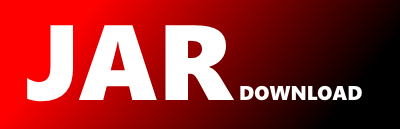
commonMain.aws.sdk.kotlin.services.applicationinsights.model.UpdateApplicationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationinsights-jvm Show documentation
Show all versions of applicationinsights-jvm Show documentation
The AWS SDK for Kotlin client for Application Insights
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationinsights.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateApplicationRequest private constructor(builder: Builder) {
/**
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*/
public val attachMissingPermission: kotlin.Boolean? = builder.attachMissingPermission
/**
* Turns auto-configuration on or off.
*/
public val autoConfigEnabled: kotlin.Boolean? = builder.autoConfigEnabled
/**
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as `instance terminated`, `failed deployment`, and others.
*/
public val cweMonitorEnabled: kotlin.Boolean? = builder.cweMonitorEnabled
/**
* When set to `true`, creates opsItems for any problems detected on an application.
*/
public val opsCenterEnabled: kotlin.Boolean? = builder.opsCenterEnabled
/**
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive notifications for updates to the opsItem.
*/
public val opsItemSnsTopicArn: kotlin.String? = builder.opsItemSnsTopicArn
/**
* Disassociates the SNS topic from the opsItem created for detected problems.
*/
public val removeSnsTopic: kotlin.Boolean? = builder.removeSnsTopic
/**
* The name of the resource group.
*/
public val resourceGroupName: kotlin.String? = builder.resourceGroupName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationinsights.model.UpdateApplicationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateApplicationRequest(")
append("attachMissingPermission=$attachMissingPermission,")
append("autoConfigEnabled=$autoConfigEnabled,")
append("cweMonitorEnabled=$cweMonitorEnabled,")
append("opsCenterEnabled=$opsCenterEnabled,")
append("opsItemSnsTopicArn=$opsItemSnsTopicArn,")
append("removeSnsTopic=$removeSnsTopic,")
append("resourceGroupName=$resourceGroupName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = attachMissingPermission?.hashCode() ?: 0
result = 31 * result + (autoConfigEnabled?.hashCode() ?: 0)
result = 31 * result + (cweMonitorEnabled?.hashCode() ?: 0)
result = 31 * result + (opsCenterEnabled?.hashCode() ?: 0)
result = 31 * result + (opsItemSnsTopicArn?.hashCode() ?: 0)
result = 31 * result + (removeSnsTopic?.hashCode() ?: 0)
result = 31 * result + (resourceGroupName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateApplicationRequest
if (attachMissingPermission != other.attachMissingPermission) return false
if (autoConfigEnabled != other.autoConfigEnabled) return false
if (cweMonitorEnabled != other.cweMonitorEnabled) return false
if (opsCenterEnabled != other.opsCenterEnabled) return false
if (opsItemSnsTopicArn != other.opsItemSnsTopicArn) return false
if (removeSnsTopic != other.removeSnsTopic) return false
if (resourceGroupName != other.resourceGroupName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationinsights.model.UpdateApplicationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*/
public var attachMissingPermission: kotlin.Boolean? = null
/**
* Turns auto-configuration on or off.
*/
public var autoConfigEnabled: kotlin.Boolean? = null
/**
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as `instance terminated`, `failed deployment`, and others.
*/
public var cweMonitorEnabled: kotlin.Boolean? = null
/**
* When set to `true`, creates opsItems for any problems detected on an application.
*/
public var opsCenterEnabled: kotlin.Boolean? = null
/**
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive notifications for updates to the opsItem.
*/
public var opsItemSnsTopicArn: kotlin.String? = null
/**
* Disassociates the SNS topic from the opsItem created for detected problems.
*/
public var removeSnsTopic: kotlin.Boolean? = null
/**
* The name of the resource group.
*/
public var resourceGroupName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationinsights.model.UpdateApplicationRequest) : this() {
this.attachMissingPermission = x.attachMissingPermission
this.autoConfigEnabled = x.autoConfigEnabled
this.cweMonitorEnabled = x.cweMonitorEnabled
this.opsCenterEnabled = x.opsCenterEnabled
this.opsItemSnsTopicArn = x.opsItemSnsTopicArn
this.removeSnsTopic = x.removeSnsTopic
this.resourceGroupName = x.resourceGroupName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationinsights.model.UpdateApplicationRequest = UpdateApplicationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy