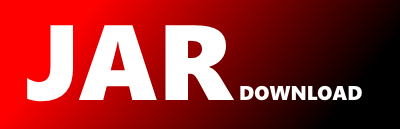
commonMain.aws.sdk.kotlin.services.appconfig.model.StopDeploymentResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconfig-jvm Show documentation
Show all versions of appconfig-jvm Show documentation
The AWS SDK for Kotlin client for AppConfig
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appconfig.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class StopDeploymentResponse private constructor(builder: Builder) {
/**
* The ID of the application that was deployed.
*/
public val applicationId: kotlin.String? = builder.applicationId
/**
* A list of extensions that were processed as part of the deployment. The extensions that were previously associated to the configuration profile, environment, or the application when `StartDeployment` was called.
*/
public val appliedExtensions: List? = builder.appliedExtensions
/**
* The time the deployment completed.
*/
public val completedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.completedAt
/**
* Information about the source location of the configuration.
*/
public val configurationLocationUri: kotlin.String? = builder.configurationLocationUri
/**
* The name of the configuration.
*/
public val configurationName: kotlin.String? = builder.configurationName
/**
* The ID of the configuration profile that was deployed.
*/
public val configurationProfileId: kotlin.String? = builder.configurationProfileId
/**
* The configuration version that was deployed.
*/
public val configurationVersion: kotlin.String? = builder.configurationVersion
/**
* Total amount of time the deployment lasted.
*/
public val deploymentDurationInMinutes: kotlin.Int = builder.deploymentDurationInMinutes
/**
* The sequence number of the deployment.
*/
public val deploymentNumber: kotlin.Int = builder.deploymentNumber
/**
* The ID of the deployment strategy that was deployed.
*/
public val deploymentStrategyId: kotlin.String? = builder.deploymentStrategyId
/**
* The description of the deployment.
*/
public val description: kotlin.String? = builder.description
/**
* The ID of the environment that was deployed.
*/
public val environmentId: kotlin.String? = builder.environmentId
/**
* A list containing all events related to a deployment. The most recent events are displayed first.
*/
public val eventLog: List? = builder.eventLog
/**
* The amount of time that AppConfig monitored for alarms before considering the deployment to be complete and no longer eligible for automatic rollback.
*/
public val finalBakeTimeInMinutes: kotlin.Int = builder.finalBakeTimeInMinutes
/**
* The percentage of targets to receive a deployed configuration during each interval.
*/
public val growthFactor: kotlin.Float? = builder.growthFactor
/**
* The algorithm used to define how percentage grew over time.
*/
public val growthType: aws.sdk.kotlin.services.appconfig.model.GrowthType? = builder.growthType
/**
* The Amazon Resource Name of the Key Management Service key used to encrypt configuration data. You can encrypt secrets stored in Secrets Manager, Amazon Simple Storage Service (Amazon S3) objects encrypted with SSE-KMS, or secure string parameters stored in Amazon Web Services Systems Manager Parameter Store.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created or updated.
*/
public val kmsKeyIdentifier: kotlin.String? = builder.kmsKeyIdentifier
/**
* The percentage of targets for which the deployment is available.
*/
public val percentageComplete: kotlin.Float? = builder.percentageComplete
/**
* The time the deployment started.
*/
public val startedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.startedAt
/**
* The state of the deployment.
*/
public val state: aws.sdk.kotlin.services.appconfig.model.DeploymentState? = builder.state
/**
* A user-defined label for an AppConfig hosted configuration version.
*/
public val versionLabel: kotlin.String? = builder.versionLabel
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appconfig.model.StopDeploymentResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StopDeploymentResponse(")
append("applicationId=$applicationId,")
append("appliedExtensions=$appliedExtensions,")
append("completedAt=$completedAt,")
append("configurationLocationUri=$configurationLocationUri,")
append("configurationName=$configurationName,")
append("configurationProfileId=$configurationProfileId,")
append("configurationVersion=$configurationVersion,")
append("deploymentDurationInMinutes=$deploymentDurationInMinutes,")
append("deploymentNumber=$deploymentNumber,")
append("deploymentStrategyId=$deploymentStrategyId,")
append("description=$description,")
append("environmentId=$environmentId,")
append("eventLog=$eventLog,")
append("finalBakeTimeInMinutes=$finalBakeTimeInMinutes,")
append("growthFactor=$growthFactor,")
append("growthType=$growthType,")
append("kmsKeyArn=$kmsKeyArn,")
append("kmsKeyIdentifier=$kmsKeyIdentifier,")
append("percentageComplete=$percentageComplete,")
append("startedAt=$startedAt,")
append("state=$state,")
append("versionLabel=$versionLabel")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationId?.hashCode() ?: 0
result = 31 * result + (appliedExtensions?.hashCode() ?: 0)
result = 31 * result + (completedAt?.hashCode() ?: 0)
result = 31 * result + (configurationLocationUri?.hashCode() ?: 0)
result = 31 * result + (configurationName?.hashCode() ?: 0)
result = 31 * result + (configurationProfileId?.hashCode() ?: 0)
result = 31 * result + (configurationVersion?.hashCode() ?: 0)
result = 31 * result + (deploymentDurationInMinutes)
result = 31 * result + (deploymentNumber)
result = 31 * result + (deploymentStrategyId?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (environmentId?.hashCode() ?: 0)
result = 31 * result + (eventLog?.hashCode() ?: 0)
result = 31 * result + (finalBakeTimeInMinutes)
result = 31 * result + (growthFactor?.hashCode() ?: 0)
result = 31 * result + (growthType?.hashCode() ?: 0)
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (kmsKeyIdentifier?.hashCode() ?: 0)
result = 31 * result + (percentageComplete?.hashCode() ?: 0)
result = 31 * result + (startedAt?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (versionLabel?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StopDeploymentResponse
if (applicationId != other.applicationId) return false
if (appliedExtensions != other.appliedExtensions) return false
if (completedAt != other.completedAt) return false
if (configurationLocationUri != other.configurationLocationUri) return false
if (configurationName != other.configurationName) return false
if (configurationProfileId != other.configurationProfileId) return false
if (configurationVersion != other.configurationVersion) return false
if (deploymentDurationInMinutes != other.deploymentDurationInMinutes) return false
if (deploymentNumber != other.deploymentNumber) return false
if (deploymentStrategyId != other.deploymentStrategyId) return false
if (description != other.description) return false
if (environmentId != other.environmentId) return false
if (eventLog != other.eventLog) return false
if (finalBakeTimeInMinutes != other.finalBakeTimeInMinutes) return false
if (!(growthFactor?.equals(other.growthFactor) ?: (other.growthFactor == null))) return false
if (growthType != other.growthType) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (kmsKeyIdentifier != other.kmsKeyIdentifier) return false
if (!(percentageComplete?.equals(other.percentageComplete) ?: (other.percentageComplete == null))) return false
if (startedAt != other.startedAt) return false
if (state != other.state) return false
if (versionLabel != other.versionLabel) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appconfig.model.StopDeploymentResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the application that was deployed.
*/
public var applicationId: kotlin.String? = null
/**
* A list of extensions that were processed as part of the deployment. The extensions that were previously associated to the configuration profile, environment, or the application when `StartDeployment` was called.
*/
public var appliedExtensions: List? = null
/**
* The time the deployment completed.
*/
public var completedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Information about the source location of the configuration.
*/
public var configurationLocationUri: kotlin.String? = null
/**
* The name of the configuration.
*/
public var configurationName: kotlin.String? = null
/**
* The ID of the configuration profile that was deployed.
*/
public var configurationProfileId: kotlin.String? = null
/**
* The configuration version that was deployed.
*/
public var configurationVersion: kotlin.String? = null
/**
* Total amount of time the deployment lasted.
*/
public var deploymentDurationInMinutes: kotlin.Int = 0
/**
* The sequence number of the deployment.
*/
public var deploymentNumber: kotlin.Int = 0
/**
* The ID of the deployment strategy that was deployed.
*/
public var deploymentStrategyId: kotlin.String? = null
/**
* The description of the deployment.
*/
public var description: kotlin.String? = null
/**
* The ID of the environment that was deployed.
*/
public var environmentId: kotlin.String? = null
/**
* A list containing all events related to a deployment. The most recent events are displayed first.
*/
public var eventLog: List? = null
/**
* The amount of time that AppConfig monitored for alarms before considering the deployment to be complete and no longer eligible for automatic rollback.
*/
public var finalBakeTimeInMinutes: kotlin.Int = 0
/**
* The percentage of targets to receive a deployed configuration during each interval.
*/
public var growthFactor: kotlin.Float? = null
/**
* The algorithm used to define how percentage grew over time.
*/
public var growthType: aws.sdk.kotlin.services.appconfig.model.GrowthType? = null
/**
* The Amazon Resource Name of the Key Management Service key used to encrypt configuration data. You can encrypt secrets stored in Secrets Manager, Amazon Simple Storage Service (Amazon S3) objects encrypted with SSE-KMS, or secure string parameters stored in Amazon Web Services Systems Manager Parameter Store.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* The Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created or updated.
*/
public var kmsKeyIdentifier: kotlin.String? = null
/**
* The percentage of targets for which the deployment is available.
*/
public var percentageComplete: kotlin.Float? = null
/**
* The time the deployment started.
*/
public var startedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The state of the deployment.
*/
public var state: aws.sdk.kotlin.services.appconfig.model.DeploymentState? = null
/**
* A user-defined label for an AppConfig hosted configuration version.
*/
public var versionLabel: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appconfig.model.StopDeploymentResponse) : this() {
this.applicationId = x.applicationId
this.appliedExtensions = x.appliedExtensions
this.completedAt = x.completedAt
this.configurationLocationUri = x.configurationLocationUri
this.configurationName = x.configurationName
this.configurationProfileId = x.configurationProfileId
this.configurationVersion = x.configurationVersion
this.deploymentDurationInMinutes = x.deploymentDurationInMinutes
this.deploymentNumber = x.deploymentNumber
this.deploymentStrategyId = x.deploymentStrategyId
this.description = x.description
this.environmentId = x.environmentId
this.eventLog = x.eventLog
this.finalBakeTimeInMinutes = x.finalBakeTimeInMinutes
this.growthFactor = x.growthFactor
this.growthType = x.growthType
this.kmsKeyArn = x.kmsKeyArn
this.kmsKeyIdentifier = x.kmsKeyIdentifier
this.percentageComplete = x.percentageComplete
this.startedAt = x.startedAt
this.state = x.state
this.versionLabel = x.versionLabel
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appconfig.model.StopDeploymentResponse = StopDeploymentResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy