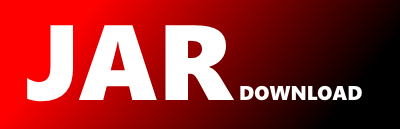
commonMain.aws.sdk.kotlin.services.applicationinsights.model.Problem.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Describes a problem that is detected by correlating observations.
*/
public class Problem private constructor(builder: Builder) {
/**
* The AWS account ID for the owner of the resource group affected by the problem.
*/
public val accountId: kotlin.String? = builder.accountId
/**
* The resource affected by the problem.
*/
public val affectedResource: kotlin.String? = builder.affectedResource
/**
* The time when the problem ended, in epoch seconds.
*/
public val endTime: aws.smithy.kotlin.runtime.time.Instant? = builder.endTime
/**
* Feedback provided by the user about the problem.
*/
public val feedback: Map? = builder.feedback
/**
* The ID of the problem.
*/
public val id: kotlin.String? = builder.id
/**
* A detailed analysis of the problem using machine learning.
*/
public val insights: kotlin.String? = builder.insights
/**
* The last time that the problem reoccurred after its last resolution.
*/
public val lastRecurrenceTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastRecurrenceTime
/**
* The number of times that the same problem reoccurred after the first time it was resolved.
*/
public val recurringCount: kotlin.Long? = builder.recurringCount
/**
* Specifies how the problem was resolved. If the value is `AUTOMATIC`, the system resolved the problem. If the value is `MANUAL`, the user resolved the problem. If the value is `UNRESOLVED`, then the problem is not resolved.
*/
public val resolutionMethod: aws.sdk.kotlin.services.applicationinsights.model.ResolutionMethod? = builder.resolutionMethod
/**
* The name of the resource group affected by the problem.
*/
public val resourceGroupName: kotlin.String? = builder.resourceGroupName
/**
* A measure of the level of impact of the problem.
*/
public val severityLevel: aws.sdk.kotlin.services.applicationinsights.model.SeverityLevel? = builder.severityLevel
/**
* The time when the problem started, in epoch seconds.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The status of the problem.
*/
public val status: aws.sdk.kotlin.services.applicationinsights.model.Status? = builder.status
/**
* The name of the problem.
*/
public val title: kotlin.String? = builder.title
/**
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*/
public val visibility: aws.sdk.kotlin.services.applicationinsights.model.Visibility? = builder.visibility
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationinsights.model.Problem = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Problem(")
append("accountId=$accountId,")
append("affectedResource=$affectedResource,")
append("endTime=$endTime,")
append("feedback=$feedback,")
append("id=$id,")
append("insights=$insights,")
append("lastRecurrenceTime=$lastRecurrenceTime,")
append("recurringCount=$recurringCount,")
append("resolutionMethod=$resolutionMethod,")
append("resourceGroupName=$resourceGroupName,")
append("severityLevel=$severityLevel,")
append("startTime=$startTime,")
append("status=$status,")
append("title=$title,")
append("visibility=$visibility")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accountId?.hashCode() ?: 0
result = 31 * result + (affectedResource?.hashCode() ?: 0)
result = 31 * result + (endTime?.hashCode() ?: 0)
result = 31 * result + (feedback?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (insights?.hashCode() ?: 0)
result = 31 * result + (lastRecurrenceTime?.hashCode() ?: 0)
result = 31 * result + (recurringCount?.hashCode() ?: 0)
result = 31 * result + (resolutionMethod?.hashCode() ?: 0)
result = 31 * result + (resourceGroupName?.hashCode() ?: 0)
result = 31 * result + (severityLevel?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (title?.hashCode() ?: 0)
result = 31 * result + (visibility?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Problem
if (accountId != other.accountId) return false
if (affectedResource != other.affectedResource) return false
if (endTime != other.endTime) return false
if (feedback != other.feedback) return false
if (id != other.id) return false
if (insights != other.insights) return false
if (lastRecurrenceTime != other.lastRecurrenceTime) return false
if (recurringCount != other.recurringCount) return false
if (resolutionMethod != other.resolutionMethod) return false
if (resourceGroupName != other.resourceGroupName) return false
if (severityLevel != other.severityLevel) return false
if (startTime != other.startTime) return false
if (status != other.status) return false
if (title != other.title) return false
if (visibility != other.visibility) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationinsights.model.Problem = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The AWS account ID for the owner of the resource group affected by the problem.
*/
public var accountId: kotlin.String? = null
/**
* The resource affected by the problem.
*/
public var affectedResource: kotlin.String? = null
/**
* The time when the problem ended, in epoch seconds.
*/
public var endTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Feedback provided by the user about the problem.
*/
public var feedback: Map? = null
/**
* The ID of the problem.
*/
public var id: kotlin.String? = null
/**
* A detailed analysis of the problem using machine learning.
*/
public var insights: kotlin.String? = null
/**
* The last time that the problem reoccurred after its last resolution.
*/
public var lastRecurrenceTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The number of times that the same problem reoccurred after the first time it was resolved.
*/
public var recurringCount: kotlin.Long? = null
/**
* Specifies how the problem was resolved. If the value is `AUTOMATIC`, the system resolved the problem. If the value is `MANUAL`, the user resolved the problem. If the value is `UNRESOLVED`, then the problem is not resolved.
*/
public var resolutionMethod: aws.sdk.kotlin.services.applicationinsights.model.ResolutionMethod? = null
/**
* The name of the resource group affected by the problem.
*/
public var resourceGroupName: kotlin.String? = null
/**
* A measure of the level of impact of the problem.
*/
public var severityLevel: aws.sdk.kotlin.services.applicationinsights.model.SeverityLevel? = null
/**
* The time when the problem started, in epoch seconds.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the problem.
*/
public var status: aws.sdk.kotlin.services.applicationinsights.model.Status? = null
/**
* The name of the problem.
*/
public var title: kotlin.String? = null
/**
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*/
public var visibility: aws.sdk.kotlin.services.applicationinsights.model.Visibility? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationinsights.model.Problem) : this() {
this.accountId = x.accountId
this.affectedResource = x.affectedResource
this.endTime = x.endTime
this.feedback = x.feedback
this.id = x.id
this.insights = x.insights
this.lastRecurrenceTime = x.lastRecurrenceTime
this.recurringCount = x.recurringCount
this.resolutionMethod = x.resolutionMethod
this.resourceGroupName = x.resourceGroupName
this.severityLevel = x.severityLevel
this.startTime = x.startTime
this.status = x.status
this.title = x.title
this.visibility = x.visibility
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationinsights.model.Problem = Problem(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy