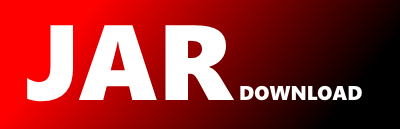
commonMain.aws.sdk.kotlin.services.applicationsignals.model.CreateServiceLevelObjectiveRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateServiceLevelObjectiveRequest private constructor(builder: Builder) {
/**
* An optional description for this SLO.
*/
public val description: kotlin.String? = builder.description
/**
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for evaluation and the attainment threshold.
*/
public val goal: aws.sdk.kotlin.services.applicationsignals.model.Goal? = builder.goal
/**
* A name for this SLO.
*/
public val name: kotlin.String? = builder.name
/**
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*/
public val sliConfig: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorConfig? = builder.sliConfig
/**
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able to associate tags with the SLO when you create the SLO, you must have the `cloudwatch:TagResource` permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by granting a user permission to access or change only resources with certain tag values.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationsignals.model.CreateServiceLevelObjectiveRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateServiceLevelObjectiveRequest(")
append("description=$description,")
append("goal=$goal,")
append("name=$name,")
append("sliConfig=$sliConfig,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (goal?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (sliConfig?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateServiceLevelObjectiveRequest
if (description != other.description) return false
if (goal != other.goal) return false
if (name != other.name) return false
if (sliConfig != other.sliConfig) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationsignals.model.CreateServiceLevelObjectiveRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* An optional description for this SLO.
*/
public var description: kotlin.String? = null
/**
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for evaluation and the attainment threshold.
*/
public var goal: aws.sdk.kotlin.services.applicationsignals.model.Goal? = null
/**
* A name for this SLO.
*/
public var name: kotlin.String? = null
/**
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*/
public var sliConfig: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorConfig? = null
/**
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able to associate tags with the SLO when you create the SLO, you must have the `cloudwatch:TagResource` permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by granting a user permission to access or change only resources with certain tag values.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationsignals.model.CreateServiceLevelObjectiveRequest) : this() {
this.description = x.description
this.goal = x.goal
this.name = x.name
this.sliConfig = x.sliConfig
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationsignals.model.CreateServiceLevelObjectiveRequest = CreateServiceLevelObjectiveRequest(this)
/**
* construct an [aws.sdk.kotlin.services.applicationsignals.model.Goal] inside the given [block]
*/
public fun goal(block: aws.sdk.kotlin.services.applicationsignals.model.Goal.Builder.() -> kotlin.Unit) {
this.goal = aws.sdk.kotlin.services.applicationsignals.model.Goal.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorConfig] inside the given [block]
*/
public fun sliConfig(block: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorConfig.Builder.() -> kotlin.Unit) {
this.sliConfig = aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy