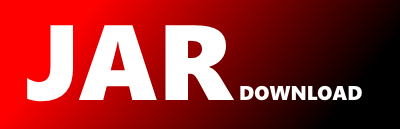
commonMain.aws.sdk.kotlin.services.applicationinsights.model.UpdateLogPatternRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateLogPatternRequest private constructor(builder: Builder) {
/**
* The log pattern. The pattern must be DFA compatible. Patterns that utilize forward lookahead or backreference constructions are not supported.
*/
public val pattern: kotlin.String? = builder.pattern
/**
* The name of the log pattern.
*/
public val patternName: kotlin.String? = builder.patternName
/**
* The name of the log pattern set.
*/
public val patternSetName: kotlin.String? = builder.patternSetName
/**
* Rank of the log pattern. Must be a value between `1` and `1,000,000`. The patterns are sorted by rank, so we recommend that you set your highest priority patterns with the lowest rank. A pattern of rank `1` will be the first to get matched to a log line. A pattern of rank `1,000,000` will be last to get matched. When you configure custom log patterns from the console, a `Low` severity pattern translates to a `750,000` rank. A `Medium` severity pattern translates to a `500,000` rank. And a `High` severity pattern translates to a `250,000` rank. Rank values less than `1` or greater than `1,000,000` are reserved for AWS-provided patterns.
*/
public val rank: kotlin.Int? = builder.rank
/**
* The name of the resource group.
*/
public val resourceGroupName: kotlin.String? = builder.resourceGroupName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationinsights.model.UpdateLogPatternRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateLogPatternRequest(")
append("pattern=$pattern,")
append("patternName=$patternName,")
append("patternSetName=$patternSetName,")
append("rank=$rank,")
append("resourceGroupName=$resourceGroupName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = pattern?.hashCode() ?: 0
result = 31 * result + (patternName?.hashCode() ?: 0)
result = 31 * result + (patternSetName?.hashCode() ?: 0)
result = 31 * result + (rank ?: 0)
result = 31 * result + (resourceGroupName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateLogPatternRequest
if (pattern != other.pattern) return false
if (patternName != other.patternName) return false
if (patternSetName != other.patternSetName) return false
if (rank != other.rank) return false
if (resourceGroupName != other.resourceGroupName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationinsights.model.UpdateLogPatternRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The log pattern. The pattern must be DFA compatible. Patterns that utilize forward lookahead or backreference constructions are not supported.
*/
public var pattern: kotlin.String? = null
/**
* The name of the log pattern.
*/
public var patternName: kotlin.String? = null
/**
* The name of the log pattern set.
*/
public var patternSetName: kotlin.String? = null
/**
* Rank of the log pattern. Must be a value between `1` and `1,000,000`. The patterns are sorted by rank, so we recommend that you set your highest priority patterns with the lowest rank. A pattern of rank `1` will be the first to get matched to a log line. A pattern of rank `1,000,000` will be last to get matched. When you configure custom log patterns from the console, a `Low` severity pattern translates to a `750,000` rank. A `Medium` severity pattern translates to a `500,000` rank. And a `High` severity pattern translates to a `250,000` rank. Rank values less than `1` or greater than `1,000,000` are reserved for AWS-provided patterns.
*/
public var rank: kotlin.Int? = null
/**
* The name of the resource group.
*/
public var resourceGroupName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationinsights.model.UpdateLogPatternRequest) : this() {
this.pattern = x.pattern
this.patternName = x.patternName
this.patternSetName = x.patternSetName
this.rank = x.rank
this.resourceGroupName = x.resourceGroupName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationinsights.model.UpdateLogPatternRequest = UpdateLogPatternRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy