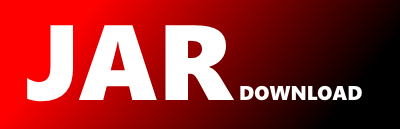
commonMain.aws.sdk.kotlin.services.applicationinsights.model.Tier.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import kotlin.collections.List
public sealed class Tier {
public abstract val value: kotlin.String
public object ActiveDirectory : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "ACTIVE_DIRECTORY"
override fun toString(): kotlin.String = "ActiveDirectory"
}
public object Custom : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "CUSTOM"
override fun toString(): kotlin.String = "Custom"
}
public object Default : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "DEFAULT"
override fun toString(): kotlin.String = "Default"
}
public object DotNetCore : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "DOT_NET_CORE"
override fun toString(): kotlin.String = "DotNetCore"
}
public object DotNetWeb : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "DOT_NET_WEB"
override fun toString(): kotlin.String = "DotNetWeb"
}
public object DotNetWebTier : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "DOT_NET_WEB_TIER"
override fun toString(): kotlin.String = "DotNetWebTier"
}
public object DotNetWorker : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "DOT_NET_WORKER"
override fun toString(): kotlin.String = "DotNetWorker"
}
public object JavaJmx : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "JAVA_JMX"
override fun toString(): kotlin.String = "JavaJmx"
}
public object Mysql : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "MYSQL"
override fun toString(): kotlin.String = "Mysql"
}
public object Oracle : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "ORACLE"
override fun toString(): kotlin.String = "Oracle"
}
public object Postgresql : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "POSTGRESQL"
override fun toString(): kotlin.String = "Postgresql"
}
public object SapHanaHighAvailability : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_HANA_HIGH_AVAILABILITY"
override fun toString(): kotlin.String = "SapHanaHighAvailability"
}
public object SapHanaMultiNode : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_HANA_MULTI_NODE"
override fun toString(): kotlin.String = "SapHanaMultiNode"
}
public object SapHanaSingleNode : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_HANA_SINGLE_NODE"
override fun toString(): kotlin.String = "SapHanaSingleNode"
}
public object SapNetweaverDistributed : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_NETWEAVER_DISTRIBUTED"
override fun toString(): kotlin.String = "SapNetweaverDistributed"
}
public object SapNetweaverHighAvailability : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_NETWEAVER_HIGH_AVAILABILITY"
override fun toString(): kotlin.String = "SapNetweaverHighAvailability"
}
public object SapNetweaverStandard : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SAP_NETWEAVER_STANDARD"
override fun toString(): kotlin.String = "SapNetweaverStandard"
}
public object Sharepoint : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SHAREPOINT"
override fun toString(): kotlin.String = "Sharepoint"
}
public object SqlServer : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SQL_SERVER"
override fun toString(): kotlin.String = "SqlServer"
}
public object SqlServerAlwaysonAvailabilityGroup : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SQL_SERVER_ALWAYSON_AVAILABILITY_GROUP"
override fun toString(): kotlin.String = "SqlServerAlwaysonAvailabilityGroup"
}
public object SqlServerFailoverClusterInstance : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override val value: kotlin.String = "SQL_SERVER_FAILOVER_CLUSTER_INSTANCE"
override fun toString(): kotlin.String = "SqlServerFailoverClusterInstance"
}
public data class SdkUnknown(override val value: kotlin.String) : aws.sdk.kotlin.services.applicationinsights.model.Tier() {
override fun toString(): kotlin.String = "SdkUnknown($value)"
}
public companion object {
/**
* Convert a raw value to one of the sealed variants or [SdkUnknown]
*/
public fun fromValue(value: kotlin.String): aws.sdk.kotlin.services.applicationinsights.model.Tier = when (value) {
"ACTIVE_DIRECTORY" -> ActiveDirectory
"CUSTOM" -> Custom
"DEFAULT" -> Default
"DOT_NET_CORE" -> DotNetCore
"DOT_NET_WEB" -> DotNetWeb
"DOT_NET_WEB_TIER" -> DotNetWebTier
"DOT_NET_WORKER" -> DotNetWorker
"JAVA_JMX" -> JavaJmx
"MYSQL" -> Mysql
"ORACLE" -> Oracle
"POSTGRESQL" -> Postgresql
"SAP_HANA_HIGH_AVAILABILITY" -> SapHanaHighAvailability
"SAP_HANA_MULTI_NODE" -> SapHanaMultiNode
"SAP_HANA_SINGLE_NODE" -> SapHanaSingleNode
"SAP_NETWEAVER_DISTRIBUTED" -> SapNetweaverDistributed
"SAP_NETWEAVER_HIGH_AVAILABILITY" -> SapNetweaverHighAvailability
"SAP_NETWEAVER_STANDARD" -> SapNetweaverStandard
"SHAREPOINT" -> Sharepoint
"SQL_SERVER" -> SqlServer
"SQL_SERVER_ALWAYSON_AVAILABILITY_GROUP" -> SqlServerAlwaysonAvailabilityGroup
"SQL_SERVER_FAILOVER_CLUSTER_INSTANCE" -> SqlServerFailoverClusterInstance
else -> SdkUnknown(value)
}
/**
* Get a list of all possible variants
*/
public fun values(): kotlin.collections.List = values
private val values: kotlin.collections.List = listOf(
ActiveDirectory,
Custom,
Default,
DotNetCore,
DotNetWeb,
DotNetWebTier,
DotNetWorker,
JavaJmx,
Mysql,
Oracle,
Postgresql,
SapHanaHighAvailability,
SapHanaMultiNode,
SapHanaSingleNode,
SapNetweaverDistributed,
SapNetweaverHighAvailability,
SapNetweaverStandard,
Sharepoint,
SqlServer,
SqlServerAlwaysonAvailabilityGroup,
SqlServerFailoverClusterInstance,
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy