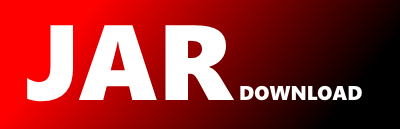
commonMain.aws.sdk.kotlin.services.applicationinsights.model.CreateApplicationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateApplicationRequest private constructor(builder: Builder) {
/**
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*/
public val attachMissingPermission: kotlin.Boolean? = builder.attachMissingPermission
/**
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*/
public val autoConfigEnabled: kotlin.Boolean? = builder.autoConfigEnabled
/**
* Configures all of the resources in the resource group by applying the recommended configurations.
*/
public val autoCreate: kotlin.Boolean? = builder.autoCreate
/**
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as `instance terminated`, `failed deployment`, and others.
*/
public val cweMonitorEnabled: kotlin.Boolean? = builder.cweMonitorEnabled
/**
* Application Insights can create applications based on a resource group or on an account. To create an account-based application using all of the resources in the account, set this parameter to `ACCOUNT_BASED`.
*/
public val groupingType: aws.sdk.kotlin.services.applicationinsights.model.GroupingType? = builder.groupingType
/**
* When set to `true`, creates opsItems for any problems detected on an application.
*/
public val opsCenterEnabled: kotlin.Boolean? = builder.opsCenterEnabled
/**
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive notifications for updates to the opsItem.
*/
public val opsItemSnsTopicArn: kotlin.String? = builder.opsItemSnsTopicArn
/**
* The name of the resource group.
*/
public val resourceGroupName: kotlin.String? = builder.resourceGroupName
/**
* List of tags to add to the application. tag key (`Key`) and an associated tag value (`Value`). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256 characters.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationinsights.model.CreateApplicationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateApplicationRequest(")
append("attachMissingPermission=$attachMissingPermission,")
append("autoConfigEnabled=$autoConfigEnabled,")
append("autoCreate=$autoCreate,")
append("cweMonitorEnabled=$cweMonitorEnabled,")
append("groupingType=$groupingType,")
append("opsCenterEnabled=$opsCenterEnabled,")
append("opsItemSnsTopicArn=$opsItemSnsTopicArn,")
append("resourceGroupName=$resourceGroupName,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = attachMissingPermission?.hashCode() ?: 0
result = 31 * result + (autoConfigEnabled?.hashCode() ?: 0)
result = 31 * result + (autoCreate?.hashCode() ?: 0)
result = 31 * result + (cweMonitorEnabled?.hashCode() ?: 0)
result = 31 * result + (groupingType?.hashCode() ?: 0)
result = 31 * result + (opsCenterEnabled?.hashCode() ?: 0)
result = 31 * result + (opsItemSnsTopicArn?.hashCode() ?: 0)
result = 31 * result + (resourceGroupName?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateApplicationRequest
if (attachMissingPermission != other.attachMissingPermission) return false
if (autoConfigEnabled != other.autoConfigEnabled) return false
if (autoCreate != other.autoCreate) return false
if (cweMonitorEnabled != other.cweMonitorEnabled) return false
if (groupingType != other.groupingType) return false
if (opsCenterEnabled != other.opsCenterEnabled) return false
if (opsItemSnsTopicArn != other.opsItemSnsTopicArn) return false
if (resourceGroupName != other.resourceGroupName) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationinsights.model.CreateApplicationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*/
public var attachMissingPermission: kotlin.Boolean? = null
/**
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*/
public var autoConfigEnabled: kotlin.Boolean? = null
/**
* Configures all of the resources in the resource group by applying the recommended configurations.
*/
public var autoCreate: kotlin.Boolean? = null
/**
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as `instance terminated`, `failed deployment`, and others.
*/
public var cweMonitorEnabled: kotlin.Boolean? = null
/**
* Application Insights can create applications based on a resource group or on an account. To create an account-based application using all of the resources in the account, set this parameter to `ACCOUNT_BASED`.
*/
public var groupingType: aws.sdk.kotlin.services.applicationinsights.model.GroupingType? = null
/**
* When set to `true`, creates opsItems for any problems detected on an application.
*/
public var opsCenterEnabled: kotlin.Boolean? = null
/**
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive notifications for updates to the opsItem.
*/
public var opsItemSnsTopicArn: kotlin.String? = null
/**
* The name of the resource group.
*/
public var resourceGroupName: kotlin.String? = null
/**
* List of tags to add to the application. tag key (`Key`) and an associated tag value (`Value`). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256 characters.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationinsights.model.CreateApplicationRequest) : this() {
this.attachMissingPermission = x.attachMissingPermission
this.autoConfigEnabled = x.autoConfigEnabled
this.autoCreate = x.autoCreate
this.cweMonitorEnabled = x.cweMonitorEnabled
this.groupingType = x.groupingType
this.opsCenterEnabled = x.opsCenterEnabled
this.opsItemSnsTopicArn = x.opsItemSnsTopicArn
this.resourceGroupName = x.resourceGroupName
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationinsights.model.CreateApplicationRequest = CreateApplicationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy