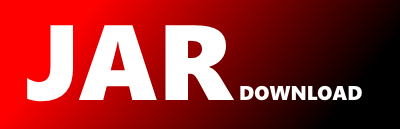
commonMain.aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationdiscoveryservice-jvm Show documentation
Show all versions of applicationdiscoveryservice-jvm Show documentation
The AWS SDK for Kotlin client for Application Discovery Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationdiscoveryservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Inventory data for installed discovery agents.
*/
public class CustomerAgentInfo private constructor(builder: Builder) {
/**
* Number of active discovery agents.
*/
public val activeAgents: kotlin.Int = builder.activeAgents
/**
* Number of blacklisted discovery agents.
*/
public val blackListedAgents: kotlin.Int = builder.blackListedAgents
/**
* Number of healthy discovery agents
*/
public val healthyAgents: kotlin.Int = builder.healthyAgents
/**
* Number of discovery agents with status SHUTDOWN.
*/
public val shutdownAgents: kotlin.Int = builder.shutdownAgents
/**
* Total number of discovery agents.
*/
public val totalAgents: kotlin.Int = builder.totalAgents
/**
* Number of unhealthy discovery agents.
*/
public val unhealthyAgents: kotlin.Int = builder.unhealthyAgents
/**
* Number of unknown discovery agents.
*/
public val unknownAgents: kotlin.Int = builder.unknownAgents
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CustomerAgentInfo(")
append("activeAgents=$activeAgents,")
append("blackListedAgents=$blackListedAgents,")
append("healthyAgents=$healthyAgents,")
append("shutdownAgents=$shutdownAgents,")
append("totalAgents=$totalAgents,")
append("unhealthyAgents=$unhealthyAgents,")
append("unknownAgents=$unknownAgents")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = activeAgents
result = 31 * result + (blackListedAgents)
result = 31 * result + (healthyAgents)
result = 31 * result + (shutdownAgents)
result = 31 * result + (totalAgents)
result = 31 * result + (unhealthyAgents)
result = 31 * result + (unknownAgents)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CustomerAgentInfo
if (activeAgents != other.activeAgents) return false
if (blackListedAgents != other.blackListedAgents) return false
if (healthyAgents != other.healthyAgents) return false
if (shutdownAgents != other.shutdownAgents) return false
if (totalAgents != other.totalAgents) return false
if (unhealthyAgents != other.unhealthyAgents) return false
if (unknownAgents != other.unknownAgents) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Number of active discovery agents.
*/
public var activeAgents: kotlin.Int = 0
/**
* Number of blacklisted discovery agents.
*/
public var blackListedAgents: kotlin.Int = 0
/**
* Number of healthy discovery agents
*/
public var healthyAgents: kotlin.Int = 0
/**
* Number of discovery agents with status SHUTDOWN.
*/
public var shutdownAgents: kotlin.Int = 0
/**
* Total number of discovery agents.
*/
public var totalAgents: kotlin.Int = 0
/**
* Number of unhealthy discovery agents.
*/
public var unhealthyAgents: kotlin.Int = 0
/**
* Number of unknown discovery agents.
*/
public var unknownAgents: kotlin.Int = 0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo) : this() {
this.activeAgents = x.activeAgents
this.blackListedAgents = x.blackListedAgents
this.healthyAgents = x.healthyAgents
this.shutdownAgents = x.shutdownAgents
this.totalAgents = x.totalAgents
this.unhealthyAgents = x.unhealthyAgents
this.unknownAgents = x.unknownAgents
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo = CustomerAgentInfo(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy