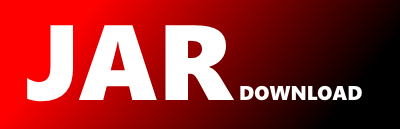
commonMain.aws.sdk.kotlin.services.appconfig.model.StartDeploymentRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
public class StartDeploymentRequest private constructor(builder: Builder) {
/**
* The application ID.
*/
public val applicationId: kotlin.String? = builder.applicationId
/**
* The configuration profile ID.
*/
public val configurationProfileId: kotlin.String? = builder.configurationProfileId
/**
* The configuration version to deploy. If deploying an AppConfig hosted configuration version, you can specify either the version number or version label. For all other configurations, you must specify the version number.
*/
public val configurationVersion: kotlin.String? = builder.configurationVersion
/**
* The deployment strategy ID.
*/
public val deploymentStrategyId: kotlin.String? = builder.deploymentStrategyId
/**
* A description of the deployment.
*/
public val description: kotlin.String? = builder.description
/**
* A map of dynamic extension parameter names to values to pass to associated extensions with `PRE_START_DEPLOYMENT` actions.
*/
public val dynamicExtensionParameters: Map? = builder.dynamicExtensionParameters
/**
* The environment ID.
*/
public val environmentId: kotlin.String? = builder.environmentId
/**
* The KMS key identifier (key ID, key alias, or key ARN). AppConfig uses this ID to encrypt the configuration data using a customer managed key.
*/
public val kmsKeyIdentifier: kotlin.String? = builder.kmsKeyIdentifier
/**
* Metadata to assign to the deployment. Tags help organize and categorize your AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.StartDeploymentRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartDeploymentRequest(")
append("applicationId=$applicationId,")
append("configurationProfileId=$configurationProfileId,")
append("configurationVersion=$configurationVersion,")
append("deploymentStrategyId=$deploymentStrategyId,")
append("description=$description,")
append("dynamicExtensionParameters=$dynamicExtensionParameters,")
append("environmentId=$environmentId,")
append("kmsKeyIdentifier=$kmsKeyIdentifier,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationId?.hashCode() ?: 0
result = 31 * result + (configurationProfileId?.hashCode() ?: 0)
result = 31 * result + (configurationVersion?.hashCode() ?: 0)
result = 31 * result + (deploymentStrategyId?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (dynamicExtensionParameters?.hashCode() ?: 0)
result = 31 * result + (environmentId?.hashCode() ?: 0)
result = 31 * result + (kmsKeyIdentifier?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartDeploymentRequest
if (applicationId != other.applicationId) return false
if (configurationProfileId != other.configurationProfileId) return false
if (configurationVersion != other.configurationVersion) return false
if (deploymentStrategyId != other.deploymentStrategyId) return false
if (description != other.description) return false
if (dynamicExtensionParameters != other.dynamicExtensionParameters) return false
if (environmentId != other.environmentId) return false
if (kmsKeyIdentifier != other.kmsKeyIdentifier) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.StartDeploymentRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The application ID.
*/
public var applicationId: kotlin.String? = null
/**
* The configuration profile ID.
*/
public var configurationProfileId: kotlin.String? = null
/**
* The configuration version to deploy. If deploying an AppConfig hosted configuration version, you can specify either the version number or version label. For all other configurations, you must specify the version number.
*/
public var configurationVersion: kotlin.String? = null
/**
* The deployment strategy ID.
*/
public var deploymentStrategyId: kotlin.String? = null
/**
* A description of the deployment.
*/
public var description: kotlin.String? = null
/**
* A map of dynamic extension parameter names to values to pass to associated extensions with `PRE_START_DEPLOYMENT` actions.
*/
public var dynamicExtensionParameters: Map? = null
/**
* The environment ID.
*/
public var environmentId: kotlin.String? = null
/**
* The KMS key identifier (key ID, key alias, or key ARN). AppConfig uses this ID to encrypt the configuration data using a customer managed key.
*/
public var kmsKeyIdentifier: kotlin.String? = null
/**
* Metadata to assign to the deployment. Tags help organize and categorize your AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.StartDeploymentRequest) : this() {
this.applicationId = x.applicationId
this.configurationProfileId = x.configurationProfileId
this.configurationVersion = x.configurationVersion
this.deploymentStrategyId = x.deploymentStrategyId
this.description = x.description
this.dynamicExtensionParameters = x.dynamicExtensionParameters
this.environmentId = x.environmentId
this.kmsKeyIdentifier = x.kmsKeyIdentifier
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.StartDeploymentRequest = StartDeploymentRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy