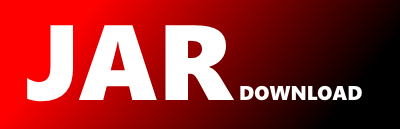
commonMain.aws.sdk.kotlin.services.applicationdiscoveryservice.model.GetDiscoverySummaryResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationdiscoveryservice-jvm Show documentation
Show all versions of applicationdiscoveryservice-jvm Show documentation
The AWS SDK for Kotlin client for Application Discovery Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationdiscoveryservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetDiscoverySummaryResponse private constructor(builder: Builder) {
/**
* Details about discovered agents, including agent status and health.
*/
public val agentSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo? = builder.agentSummary
/**
* Details about Agentless Collector collectors, including status.
*/
public val agentlessCollectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentlessCollectorInfo? = builder.agentlessCollectorSummary
/**
* The number of applications discovered.
*/
public val applications: kotlin.Long = builder.applications
/**
* Details about discovered connectors, including connector status and health.
*/
public val connectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerConnectorInfo? = builder.connectorSummary
/**
* Details about Migration Evaluator collectors, including collector status and health.
*/
public val meCollectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerMeCollectorInfo? = builder.meCollectorSummary
/**
* The number of servers discovered.
*/
public val servers: kotlin.Long = builder.servers
/**
* The number of servers mapped to applications.
*/
public val serversMappedToApplications: kotlin.Long = builder.serversMappedToApplications
/**
* The number of servers mapped to tags.
*/
public val serversMappedtoTags: kotlin.Long = builder.serversMappedtoTags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationdiscoveryservice.model.GetDiscoverySummaryResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetDiscoverySummaryResponse(")
append("agentSummary=$agentSummary,")
append("agentlessCollectorSummary=$agentlessCollectorSummary,")
append("applications=$applications,")
append("connectorSummary=$connectorSummary,")
append("meCollectorSummary=$meCollectorSummary,")
append("servers=$servers,")
append("serversMappedToApplications=$serversMappedToApplications,")
append("serversMappedtoTags=$serversMappedtoTags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentSummary?.hashCode() ?: 0
result = 31 * result + (agentlessCollectorSummary?.hashCode() ?: 0)
result = 31 * result + (applications.hashCode())
result = 31 * result + (connectorSummary?.hashCode() ?: 0)
result = 31 * result + (meCollectorSummary?.hashCode() ?: 0)
result = 31 * result + (servers.hashCode())
result = 31 * result + (serversMappedToApplications.hashCode())
result = 31 * result + (serversMappedtoTags.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetDiscoverySummaryResponse
if (agentSummary != other.agentSummary) return false
if (agentlessCollectorSummary != other.agentlessCollectorSummary) return false
if (applications != other.applications) return false
if (connectorSummary != other.connectorSummary) return false
if (meCollectorSummary != other.meCollectorSummary) return false
if (servers != other.servers) return false
if (serversMappedToApplications != other.serversMappedToApplications) return false
if (serversMappedtoTags != other.serversMappedtoTags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationdiscoveryservice.model.GetDiscoverySummaryResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Details about discovered agents, including agent status and health.
*/
public var agentSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo? = null
/**
* Details about Agentless Collector collectors, including status.
*/
public var agentlessCollectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentlessCollectorInfo? = null
/**
* The number of applications discovered.
*/
public var applications: kotlin.Long = 0L
/**
* Details about discovered connectors, including connector status and health.
*/
public var connectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerConnectorInfo? = null
/**
* Details about Migration Evaluator collectors, including collector status and health.
*/
public var meCollectorSummary: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerMeCollectorInfo? = null
/**
* The number of servers discovered.
*/
public var servers: kotlin.Long = 0L
/**
* The number of servers mapped to applications.
*/
public var serversMappedToApplications: kotlin.Long = 0L
/**
* The number of servers mapped to tags.
*/
public var serversMappedtoTags: kotlin.Long = 0L
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationdiscoveryservice.model.GetDiscoverySummaryResponse) : this() {
this.agentSummary = x.agentSummary
this.agentlessCollectorSummary = x.agentlessCollectorSummary
this.applications = x.applications
this.connectorSummary = x.connectorSummary
this.meCollectorSummary = x.meCollectorSummary
this.servers = x.servers
this.serversMappedToApplications = x.serversMappedToApplications
this.serversMappedtoTags = x.serversMappedtoTags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationdiscoveryservice.model.GetDiscoverySummaryResponse = GetDiscoverySummaryResponse(this)
/**
* construct an [aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo] inside the given [block]
*/
public fun agentSummary(block: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo.Builder.() -> kotlin.Unit) {
this.agentSummary = aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentInfo.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentlessCollectorInfo] inside the given [block]
*/
public fun agentlessCollectorSummary(block: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentlessCollectorInfo.Builder.() -> kotlin.Unit) {
this.agentlessCollectorSummary = aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerAgentlessCollectorInfo.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerConnectorInfo] inside the given [block]
*/
public fun connectorSummary(block: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerConnectorInfo.Builder.() -> kotlin.Unit) {
this.connectorSummary = aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerConnectorInfo.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerMeCollectorInfo] inside the given [block]
*/
public fun meCollectorSummary(block: aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerMeCollectorInfo.Builder.() -> kotlin.Unit) {
this.meCollectorSummary = aws.sdk.kotlin.services.applicationdiscoveryservice.model.CustomerMeCollectorInfo.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy