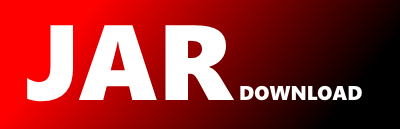
aws.sdk.kotlin.services.amplify.model.UpdateAppRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.amplify.model
/**
* The request structure for the update app request.
*/
class UpdateAppRequest private constructor(builder: Builder) {
/**
* The personal access token for a third-party source control system for an Amplify app.
* The token is used to create webhook and a read-only deploy key. The token is not stored.
*/
val accessToken: kotlin.String? = builder.accessToken
/**
* The unique ID for an Amplify app.
*/
val appId: kotlin.String? = builder.appId
/**
* The automated branch creation configuration for an Amplify app.
*/
val autoBranchCreationConfig: aws.sdk.kotlin.services.amplify.model.AutoBranchCreationConfig? = builder.autoBranchCreationConfig
/**
* Describes the automated branch creation glob patterns for an Amplify app.
*/
val autoBranchCreationPatterns: List? = builder.autoBranchCreationPatterns
/**
* The basic authorization credentials for an Amplify app.
*/
val basicAuthCredentials: kotlin.String? = builder.basicAuthCredentials
/**
* The build specification (build spec) for an Amplify app.
*/
val buildSpec: kotlin.String? = builder.buildSpec
/**
* The custom HTTP headers for an Amplify app.
*/
val customHeaders: kotlin.String? = builder.customHeaders
/**
* The custom redirect and rewrite rules for an Amplify app.
*/
val customRules: List? = builder.customRules
/**
* The description for an Amplify app.
*/
val description: kotlin.String? = builder.description
/**
* Enables automated branch creation for an Amplify app.
*/
val enableAutoBranchCreation: kotlin.Boolean? = builder.enableAutoBranchCreation
/**
* Enables basic authorization for an Amplify app.
*/
val enableBasicAuth: kotlin.Boolean? = builder.enableBasicAuth
/**
* Enables branch auto-building for an Amplify app.
*/
val enableBranchAutoBuild: kotlin.Boolean? = builder.enableBranchAutoBuild
/**
* Automatically disconnects a branch in the Amplify Console when you delete a branch
* from your Git repository.
*/
val enableBranchAutoDeletion: kotlin.Boolean? = builder.enableBranchAutoDeletion
/**
* The environment variables for an Amplify app.
*/
val environmentVariables: Map? = builder.environmentVariables
/**
* The AWS Identity and Access Management (IAM) service role for an Amplify app.
*/
val iamServiceRoleArn: kotlin.String? = builder.iamServiceRoleArn
/**
* The name for an Amplify app.
*/
val name: kotlin.String? = builder.name
/**
* The OAuth token for a third-party source control system for an Amplify app. The token
* is used to create a webhook and a read-only deploy key. The OAuth token is not stored.
*/
val oauthToken: kotlin.String? = builder.oauthToken
/**
* The platform for an Amplify app.
*/
val platform: aws.sdk.kotlin.services.amplify.model.Platform? = builder.platform
/**
* The name of the repository for an Amplify app
*/
val repository: kotlin.String? = builder.repository
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.amplify.model.UpdateAppRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateAppRequest(")
append("accessToken=*** Sensitive Data Redacted ***,")
append("appId=$appId,")
append("autoBranchCreationConfig=$autoBranchCreationConfig,")
append("autoBranchCreationPatterns=$autoBranchCreationPatterns,")
append("basicAuthCredentials=*** Sensitive Data Redacted ***,")
append("buildSpec=$buildSpec,")
append("customHeaders=$customHeaders,")
append("customRules=$customRules,")
append("description=$description,")
append("enableAutoBranchCreation=$enableAutoBranchCreation,")
append("enableBasicAuth=$enableBasicAuth,")
append("enableBranchAutoBuild=$enableBranchAutoBuild,")
append("enableBranchAutoDeletion=$enableBranchAutoDeletion,")
append("environmentVariables=$environmentVariables,")
append("iamServiceRoleArn=$iamServiceRoleArn,")
append("name=$name,")
append("oauthToken=*** Sensitive Data Redacted ***,")
append("platform=$platform,")
append("repository=$repository)")
}
override fun hashCode(): kotlin.Int {
var result = accessToken?.hashCode() ?: 0
result = 31 * result + (appId?.hashCode() ?: 0)
result = 31 * result + (autoBranchCreationConfig?.hashCode() ?: 0)
result = 31 * result + (autoBranchCreationPatterns?.hashCode() ?: 0)
result = 31 * result + (basicAuthCredentials?.hashCode() ?: 0)
result = 31 * result + (buildSpec?.hashCode() ?: 0)
result = 31 * result + (customHeaders?.hashCode() ?: 0)
result = 31 * result + (customRules?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (enableAutoBranchCreation?.hashCode() ?: 0)
result = 31 * result + (enableBasicAuth?.hashCode() ?: 0)
result = 31 * result + (enableBranchAutoBuild?.hashCode() ?: 0)
result = 31 * result + (enableBranchAutoDeletion?.hashCode() ?: 0)
result = 31 * result + (environmentVariables?.hashCode() ?: 0)
result = 31 * result + (iamServiceRoleArn?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (oauthToken?.hashCode() ?: 0)
result = 31 * result + (platform?.hashCode() ?: 0)
result = 31 * result + (repository?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateAppRequest
if (accessToken != other.accessToken) return false
if (appId != other.appId) return false
if (autoBranchCreationConfig != other.autoBranchCreationConfig) return false
if (autoBranchCreationPatterns != other.autoBranchCreationPatterns) return false
if (basicAuthCredentials != other.basicAuthCredentials) return false
if (buildSpec != other.buildSpec) return false
if (customHeaders != other.customHeaders) return false
if (customRules != other.customRules) return false
if (description != other.description) return false
if (enableAutoBranchCreation != other.enableAutoBranchCreation) return false
if (enableBasicAuth != other.enableBasicAuth) return false
if (enableBranchAutoBuild != other.enableBranchAutoBuild) return false
if (enableBranchAutoDeletion != other.enableBranchAutoDeletion) return false
if (environmentVariables != other.environmentVariables) return false
if (iamServiceRoleArn != other.iamServiceRoleArn) return false
if (name != other.name) return false
if (oauthToken != other.oauthToken) return false
if (platform != other.platform) return false
if (repository != other.repository) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.amplify.model.UpdateAppRequest = Builder(this).apply(block).build()
class Builder {
/**
* The personal access token for a third-party source control system for an Amplify app.
* The token is used to create webhook and a read-only deploy key. The token is not stored.
*/
var accessToken: kotlin.String? = null
/**
* The unique ID for an Amplify app.
*/
var appId: kotlin.String? = null
/**
* The automated branch creation configuration for an Amplify app.
*/
var autoBranchCreationConfig: aws.sdk.kotlin.services.amplify.model.AutoBranchCreationConfig? = null
/**
* Describes the automated branch creation glob patterns for an Amplify app.
*/
var autoBranchCreationPatterns: List? = null
/**
* The basic authorization credentials for an Amplify app.
*/
var basicAuthCredentials: kotlin.String? = null
/**
* The build specification (build spec) for an Amplify app.
*/
var buildSpec: kotlin.String? = null
/**
* The custom HTTP headers for an Amplify app.
*/
var customHeaders: kotlin.String? = null
/**
* The custom redirect and rewrite rules for an Amplify app.
*/
var customRules: List? = null
/**
* The description for an Amplify app.
*/
var description: kotlin.String? = null
/**
* Enables automated branch creation for an Amplify app.
*/
var enableAutoBranchCreation: kotlin.Boolean? = null
/**
* Enables basic authorization for an Amplify app.
*/
var enableBasicAuth: kotlin.Boolean? = null
/**
* Enables branch auto-building for an Amplify app.
*/
var enableBranchAutoBuild: kotlin.Boolean? = null
/**
* Automatically disconnects a branch in the Amplify Console when you delete a branch
* from your Git repository.
*/
var enableBranchAutoDeletion: kotlin.Boolean? = null
/**
* The environment variables for an Amplify app.
*/
var environmentVariables: Map? = null
/**
* The AWS Identity and Access Management (IAM) service role for an Amplify app.
*/
var iamServiceRoleArn: kotlin.String? = null
/**
* The name for an Amplify app.
*/
var name: kotlin.String? = null
/**
* The OAuth token for a third-party source control system for an Amplify app. The token
* is used to create a webhook and a read-only deploy key. The OAuth token is not stored.
*/
var oauthToken: kotlin.String? = null
/**
* The platform for an Amplify app.
*/
var platform: aws.sdk.kotlin.services.amplify.model.Platform? = null
/**
* The name of the repository for an Amplify app
*/
var repository: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.amplify.model.UpdateAppRequest) : this() {
this.accessToken = x.accessToken
this.appId = x.appId
this.autoBranchCreationConfig = x.autoBranchCreationConfig
this.autoBranchCreationPatterns = x.autoBranchCreationPatterns
this.basicAuthCredentials = x.basicAuthCredentials
this.buildSpec = x.buildSpec
this.customHeaders = x.customHeaders
this.customRules = x.customRules
this.description = x.description
this.enableAutoBranchCreation = x.enableAutoBranchCreation
this.enableBasicAuth = x.enableBasicAuth
this.enableBranchAutoBuild = x.enableBranchAutoBuild
this.enableBranchAutoDeletion = x.enableBranchAutoDeletion
this.environmentVariables = x.environmentVariables
this.iamServiceRoleArn = x.iamServiceRoleArn
this.name = x.name
this.oauthToken = x.oauthToken
this.platform = x.platform
this.repository = x.repository
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.amplify.model.UpdateAppRequest = UpdateAppRequest(this)
/**
* construct an [aws.sdk.kotlin.services.amplify.model.AutoBranchCreationConfig] inside the given [block]
*/
fun autoBranchCreationConfig(block: aws.sdk.kotlin.services.amplify.model.AutoBranchCreationConfig.Builder.() -> kotlin.Unit) {
this.autoBranchCreationConfig = aws.sdk.kotlin.services.amplify.model.AutoBranchCreationConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy