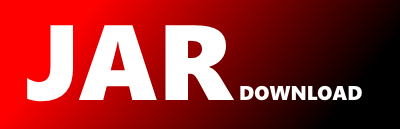
commonMain.aws.sdk.kotlin.services.account.model.PutContactInformationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of account-jvm Show documentation
Show all versions of account-jvm Show documentation
The AWS SDK for Kotlin client for Account
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.account.model
import aws.smithy.kotlin.runtime.SdkDsl
public class PutContactInformationRequest private constructor(builder: Builder) {
/**
* Specifies the 12-digit account ID number of the Amazon Web Services account that you want to access or modify with this operation. If you don't specify this parameter, it defaults to the Amazon Web Services account of the identity used to call the operation. To use this parameter, the caller must be an identity in the [organization's management account](https://docs.aws.amazon.com/organizations/latest/userguide/orgs_getting-started_concepts.html#account) or a delegated administrator account. The specified account ID must be a member account in the same organization. The organization must have [all features enabled](https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_org_support-all-features.html), and the organization must have [trusted access](https://docs.aws.amazon.com/organizations/latest/userguide/using-orgs-trusted-access.html) enabled for the Account Management service, and optionally a [delegated admin](https://docs.aws.amazon.com/organizations/latest/userguide/using-orgs-delegated-admin.html) account assigned.
*
* The management account can't specify its own `AccountId`. It must call the operation in standalone context by not including the `AccountId` parameter.
*
* To call this operation on an account that is not a member of an organization, don't specify this parameter. Instead, call the operation using an identity belonging to the account whose contacts you wish to retrieve or modify.
*/
public val accountId: kotlin.String? = builder.accountId
/**
* Contains the details of the primary contact information associated with an Amazon Web Services account.
*/
public val contactInformation: aws.sdk.kotlin.services.account.model.ContactInformation? = builder.contactInformation
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.account.model.PutContactInformationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutContactInformationRequest(")
append("accountId=$accountId,")
append("contactInformation=$contactInformation")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accountId?.hashCode() ?: 0
result = 31 * result + (contactInformation?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutContactInformationRequest
if (accountId != other.accountId) return false
if (contactInformation != other.contactInformation) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.account.model.PutContactInformationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the 12-digit account ID number of the Amazon Web Services account that you want to access or modify with this operation. If you don't specify this parameter, it defaults to the Amazon Web Services account of the identity used to call the operation. To use this parameter, the caller must be an identity in the [organization's management account](https://docs.aws.amazon.com/organizations/latest/userguide/orgs_getting-started_concepts.html#account) or a delegated administrator account. The specified account ID must be a member account in the same organization. The organization must have [all features enabled](https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_org_support-all-features.html), and the organization must have [trusted access](https://docs.aws.amazon.com/organizations/latest/userguide/using-orgs-trusted-access.html) enabled for the Account Management service, and optionally a [delegated admin](https://docs.aws.amazon.com/organizations/latest/userguide/using-orgs-delegated-admin.html) account assigned.
*
* The management account can't specify its own `AccountId`. It must call the operation in standalone context by not including the `AccountId` parameter.
*
* To call this operation on an account that is not a member of an organization, don't specify this parameter. Instead, call the operation using an identity belonging to the account whose contacts you wish to retrieve or modify.
*/
public var accountId: kotlin.String? = null
/**
* Contains the details of the primary contact information associated with an Amazon Web Services account.
*/
public var contactInformation: aws.sdk.kotlin.services.account.model.ContactInformation? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.account.model.PutContactInformationRequest) : this() {
this.accountId = x.accountId
this.contactInformation = x.contactInformation
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.account.model.PutContactInformationRequest = PutContactInformationRequest(this)
/**
* construct an [aws.sdk.kotlin.services.account.model.ContactInformation] inside the given [block]
*/
public fun contactInformation(block: aws.sdk.kotlin.services.account.model.ContactInformation.Builder.() -> kotlin.Unit) {
this.contactInformation = aws.sdk.kotlin.services.account.model.ContactInformation.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy