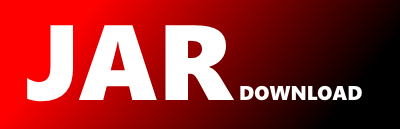
commonMain.aws.sdk.kotlin.services.appconfig.model.CreateExtensionRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateExtensionRequest private constructor(builder: Builder) {
/**
* The actions defined in the extension.
*/
public val actions: Map>? = builder.actions
/**
* Information about the extension.
*/
public val description: kotlin.String? = builder.description
/**
* You can omit this field when you create an extension. When you create a new version, specify the most recent current version number. For example, you create version 3, enter 2 for this field.
*/
public val latestVersionNumber: kotlin.Int? = builder.latestVersionNumber
/**
* A name for the extension. Each extension name in your account must be unique. Extension versions use the same name.
*/
public val name: kotlin.String? = builder.name
/**
* The parameters accepted by the extension. You specify parameter values when you associate the extension to an AppConfig resource by using the `CreateExtensionAssociation` API action. For Lambda extension actions, these parameters are included in the Lambda request object.
*/
public val parameters: Map? = builder.parameters
/**
* Adds one or more tags for the specified extension. Tags are metadata that help you categorize resources in different ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you define.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.CreateExtensionRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateExtensionRequest(")
append("actions=$actions,")
append("description=$description,")
append("latestVersionNumber=$latestVersionNumber,")
append("name=$name,")
append("parameters=$parameters,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actions?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (latestVersionNumber ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (parameters?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateExtensionRequest
if (actions != other.actions) return false
if (description != other.description) return false
if (latestVersionNumber != other.latestVersionNumber) return false
if (name != other.name) return false
if (parameters != other.parameters) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.CreateExtensionRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The actions defined in the extension.
*/
public var actions: Map>? = null
/**
* Information about the extension.
*/
public var description: kotlin.String? = null
/**
* You can omit this field when you create an extension. When you create a new version, specify the most recent current version number. For example, you create version 3, enter 2 for this field.
*/
public var latestVersionNumber: kotlin.Int? = null
/**
* A name for the extension. Each extension name in your account must be unique. Extension versions use the same name.
*/
public var name: kotlin.String? = null
/**
* The parameters accepted by the extension. You specify parameter values when you associate the extension to an AppConfig resource by using the `CreateExtensionAssociation` API action. For Lambda extension actions, these parameters are included in the Lambda request object.
*/
public var parameters: Map? = null
/**
* Adds one or more tags for the specified extension. Tags are metadata that help you categorize resources in different ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you define.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.CreateExtensionRequest) : this() {
this.actions = x.actions
this.description = x.description
this.latestVersionNumber = x.latestVersionNumber
this.name = x.name
this.parameters = x.parameters
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.CreateExtensionRequest = CreateExtensionRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy