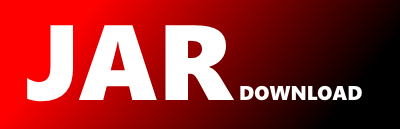
commonMain.aws.sdk.kotlin.services.appconfig.model.ConfigurationProfileSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A summary of a configuration profile.
*/
public class ConfigurationProfileSummary private constructor(builder: Builder) {
/**
* The application ID.
*/
public val applicationId: kotlin.String? = builder.applicationId
/**
* The ID of the configuration profile.
*/
public val id: kotlin.String? = builder.id
/**
* The URI location of the configuration.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* The name of the configuration profile.
*/
public val name: kotlin.String? = builder.name
/**
* The type of configurations contained in the profile. AppConfig supports `feature flags` and `freeform` configurations. We recommend you create feature flag configurations to enable or disable new features and freeform configurations to distribute configurations to an application. When calling this API, enter one of the following values for `Type`:
*
* `AWS.AppConfig.FeatureFlags`
*
* `AWS.Freeform`
*/
public val type: kotlin.String? = builder.type
/**
* The types of validators in the configuration profile.
*/
public val validatorTypes: List? = builder.validatorTypes
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.ConfigurationProfileSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConfigurationProfileSummary(")
append("applicationId=$applicationId,")
append("id=$id,")
append("locationUri=$locationUri,")
append("name=$name,")
append("type=$type,")
append("validatorTypes=$validatorTypes")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationId?.hashCode() ?: 0
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (validatorTypes?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConfigurationProfileSummary
if (applicationId != other.applicationId) return false
if (id != other.id) return false
if (locationUri != other.locationUri) return false
if (name != other.name) return false
if (type != other.type) return false
if (validatorTypes != other.validatorTypes) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.ConfigurationProfileSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The application ID.
*/
public var applicationId: kotlin.String? = null
/**
* The ID of the configuration profile.
*/
public var id: kotlin.String? = null
/**
* The URI location of the configuration.
*/
public var locationUri: kotlin.String? = null
/**
* The name of the configuration profile.
*/
public var name: kotlin.String? = null
/**
* The type of configurations contained in the profile. AppConfig supports `feature flags` and `freeform` configurations. We recommend you create feature flag configurations to enable or disable new features and freeform configurations to distribute configurations to an application. When calling this API, enter one of the following values for `Type`:
*
* `AWS.AppConfig.FeatureFlags`
*
* `AWS.Freeform`
*/
public var type: kotlin.String? = null
/**
* The types of validators in the configuration profile.
*/
public var validatorTypes: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.ConfigurationProfileSummary) : this() {
this.applicationId = x.applicationId
this.id = x.id
this.locationUri = x.locationUri
this.name = x.name
this.type = x.type
this.validatorTypes = x.validatorTypes
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.ConfigurationProfileSummary = ConfigurationProfileSummary(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy