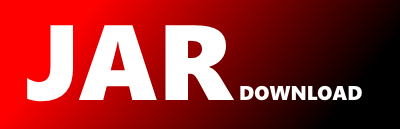
commonMain.aws.sdk.kotlin.services.appfabric.model.AppAuthorizationSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appfabric-jvm Show documentation
Show all versions of appfabric-jvm Show documentation
The AWS SDK for Kotlin client for AppFabric
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appfabric.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Contains a summary of an app authorization.
*/
public class AppAuthorizationSummary private constructor(builder: Builder) {
/**
* The name of the application.
*/
public val app: kotlin.String = requireNotNull(builder.app) { "A non-null value must be provided for app" }
/**
* The Amazon Resource Name (ARN) of the app authorization.
*/
public val appAuthorizationArn: kotlin.String = requireNotNull(builder.appAuthorizationArn) { "A non-null value must be provided for appAuthorizationArn" }
/**
* The Amazon Resource Name (ARN) of the app bundle for the app authorization.
*/
public val appBundleArn: kotlin.String = requireNotNull(builder.appBundleArn) { "A non-null value must be provided for appBundleArn" }
/**
* The state of the app authorization.
*
* The following states are possible:
* + `PendingConnect`: The initial state of the app authorization. The app authorization is created but not yet connected.
* + `Connected`: The app authorization is connected to the application, and is ready to be used.
* + `ConnectionValidationFailed`: The app authorization received a validation exception when trying to connect to the application. If the app authorization is in this state, you should verify the configured credentials and try to connect the app authorization again.
* + `TokenAutoRotationFailed`: AppFabric failed to refresh the access token. If the app authorization is in this state, you should try to reconnect the app authorization.
*/
public val status: aws.sdk.kotlin.services.appfabric.model.AppAuthorizationStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
/**
* Contains information about an application tenant, such as the application display name and identifier.
*/
public val tenant: aws.sdk.kotlin.services.appfabric.model.Tenant? = builder.tenant
/**
* Timestamp for when the app authorization was last updated.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.updatedAt) { "A non-null value must be provided for updatedAt" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appfabric.model.AppAuthorizationSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AppAuthorizationSummary(")
append("app=$app,")
append("appAuthorizationArn=$appAuthorizationArn,")
append("appBundleArn=$appBundleArn,")
append("status=$status,")
append("tenant=$tenant,")
append("updatedAt=$updatedAt")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = app.hashCode()
result = 31 * result + (appAuthorizationArn.hashCode())
result = 31 * result + (appBundleArn.hashCode())
result = 31 * result + (status.hashCode())
result = 31 * result + (tenant?.hashCode() ?: 0)
result = 31 * result + (updatedAt.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AppAuthorizationSummary
if (app != other.app) return false
if (appAuthorizationArn != other.appAuthorizationArn) return false
if (appBundleArn != other.appBundleArn) return false
if (status != other.status) return false
if (tenant != other.tenant) return false
if (updatedAt != other.updatedAt) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appfabric.model.AppAuthorizationSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The name of the application.
*/
public var app: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the app authorization.
*/
public var appAuthorizationArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the app bundle for the app authorization.
*/
public var appBundleArn: kotlin.String? = null
/**
* The state of the app authorization.
*
* The following states are possible:
* + `PendingConnect`: The initial state of the app authorization. The app authorization is created but not yet connected.
* + `Connected`: The app authorization is connected to the application, and is ready to be used.
* + `ConnectionValidationFailed`: The app authorization received a validation exception when trying to connect to the application. If the app authorization is in this state, you should verify the configured credentials and try to connect the app authorization again.
* + `TokenAutoRotationFailed`: AppFabric failed to refresh the access token. If the app authorization is in this state, you should try to reconnect the app authorization.
*/
public var status: aws.sdk.kotlin.services.appfabric.model.AppAuthorizationStatus? = null
/**
* Contains information about an application tenant, such as the application display name and identifier.
*/
public var tenant: aws.sdk.kotlin.services.appfabric.model.Tenant? = null
/**
* Timestamp for when the app authorization was last updated.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appfabric.model.AppAuthorizationSummary) : this() {
this.app = x.app
this.appAuthorizationArn = x.appAuthorizationArn
this.appBundleArn = x.appBundleArn
this.status = x.status
this.tenant = x.tenant
this.updatedAt = x.updatedAt
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appfabric.model.AppAuthorizationSummary = AppAuthorizationSummary(this)
/**
* construct an [aws.sdk.kotlin.services.appfabric.model.Tenant] inside the given [block]
*/
public fun tenant(block: aws.sdk.kotlin.services.appfabric.model.Tenant.Builder.() -> kotlin.Unit) {
this.tenant = aws.sdk.kotlin.services.appfabric.model.Tenant.invoke(block)
}
internal fun correctErrors(): Builder {
if (app == null) app = ""
if (appAuthorizationArn == null) appAuthorizationArn = ""
if (appBundleArn == null) appBundleArn = ""
if (status == null) status = AppAuthorizationStatus.SdkUnknown("no value provided")
if (updatedAt == null) updatedAt = Instant.fromEpochSeconds(0)
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy