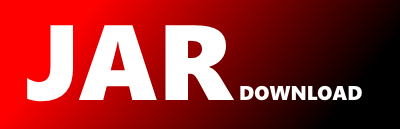
commonMain.aws.sdk.kotlin.services.applicationinsights.model.Observation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Describes an anomaly or error with the application.
*/
public class Observation private constructor(builder: Builder) {
/**
* The detail type of the CloudWatch Event-based observation, for example, `EC2 Instance State-change Notification`.
*/
public val cloudWatchEventDetailType: kotlin.String? = builder.cloudWatchEventDetailType
/**
* The ID of the CloudWatch Event-based observation related to the detected problem.
*/
public val cloudWatchEventId: kotlin.String? = builder.cloudWatchEventId
/**
* The source of the CloudWatch Event.
*/
public val cloudWatchEventSource: aws.sdk.kotlin.services.applicationinsights.model.CloudWatchEventSource? = builder.cloudWatchEventSource
/**
* The CodeDeploy application to which the deployment belongs.
*/
public val codeDeployApplication: kotlin.String? = builder.codeDeployApplication
/**
* The deployment group to which the CodeDeploy deployment belongs.
*/
public val codeDeployDeploymentGroup: kotlin.String? = builder.codeDeployDeploymentGroup
/**
* The deployment ID of the CodeDeploy-based observation related to the detected problem.
*/
public val codeDeployDeploymentId: kotlin.String? = builder.codeDeployDeploymentId
/**
* The instance group to which the CodeDeploy instance belongs.
*/
public val codeDeployInstanceGroupId: kotlin.String? = builder.codeDeployInstanceGroupId
/**
* The status of the CodeDeploy deployment, for example `SUCCESS` or ` FAILURE`.
*/
public val codeDeployState: kotlin.String? = builder.codeDeployState
/**
* The cause of an EBS CloudWatch event.
*/
public val ebsCause: kotlin.String? = builder.ebsCause
/**
* The type of EBS CloudWatch event, such as `createVolume`, `deleteVolume` or `attachVolume`.
*/
public val ebsEvent: kotlin.String? = builder.ebsEvent
/**
* The request ID of an EBS CloudWatch event.
*/
public val ebsRequestId: kotlin.String? = builder.ebsRequestId
/**
* The result of an EBS CloudWatch event, such as `failed` or `succeeded`.
*/
public val ebsResult: kotlin.String? = builder.ebsResult
/**
* The state of the instance, such as `STOPPING` or `TERMINATING`.
*/
public val ec2State: kotlin.String? = builder.ec2State
/**
* The time when the observation ended, in epoch seconds.
*/
public val endTime: aws.smithy.kotlin.runtime.time.Instant? = builder.endTime
/**
* The Amazon Resource Name (ARN) of the AWS Health Event-based observation.
*/
public val healthEventArn: kotlin.String? = builder.healthEventArn
/**
* The description of the AWS Health event provided by the service, such as Amazon EC2.
*/
public val healthEventDescription: kotlin.String? = builder.healthEventDescription
/**
* The category of the AWS Health event, such as `issue`.
*/
public val healthEventTypeCategory: kotlin.String? = builder.healthEventTypeCategory
/**
* The type of the AWS Health event, for example, `AWS_EC2_POWER_CONNECTIVITY_ISSUE`.
*/
public val healthEventTypeCode: kotlin.String? = builder.healthEventTypeCode
/**
* The service to which the AWS Health Event belongs, such as EC2.
*/
public val healthService: kotlin.String? = builder.healthService
/**
* The ID of the observation type.
*/
public val id: kotlin.String? = builder.id
/**
* The timestamp in the CloudWatch Logs that specifies when the matched line occurred.
*/
public val lineTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lineTime
/**
* The log filter of the observation.
*/
public val logFilter: aws.sdk.kotlin.services.applicationinsights.model.LogFilter? = builder.logFilter
/**
* The log group name.
*/
public val logGroup: kotlin.String? = builder.logGroup
/**
* The log text of the observation.
*/
public val logText: kotlin.String? = builder.logText
/**
* The name of the observation metric.
*/
public val metricName: kotlin.String? = builder.metricName
/**
* The namespace of the observation metric.
*/
public val metricNamespace: kotlin.String? = builder.metricNamespace
/**
* The category of an RDS event.
*/
public val rdsEventCategories: kotlin.String? = builder.rdsEventCategories
/**
* The message of an RDS event.
*/
public val rdsEventMessage: kotlin.String? = builder.rdsEventMessage
/**
* The name of the S3 CloudWatch Event-based observation.
*/
public val s3EventName: kotlin.String? = builder.s3EventName
/**
* The source resource ARN of the observation.
*/
public val sourceArn: kotlin.String? = builder.sourceArn
/**
* The source type of the observation.
*/
public val sourceType: kotlin.String? = builder.sourceType
/**
* The time when the observation was first detected, in epoch seconds.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The Amazon Resource Name (ARN) of the step function-based observation.
*/
public val statesArn: kotlin.String? = builder.statesArn
/**
* The Amazon Resource Name (ARN) of the step function execution-based observation.
*/
public val statesExecutionArn: kotlin.String? = builder.statesExecutionArn
/**
* The input to the step function-based observation.
*/
public val statesInput: kotlin.String? = builder.statesInput
/**
* The status of the step function-related observation.
*/
public val statesStatus: kotlin.String? = builder.statesStatus
/**
* The unit of the source observation metric.
*/
public val unit: kotlin.String? = builder.unit
/**
* The value of the source observation metric.
*/
public val value: kotlin.Double? = builder.value
/**
* The X-Ray request error percentage for this node.
*/
public val xRayErrorPercent: kotlin.Int? = builder.xRayErrorPercent
/**
* The X-Ray request fault percentage for this node.
*/
public val xRayFaultPercent: kotlin.Int? = builder.xRayFaultPercent
/**
* The name of the X-Ray node.
*/
public val xRayNodeName: kotlin.String? = builder.xRayNodeName
/**
* The type of the X-Ray node.
*/
public val xRayNodeType: kotlin.String? = builder.xRayNodeType
/**
* The X-Ray node request average latency for this node.
*/
public val xRayRequestAverageLatency: kotlin.Long? = builder.xRayRequestAverageLatency
/**
* The X-Ray request count for this node.
*/
public val xRayRequestCount: kotlin.Int? = builder.xRayRequestCount
/**
* The X-Ray request throttle percentage for this node.
*/
public val xRayThrottlePercent: kotlin.Int? = builder.xRayThrottlePercent
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationinsights.model.Observation = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Observation(")
append("cloudWatchEventDetailType=$cloudWatchEventDetailType,")
append("cloudWatchEventId=$cloudWatchEventId,")
append("cloudWatchEventSource=$cloudWatchEventSource,")
append("codeDeployApplication=$codeDeployApplication,")
append("codeDeployDeploymentGroup=$codeDeployDeploymentGroup,")
append("codeDeployDeploymentId=$codeDeployDeploymentId,")
append("codeDeployInstanceGroupId=$codeDeployInstanceGroupId,")
append("codeDeployState=$codeDeployState,")
append("ebsCause=$ebsCause,")
append("ebsEvent=$ebsEvent,")
append("ebsRequestId=$ebsRequestId,")
append("ebsResult=$ebsResult,")
append("ec2State=$ec2State,")
append("endTime=$endTime,")
append("healthEventArn=$healthEventArn,")
append("healthEventDescription=$healthEventDescription,")
append("healthEventTypeCategory=$healthEventTypeCategory,")
append("healthEventTypeCode=$healthEventTypeCode,")
append("healthService=$healthService,")
append("id=$id,")
append("lineTime=$lineTime,")
append("logFilter=$logFilter,")
append("logGroup=$logGroup,")
append("logText=$logText,")
append("metricName=$metricName,")
append("metricNamespace=$metricNamespace,")
append("rdsEventCategories=$rdsEventCategories,")
append("rdsEventMessage=$rdsEventMessage,")
append("s3EventName=$s3EventName,")
append("sourceArn=$sourceArn,")
append("sourceType=$sourceType,")
append("startTime=$startTime,")
append("statesArn=$statesArn,")
append("statesExecutionArn=$statesExecutionArn,")
append("statesInput=$statesInput,")
append("statesStatus=$statesStatus,")
append("unit=$unit,")
append("value=$value,")
append("xRayErrorPercent=$xRayErrorPercent,")
append("xRayFaultPercent=$xRayFaultPercent,")
append("xRayNodeName=$xRayNodeName,")
append("xRayNodeType=$xRayNodeType,")
append("xRayRequestAverageLatency=$xRayRequestAverageLatency,")
append("xRayRequestCount=$xRayRequestCount,")
append("xRayThrottlePercent=$xRayThrottlePercent")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cloudWatchEventDetailType?.hashCode() ?: 0
result = 31 * result + (cloudWatchEventId?.hashCode() ?: 0)
result = 31 * result + (cloudWatchEventSource?.hashCode() ?: 0)
result = 31 * result + (codeDeployApplication?.hashCode() ?: 0)
result = 31 * result + (codeDeployDeploymentGroup?.hashCode() ?: 0)
result = 31 * result + (codeDeployDeploymentId?.hashCode() ?: 0)
result = 31 * result + (codeDeployInstanceGroupId?.hashCode() ?: 0)
result = 31 * result + (codeDeployState?.hashCode() ?: 0)
result = 31 * result + (ebsCause?.hashCode() ?: 0)
result = 31 * result + (ebsEvent?.hashCode() ?: 0)
result = 31 * result + (ebsRequestId?.hashCode() ?: 0)
result = 31 * result + (ebsResult?.hashCode() ?: 0)
result = 31 * result + (ec2State?.hashCode() ?: 0)
result = 31 * result + (endTime?.hashCode() ?: 0)
result = 31 * result + (healthEventArn?.hashCode() ?: 0)
result = 31 * result + (healthEventDescription?.hashCode() ?: 0)
result = 31 * result + (healthEventTypeCategory?.hashCode() ?: 0)
result = 31 * result + (healthEventTypeCode?.hashCode() ?: 0)
result = 31 * result + (healthService?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (lineTime?.hashCode() ?: 0)
result = 31 * result + (logFilter?.hashCode() ?: 0)
result = 31 * result + (logGroup?.hashCode() ?: 0)
result = 31 * result + (logText?.hashCode() ?: 0)
result = 31 * result + (metricName?.hashCode() ?: 0)
result = 31 * result + (metricNamespace?.hashCode() ?: 0)
result = 31 * result + (rdsEventCategories?.hashCode() ?: 0)
result = 31 * result + (rdsEventMessage?.hashCode() ?: 0)
result = 31 * result + (s3EventName?.hashCode() ?: 0)
result = 31 * result + (sourceArn?.hashCode() ?: 0)
result = 31 * result + (sourceType?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (statesArn?.hashCode() ?: 0)
result = 31 * result + (statesExecutionArn?.hashCode() ?: 0)
result = 31 * result + (statesInput?.hashCode() ?: 0)
result = 31 * result + (statesStatus?.hashCode() ?: 0)
result = 31 * result + (unit?.hashCode() ?: 0)
result = 31 * result + (value?.hashCode() ?: 0)
result = 31 * result + (xRayErrorPercent ?: 0)
result = 31 * result + (xRayFaultPercent ?: 0)
result = 31 * result + (xRayNodeName?.hashCode() ?: 0)
result = 31 * result + (xRayNodeType?.hashCode() ?: 0)
result = 31 * result + (xRayRequestAverageLatency?.hashCode() ?: 0)
result = 31 * result + (xRayRequestCount ?: 0)
result = 31 * result + (xRayThrottlePercent ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Observation
if (cloudWatchEventDetailType != other.cloudWatchEventDetailType) return false
if (cloudWatchEventId != other.cloudWatchEventId) return false
if (cloudWatchEventSource != other.cloudWatchEventSource) return false
if (codeDeployApplication != other.codeDeployApplication) return false
if (codeDeployDeploymentGroup != other.codeDeployDeploymentGroup) return false
if (codeDeployDeploymentId != other.codeDeployDeploymentId) return false
if (codeDeployInstanceGroupId != other.codeDeployInstanceGroupId) return false
if (codeDeployState != other.codeDeployState) return false
if (ebsCause != other.ebsCause) return false
if (ebsEvent != other.ebsEvent) return false
if (ebsRequestId != other.ebsRequestId) return false
if (ebsResult != other.ebsResult) return false
if (ec2State != other.ec2State) return false
if (endTime != other.endTime) return false
if (healthEventArn != other.healthEventArn) return false
if (healthEventDescription != other.healthEventDescription) return false
if (healthEventTypeCategory != other.healthEventTypeCategory) return false
if (healthEventTypeCode != other.healthEventTypeCode) return false
if (healthService != other.healthService) return false
if (id != other.id) return false
if (lineTime != other.lineTime) return false
if (logFilter != other.logFilter) return false
if (logGroup != other.logGroup) return false
if (logText != other.logText) return false
if (metricName != other.metricName) return false
if (metricNamespace != other.metricNamespace) return false
if (rdsEventCategories != other.rdsEventCategories) return false
if (rdsEventMessage != other.rdsEventMessage) return false
if (s3EventName != other.s3EventName) return false
if (sourceArn != other.sourceArn) return false
if (sourceType != other.sourceType) return false
if (startTime != other.startTime) return false
if (statesArn != other.statesArn) return false
if (statesExecutionArn != other.statesExecutionArn) return false
if (statesInput != other.statesInput) return false
if (statesStatus != other.statesStatus) return false
if (unit != other.unit) return false
if (!(value?.equals(other.value) ?: (other.value == null))) return false
if (xRayErrorPercent != other.xRayErrorPercent) return false
if (xRayFaultPercent != other.xRayFaultPercent) return false
if (xRayNodeName != other.xRayNodeName) return false
if (xRayNodeType != other.xRayNodeType) return false
if (xRayRequestAverageLatency != other.xRayRequestAverageLatency) return false
if (xRayRequestCount != other.xRayRequestCount) return false
if (xRayThrottlePercent != other.xRayThrottlePercent) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationinsights.model.Observation = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The detail type of the CloudWatch Event-based observation, for example, `EC2 Instance State-change Notification`.
*/
public var cloudWatchEventDetailType: kotlin.String? = null
/**
* The ID of the CloudWatch Event-based observation related to the detected problem.
*/
public var cloudWatchEventId: kotlin.String? = null
/**
* The source of the CloudWatch Event.
*/
public var cloudWatchEventSource: aws.sdk.kotlin.services.applicationinsights.model.CloudWatchEventSource? = null
/**
* The CodeDeploy application to which the deployment belongs.
*/
public var codeDeployApplication: kotlin.String? = null
/**
* The deployment group to which the CodeDeploy deployment belongs.
*/
public var codeDeployDeploymentGroup: kotlin.String? = null
/**
* The deployment ID of the CodeDeploy-based observation related to the detected problem.
*/
public var codeDeployDeploymentId: kotlin.String? = null
/**
* The instance group to which the CodeDeploy instance belongs.
*/
public var codeDeployInstanceGroupId: kotlin.String? = null
/**
* The status of the CodeDeploy deployment, for example `SUCCESS` or ` FAILURE`.
*/
public var codeDeployState: kotlin.String? = null
/**
* The cause of an EBS CloudWatch event.
*/
public var ebsCause: kotlin.String? = null
/**
* The type of EBS CloudWatch event, such as `createVolume`, `deleteVolume` or `attachVolume`.
*/
public var ebsEvent: kotlin.String? = null
/**
* The request ID of an EBS CloudWatch event.
*/
public var ebsRequestId: kotlin.String? = null
/**
* The result of an EBS CloudWatch event, such as `failed` or `succeeded`.
*/
public var ebsResult: kotlin.String? = null
/**
* The state of the instance, such as `STOPPING` or `TERMINATING`.
*/
public var ec2State: kotlin.String? = null
/**
* The time when the observation ended, in epoch seconds.
*/
public var endTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the AWS Health Event-based observation.
*/
public var healthEventArn: kotlin.String? = null
/**
* The description of the AWS Health event provided by the service, such as Amazon EC2.
*/
public var healthEventDescription: kotlin.String? = null
/**
* The category of the AWS Health event, such as `issue`.
*/
public var healthEventTypeCategory: kotlin.String? = null
/**
* The type of the AWS Health event, for example, `AWS_EC2_POWER_CONNECTIVITY_ISSUE`.
*/
public var healthEventTypeCode: kotlin.String? = null
/**
* The service to which the AWS Health Event belongs, such as EC2.
*/
public var healthService: kotlin.String? = null
/**
* The ID of the observation type.
*/
public var id: kotlin.String? = null
/**
* The timestamp in the CloudWatch Logs that specifies when the matched line occurred.
*/
public var lineTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The log filter of the observation.
*/
public var logFilter: aws.sdk.kotlin.services.applicationinsights.model.LogFilter? = null
/**
* The log group name.
*/
public var logGroup: kotlin.String? = null
/**
* The log text of the observation.
*/
public var logText: kotlin.String? = null
/**
* The name of the observation metric.
*/
public var metricName: kotlin.String? = null
/**
* The namespace of the observation metric.
*/
public var metricNamespace: kotlin.String? = null
/**
* The category of an RDS event.
*/
public var rdsEventCategories: kotlin.String? = null
/**
* The message of an RDS event.
*/
public var rdsEventMessage: kotlin.String? = null
/**
* The name of the S3 CloudWatch Event-based observation.
*/
public var s3EventName: kotlin.String? = null
/**
* The source resource ARN of the observation.
*/
public var sourceArn: kotlin.String? = null
/**
* The source type of the observation.
*/
public var sourceType: kotlin.String? = null
/**
* The time when the observation was first detected, in epoch seconds.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the step function-based observation.
*/
public var statesArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the step function execution-based observation.
*/
public var statesExecutionArn: kotlin.String? = null
/**
* The input to the step function-based observation.
*/
public var statesInput: kotlin.String? = null
/**
* The status of the step function-related observation.
*/
public var statesStatus: kotlin.String? = null
/**
* The unit of the source observation metric.
*/
public var unit: kotlin.String? = null
/**
* The value of the source observation metric.
*/
public var value: kotlin.Double? = null
/**
* The X-Ray request error percentage for this node.
*/
public var xRayErrorPercent: kotlin.Int? = null
/**
* The X-Ray request fault percentage for this node.
*/
public var xRayFaultPercent: kotlin.Int? = null
/**
* The name of the X-Ray node.
*/
public var xRayNodeName: kotlin.String? = null
/**
* The type of the X-Ray node.
*/
public var xRayNodeType: kotlin.String? = null
/**
* The X-Ray node request average latency for this node.
*/
public var xRayRequestAverageLatency: kotlin.Long? = null
/**
* The X-Ray request count for this node.
*/
public var xRayRequestCount: kotlin.Int? = null
/**
* The X-Ray request throttle percentage for this node.
*/
public var xRayThrottlePercent: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationinsights.model.Observation) : this() {
this.cloudWatchEventDetailType = x.cloudWatchEventDetailType
this.cloudWatchEventId = x.cloudWatchEventId
this.cloudWatchEventSource = x.cloudWatchEventSource
this.codeDeployApplication = x.codeDeployApplication
this.codeDeployDeploymentGroup = x.codeDeployDeploymentGroup
this.codeDeployDeploymentId = x.codeDeployDeploymentId
this.codeDeployInstanceGroupId = x.codeDeployInstanceGroupId
this.codeDeployState = x.codeDeployState
this.ebsCause = x.ebsCause
this.ebsEvent = x.ebsEvent
this.ebsRequestId = x.ebsRequestId
this.ebsResult = x.ebsResult
this.ec2State = x.ec2State
this.endTime = x.endTime
this.healthEventArn = x.healthEventArn
this.healthEventDescription = x.healthEventDescription
this.healthEventTypeCategory = x.healthEventTypeCategory
this.healthEventTypeCode = x.healthEventTypeCode
this.healthService = x.healthService
this.id = x.id
this.lineTime = x.lineTime
this.logFilter = x.logFilter
this.logGroup = x.logGroup
this.logText = x.logText
this.metricName = x.metricName
this.metricNamespace = x.metricNamespace
this.rdsEventCategories = x.rdsEventCategories
this.rdsEventMessage = x.rdsEventMessage
this.s3EventName = x.s3EventName
this.sourceArn = x.sourceArn
this.sourceType = x.sourceType
this.startTime = x.startTime
this.statesArn = x.statesArn
this.statesExecutionArn = x.statesExecutionArn
this.statesInput = x.statesInput
this.statesStatus = x.statesStatus
this.unit = x.unit
this.value = x.value
this.xRayErrorPercent = x.xRayErrorPercent
this.xRayFaultPercent = x.xRayFaultPercent
this.xRayNodeName = x.xRayNodeName
this.xRayNodeType = x.xRayNodeType
this.xRayRequestAverageLatency = x.xRayRequestAverageLatency
this.xRayRequestCount = x.xRayRequestCount
this.xRayThrottlePercent = x.xRayThrottlePercent
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationinsights.model.Observation = Observation(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy