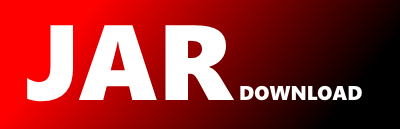
commonMain.aws.sdk.kotlin.services.applicationsignals.model.Goal.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* This structure contains the attributes that determine the goal of an SLO. This includes the time period for evaluation and the attainment threshold.
*/
public class Goal private constructor(builder: Builder) {
/**
* The threshold that determines if the goal is being met. An *attainment goal* is the ratio of good periods that meet the threshold requirements to the total periods within the interval. For example, an attainment goal of 99.9% means that within your interval, you are targeting 99.9% of the periods to be in healthy state.
*
* If you omit this parameter, 99 is used to represent 99% as the attainment goal.
*/
public val attainmentGoal: kotlin.Double? = builder.attainmentGoal
/**
* The time period used to evaluate the SLO. It can be either a calendar interval or rolling interval.
*
* If you omit this parameter, a rolling interval of 7 days is used.
*/
public val interval: aws.sdk.kotlin.services.applicationsignals.model.Interval? = builder.interval
/**
* The percentage of remaining budget over total budget that you want to get warnings for. If you omit this parameter, the default of 50.0 is used.
*/
public val warningThreshold: kotlin.Double? = builder.warningThreshold
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationsignals.model.Goal = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Goal(")
append("attainmentGoal=$attainmentGoal,")
append("interval=$interval,")
append("warningThreshold=$warningThreshold")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = attainmentGoal?.hashCode() ?: 0
result = 31 * result + (interval?.hashCode() ?: 0)
result = 31 * result + (warningThreshold?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Goal
if (attainmentGoal != other.attainmentGoal) return false
if (interval != other.interval) return false
if (warningThreshold != other.warningThreshold) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationsignals.model.Goal = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The threshold that determines if the goal is being met. An *attainment goal* is the ratio of good periods that meet the threshold requirements to the total periods within the interval. For example, an attainment goal of 99.9% means that within your interval, you are targeting 99.9% of the periods to be in healthy state.
*
* If you omit this parameter, 99 is used to represent 99% as the attainment goal.
*/
public var attainmentGoal: kotlin.Double? = null
/**
* The time period used to evaluate the SLO. It can be either a calendar interval or rolling interval.
*
* If you omit this parameter, a rolling interval of 7 days is used.
*/
public var interval: aws.sdk.kotlin.services.applicationsignals.model.Interval? = null
/**
* The percentage of remaining budget over total budget that you want to get warnings for. If you omit this parameter, the default of 50.0 is used.
*/
public var warningThreshold: kotlin.Double? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationsignals.model.Goal) : this() {
this.attainmentGoal = x.attainmentGoal
this.interval = x.interval
this.warningThreshold = x.warningThreshold
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationsignals.model.Goal = Goal(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy