryRegistration
,
* MailboxRegistration
) to indicate that the registration
* no longer exists is to throw NoSuchObjectException
.
* Unfortunately if the registration object is implemented as a smart
* proxy that uses RMI to communicate back to the server (instead of a
* straight RMI object) it is not possible for the server to throw
* NoSuchObjectException
directly since RMI will wrap
* such an exception in a ServerException
.
*
* ThrowThis
provides a solution to this problem. When
* the server wants to throw a RemoteException
it creates a
* ThrowThis
object that wraps the
* RemoteException
and then throws the
* ThrowThis
. The proxy catches ThrowThis
* exceptions and in the catch block and calls
* throwRemoteException
to throw the intended exception.
*
* @author Sun Microsystems, Inc.
*
* @see java.rmi.NoSuchObjectException
* @see java.rmi.ServerException
*/
@AtomicSerial
public class ThrowThis extends AtomicException {
private static final long serialVersionUID = 956456443698267251L;
/**
* Exception we want the proxy to throw
* @serial
*/
final private RemoteException toThrow;
/**
* AtomicSerial
* @param arg
* @throws IOException
* @throws java.lang.ClassNotFoundException
*/
public ThrowThis(GetArg arg) throws IOException, ClassNotFoundException{
super(check(arg));
toThrow = arg.get("toThrow", null, RemoteException.class);
}
private static GetArg check(GetArg arg) throws IOException, ClassNotFoundException{
Valid.notNull(arg.get("toThrow", null, RemoteException.class),
"RemoteException cannot be null");
return arg;
}
/**
* Simple constructor
* @param toThrow RemoteException
you proxy to throw
*/
public ThrowThis(RemoteException toThrow) {
super();
this.toThrow = toThrow;
}
/**
* Simple constructor. Note that, in general, objects of this class
* are just a detail of the private protocol between the proxy and
* its server, and are not intended to leak out of a public api.
*
* @param toThrow RemoteException
you proxy to throw
* @param message Message to construct super object with
*/
public ThrowThis(RemoteException toThrow, String message) {
super(message);
this.toThrow = toThrow;
}
/**
* Throw the RemoteException
the server wants thrown
* @throws java.rmi.RemoteException when called.
*/
public void throwRemoteException() throws RemoteException {
throw toThrow;
}
/**
* Returns the detail message, including the message from the nested
* exception the server wants thrown
*/
public String getMessage() {
return super.getMessage() +
"; that is transporting: \n\t" +
toThrow.toString();
}
/**
* Prints the composite message to System.err
.
*/
public void printStackTrace()
{
printStackTrace(System.err);
}
/**
* Prints the composite message and the embedded stack trace to
* the specified print writer pw
* @param pw the print writer
*/
public void printStackTrace(java.io.PrintWriter pw)
{
synchronized(pw) {
pw.println(this);
toThrow.printStackTrace(pw);
}
}
/**
* Prints the composite message and the embedded stack trace to
* the specified stream ps
.
* @param ps the print stream
*/
public void printStackTrace(java.io.PrintStream ps)
{
synchronized(ps) {
ps.println(this);
toThrow.printStackTrace(ps);
}
}
}