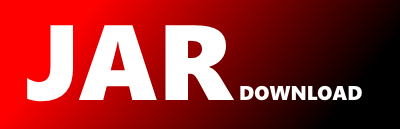
commonMain.aws.sdk.kotlin.services.appconfig.model.CreateExtensionAssociationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateExtensionAssociationRequest private constructor(builder: Builder) {
/**
* The name, the ID, or the Amazon Resource Name (ARN) of the extension.
*/
public val extensionIdentifier: kotlin.String? = builder.extensionIdentifier
/**
* The version number of the extension. If not specified, AppConfig uses the maximum version of the extension.
*/
public val extensionVersionNumber: kotlin.Int? = builder.extensionVersionNumber
/**
* The parameter names and values defined in the extensions. Extension parameters marked `Required` must be entered for this field.
*/
public val parameters: Map? = builder.parameters
/**
* The ARN of an application, configuration profile, or environment.
*/
public val resourceIdentifier: kotlin.String? = builder.resourceIdentifier
/**
* Adds one or more tags for the specified extension association. Tags are metadata that help you categorize resources in different ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you define.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.CreateExtensionAssociationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateExtensionAssociationRequest(")
append("extensionIdentifier=$extensionIdentifier,")
append("extensionVersionNumber=$extensionVersionNumber,")
append("parameters=$parameters,")
append("resourceIdentifier=$resourceIdentifier,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = extensionIdentifier?.hashCode() ?: 0
result = 31 * result + (extensionVersionNumber ?: 0)
result = 31 * result + (parameters?.hashCode() ?: 0)
result = 31 * result + (resourceIdentifier?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateExtensionAssociationRequest
if (extensionIdentifier != other.extensionIdentifier) return false
if (extensionVersionNumber != other.extensionVersionNumber) return false
if (parameters != other.parameters) return false
if (resourceIdentifier != other.resourceIdentifier) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.CreateExtensionAssociationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The name, the ID, or the Amazon Resource Name (ARN) of the extension.
*/
public var extensionIdentifier: kotlin.String? = null
/**
* The version number of the extension. If not specified, AppConfig uses the maximum version of the extension.
*/
public var extensionVersionNumber: kotlin.Int? = null
/**
* The parameter names and values defined in the extensions. Extension parameters marked `Required` must be entered for this field.
*/
public var parameters: Map? = null
/**
* The ARN of an application, configuration profile, or environment.
*/
public var resourceIdentifier: kotlin.String? = null
/**
* Adds one or more tags for the specified extension association. Tags are metadata that help you categorize resources in different ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you define.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.CreateExtensionAssociationRequest) : this() {
this.extensionIdentifier = x.extensionIdentifier
this.extensionVersionNumber = x.extensionVersionNumber
this.parameters = x.parameters
this.resourceIdentifier = x.resourceIdentifier
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.CreateExtensionAssociationRequest = CreateExtensionAssociationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy