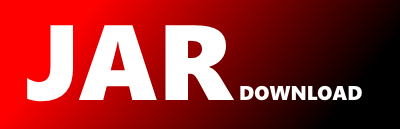
aws.sdk.kotlin.services.amplify.model.CreateBranchRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.amplify.model
/**
* The request structure for the create branch request.
*/
class CreateBranchRequest private constructor(builder: Builder) {
/**
* The unique ID for an Amplify app.
*/
val appId: kotlin.String? = builder.appId
/**
* The Amazon Resource Name (ARN) for a backend environment that is part of an Amplify
* app.
*/
val backendEnvironmentArn: kotlin.String? = builder.backendEnvironmentArn
/**
* The basic authorization credentials for the branch.
*/
val basicAuthCredentials: kotlin.String? = builder.basicAuthCredentials
/**
* The name for the branch.
*/
val branchName: kotlin.String? = builder.branchName
/**
* The build specification (build spec) for the branch.
*/
val buildSpec: kotlin.String? = builder.buildSpec
/**
* The description for the branch.
*/
val description: kotlin.String? = builder.description
/**
* The display name for a branch. This is used as the default domain prefix.
*/
val displayName: kotlin.String? = builder.displayName
/**
* Enables auto building for the branch.
*/
val enableAutoBuild: kotlin.Boolean? = builder.enableAutoBuild
/**
* Enables basic authorization for the branch.
*/
val enableBasicAuth: kotlin.Boolean? = builder.enableBasicAuth
/**
* Enables notifications for the branch.
*/
val enableNotification: kotlin.Boolean? = builder.enableNotification
/**
* Enables performance mode for the branch.
* Performance mode optimizes for faster hosting performance by keeping content cached at the edge for a longer interval. When performance mode is enabled, hosting configuration or code changes can take up to 10 minutes to roll out.
*/
val enablePerformanceMode: kotlin.Boolean? = builder.enablePerformanceMode
/**
* Enables pull request previews for this branch.
*/
val enablePullRequestPreview: kotlin.Boolean? = builder.enablePullRequestPreview
/**
* The environment variables for the branch.
*/
val environmentVariables: Map? = builder.environmentVariables
/**
* The framework for the branch.
*/
val framework: kotlin.String? = builder.framework
/**
* The Amplify environment name for the pull request.
*/
val pullRequestEnvironmentName: kotlin.String? = builder.pullRequestEnvironmentName
/**
* Describes the current stage for the branch.
*/
val stage: aws.sdk.kotlin.services.amplify.model.Stage? = builder.stage
/**
* The tag for the branch.
*/
val tags: Map? = builder.tags
/**
* The content Time To Live (TTL) for the website in seconds.
*/
val ttl: kotlin.String? = builder.ttl
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.amplify.model.CreateBranchRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateBranchRequest(")
append("appId=$appId,")
append("backendEnvironmentArn=$backendEnvironmentArn,")
append("basicAuthCredentials=*** Sensitive Data Redacted ***,")
append("branchName=$branchName,")
append("buildSpec=$buildSpec,")
append("description=$description,")
append("displayName=$displayName,")
append("enableAutoBuild=$enableAutoBuild,")
append("enableBasicAuth=$enableBasicAuth,")
append("enableNotification=$enableNotification,")
append("enablePerformanceMode=$enablePerformanceMode,")
append("enablePullRequestPreview=$enablePullRequestPreview,")
append("environmentVariables=$environmentVariables,")
append("framework=$framework,")
append("pullRequestEnvironmentName=$pullRequestEnvironmentName,")
append("stage=$stage,")
append("tags=$tags,")
append("ttl=$ttl)")
}
override fun hashCode(): kotlin.Int {
var result = appId?.hashCode() ?: 0
result = 31 * result + (backendEnvironmentArn?.hashCode() ?: 0)
result = 31 * result + (basicAuthCredentials?.hashCode() ?: 0)
result = 31 * result + (branchName?.hashCode() ?: 0)
result = 31 * result + (buildSpec?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (displayName?.hashCode() ?: 0)
result = 31 * result + (enableAutoBuild?.hashCode() ?: 0)
result = 31 * result + (enableBasicAuth?.hashCode() ?: 0)
result = 31 * result + (enableNotification?.hashCode() ?: 0)
result = 31 * result + (enablePerformanceMode?.hashCode() ?: 0)
result = 31 * result + (enablePullRequestPreview?.hashCode() ?: 0)
result = 31 * result + (environmentVariables?.hashCode() ?: 0)
result = 31 * result + (framework?.hashCode() ?: 0)
result = 31 * result + (pullRequestEnvironmentName?.hashCode() ?: 0)
result = 31 * result + (stage?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (ttl?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateBranchRequest
if (appId != other.appId) return false
if (backendEnvironmentArn != other.backendEnvironmentArn) return false
if (basicAuthCredentials != other.basicAuthCredentials) return false
if (branchName != other.branchName) return false
if (buildSpec != other.buildSpec) return false
if (description != other.description) return false
if (displayName != other.displayName) return false
if (enableAutoBuild != other.enableAutoBuild) return false
if (enableBasicAuth != other.enableBasicAuth) return false
if (enableNotification != other.enableNotification) return false
if (enablePerformanceMode != other.enablePerformanceMode) return false
if (enablePullRequestPreview != other.enablePullRequestPreview) return false
if (environmentVariables != other.environmentVariables) return false
if (framework != other.framework) return false
if (pullRequestEnvironmentName != other.pullRequestEnvironmentName) return false
if (stage != other.stage) return false
if (tags != other.tags) return false
if (ttl != other.ttl) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.amplify.model.CreateBranchRequest = Builder(this).apply(block).build()
class Builder {
/**
* The unique ID for an Amplify app.
*/
var appId: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) for a backend environment that is part of an Amplify
* app.
*/
var backendEnvironmentArn: kotlin.String? = null
/**
* The basic authorization credentials for the branch.
*/
var basicAuthCredentials: kotlin.String? = null
/**
* The name for the branch.
*/
var branchName: kotlin.String? = null
/**
* The build specification (build spec) for the branch.
*/
var buildSpec: kotlin.String? = null
/**
* The description for the branch.
*/
var description: kotlin.String? = null
/**
* The display name for a branch. This is used as the default domain prefix.
*/
var displayName: kotlin.String? = null
/**
* Enables auto building for the branch.
*/
var enableAutoBuild: kotlin.Boolean? = null
/**
* Enables basic authorization for the branch.
*/
var enableBasicAuth: kotlin.Boolean? = null
/**
* Enables notifications for the branch.
*/
var enableNotification: kotlin.Boolean? = null
/**
* Enables performance mode for the branch.
* Performance mode optimizes for faster hosting performance by keeping content cached at the edge for a longer interval. When performance mode is enabled, hosting configuration or code changes can take up to 10 minutes to roll out.
*/
var enablePerformanceMode: kotlin.Boolean? = null
/**
* Enables pull request previews for this branch.
*/
var enablePullRequestPreview: kotlin.Boolean? = null
/**
* The environment variables for the branch.
*/
var environmentVariables: Map? = null
/**
* The framework for the branch.
*/
var framework: kotlin.String? = null
/**
* The Amplify environment name for the pull request.
*/
var pullRequestEnvironmentName: kotlin.String? = null
/**
* Describes the current stage for the branch.
*/
var stage: aws.sdk.kotlin.services.amplify.model.Stage? = null
/**
* The tag for the branch.
*/
var tags: Map? = null
/**
* The content Time To Live (TTL) for the website in seconds.
*/
var ttl: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.amplify.model.CreateBranchRequest) : this() {
this.appId = x.appId
this.backendEnvironmentArn = x.backendEnvironmentArn
this.basicAuthCredentials = x.basicAuthCredentials
this.branchName = x.branchName
this.buildSpec = x.buildSpec
this.description = x.description
this.displayName = x.displayName
this.enableAutoBuild = x.enableAutoBuild
this.enableBasicAuth = x.enableBasicAuth
this.enableNotification = x.enableNotification
this.enablePerformanceMode = x.enablePerformanceMode
this.enablePullRequestPreview = x.enablePullRequestPreview
this.environmentVariables = x.environmentVariables
this.framework = x.framework
this.pullRequestEnvironmentName = x.pullRequestEnvironmentName
this.stage = x.stage
this.tags = x.tags
this.ttl = x.ttl
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.amplify.model.CreateBranchRequest = CreateBranchRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy