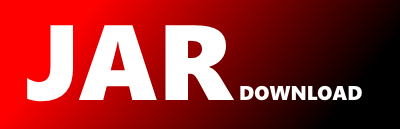
commonMain.aws.sdk.kotlin.services.appconfig.model.GetConfigurationProfileResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetConfigurationProfileResponse private constructor(builder: Builder) {
/**
* The application ID.
*/
public val applicationId: kotlin.String? = builder.applicationId
/**
* The configuration profile description.
*/
public val description: kotlin.String? = builder.description
/**
* The configuration profile ID.
*/
public val id: kotlin.String? = builder.id
/**
* The Amazon Resource Name of the Key Management Service key to encrypt new configuration data versions in the AppConfig hosted configuration store. This attribute is only used for `hosted` configuration types. To encrypt data managed in other configuration stores, see the documentation for how to specify an KMS key for that particular service.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created or updated.
*/
public val kmsKeyIdentifier: kotlin.String? = builder.kmsKeyIdentifier
/**
* The URI location of the configuration.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* The name of the configuration profile.
*/
public val name: kotlin.String? = builder.name
/**
* The ARN of an IAM role with permission to access the configuration at the specified `LocationUri`.
*/
public val retrievalRoleArn: kotlin.String? = builder.retrievalRoleArn
/**
* The type of configurations contained in the profile. AppConfig supports `feature flags` and `freeform` configurations. We recommend you create feature flag configurations to enable or disable new features and freeform configurations to distribute configurations to an application. When calling this API, enter one of the following values for `Type`:
*
* `AWS.AppConfig.FeatureFlags`
*
* `AWS.Freeform`
*/
public val type: kotlin.String? = builder.type
/**
* A list of methods for validating the configuration.
*/
public val validators: List? = builder.validators
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.GetConfigurationProfileResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetConfigurationProfileResponse(")
append("applicationId=$applicationId,")
append("description=$description,")
append("id=$id,")
append("kmsKeyArn=$kmsKeyArn,")
append("kmsKeyIdentifier=$kmsKeyIdentifier,")
append("locationUri=$locationUri,")
append("name=$name,")
append("retrievalRoleArn=$retrievalRoleArn,")
append("type=$type,")
append("validators=$validators")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationId?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (kmsKeyIdentifier?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (retrievalRoleArn?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (validators?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetConfigurationProfileResponse
if (applicationId != other.applicationId) return false
if (description != other.description) return false
if (id != other.id) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (kmsKeyIdentifier != other.kmsKeyIdentifier) return false
if (locationUri != other.locationUri) return false
if (name != other.name) return false
if (retrievalRoleArn != other.retrievalRoleArn) return false
if (type != other.type) return false
if (validators != other.validators) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.GetConfigurationProfileResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The application ID.
*/
public var applicationId: kotlin.String? = null
/**
* The configuration profile description.
*/
public var description: kotlin.String? = null
/**
* The configuration profile ID.
*/
public var id: kotlin.String? = null
/**
* The Amazon Resource Name of the Key Management Service key to encrypt new configuration data versions in the AppConfig hosted configuration store. This attribute is only used for `hosted` configuration types. To encrypt data managed in other configuration stores, see the documentation for how to specify an KMS key for that particular service.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created or updated.
*/
public var kmsKeyIdentifier: kotlin.String? = null
/**
* The URI location of the configuration.
*/
public var locationUri: kotlin.String? = null
/**
* The name of the configuration profile.
*/
public var name: kotlin.String? = null
/**
* The ARN of an IAM role with permission to access the configuration at the specified `LocationUri`.
*/
public var retrievalRoleArn: kotlin.String? = null
/**
* The type of configurations contained in the profile. AppConfig supports `feature flags` and `freeform` configurations. We recommend you create feature flag configurations to enable or disable new features and freeform configurations to distribute configurations to an application. When calling this API, enter one of the following values for `Type`:
*
* `AWS.AppConfig.FeatureFlags`
*
* `AWS.Freeform`
*/
public var type: kotlin.String? = null
/**
* A list of methods for validating the configuration.
*/
public var validators: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.GetConfigurationProfileResponse) : this() {
this.applicationId = x.applicationId
this.description = x.description
this.id = x.id
this.kmsKeyArn = x.kmsKeyArn
this.kmsKeyIdentifier = x.kmsKeyIdentifier
this.locationUri = x.locationUri
this.name = x.name
this.retrievalRoleArn = x.retrievalRoleArn
this.type = x.type
this.validators = x.validators
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.GetConfigurationProfileResponse = GetConfigurationProfileResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy