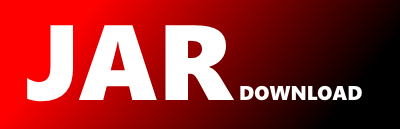
commonMain.aws.sdk.kotlin.services.applicationsignals.model.ServiceDependent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* This structure contains information about a service dependent that was discovered by Application Signals. A dependent is an entity that invoked the specified service during the provided time range. Dependents include other services, CloudWatch Synthetics canaries, and clients that are instrumented with CloudWatch RUM app monitors.
*/
public class ServiceDependent private constructor(builder: Builder) {
/**
* This is a string-to-string map. It can include the following fields.
* + `Type` designates the type of object this is.
* + `ResourceType` specifies the type of the resource. This field is used only when the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Name` specifies the name of the object. This is used only if the value of the `Type` field is `Service`, `RemoteService`, or `AWS::Service`.
* + `Identifier` identifies the resource objects of this resource. This is used only if the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Environment` specifies the location where this object is hosted, or what it belongs to.
*/
public val dependentKeyAttributes: Map = requireNotNull(builder.dependentKeyAttributes) { "A non-null value must be provided for dependentKeyAttributes" }
/**
* If the dependent invoker was a service that invoked it from an operation, the name of that dependent operation is displayed here.
*/
public val dependentOperationName: kotlin.String? = builder.dependentOperationName
/**
* An array of structures that each contain information about one metric associated with this service dependent that was discovered by Application Signals.
*/
public val metricReferences: List = requireNotNull(builder.metricReferences) { "A non-null value must be provided for metricReferences" }
/**
* If the invoked entity is an operation on an entity, the name of that dependent operation is displayed here.
*/
public val operationName: kotlin.String? = builder.operationName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationsignals.model.ServiceDependent = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ServiceDependent(")
append("dependentKeyAttributes=$dependentKeyAttributes,")
append("dependentOperationName=$dependentOperationName,")
append("metricReferences=$metricReferences,")
append("operationName=$operationName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dependentKeyAttributes.hashCode()
result = 31 * result + (dependentOperationName?.hashCode() ?: 0)
result = 31 * result + (metricReferences.hashCode())
result = 31 * result + (operationName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ServiceDependent
if (dependentKeyAttributes != other.dependentKeyAttributes) return false
if (dependentOperationName != other.dependentOperationName) return false
if (metricReferences != other.metricReferences) return false
if (operationName != other.operationName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationsignals.model.ServiceDependent = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* This is a string-to-string map. It can include the following fields.
* + `Type` designates the type of object this is.
* + `ResourceType` specifies the type of the resource. This field is used only when the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Name` specifies the name of the object. This is used only if the value of the `Type` field is `Service`, `RemoteService`, or `AWS::Service`.
* + `Identifier` identifies the resource objects of this resource. This is used only if the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Environment` specifies the location where this object is hosted, or what it belongs to.
*/
public var dependentKeyAttributes: Map? = null
/**
* If the dependent invoker was a service that invoked it from an operation, the name of that dependent operation is displayed here.
*/
public var dependentOperationName: kotlin.String? = null
/**
* An array of structures that each contain information about one metric associated with this service dependent that was discovered by Application Signals.
*/
public var metricReferences: List? = null
/**
* If the invoked entity is an operation on an entity, the name of that dependent operation is displayed here.
*/
public var operationName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationsignals.model.ServiceDependent) : this() {
this.dependentKeyAttributes = x.dependentKeyAttributes
this.dependentOperationName = x.dependentOperationName
this.metricReferences = x.metricReferences
this.operationName = x.operationName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationsignals.model.ServiceDependent = ServiceDependent(this)
internal fun correctErrors(): Builder {
if (dependentKeyAttributes == null) dependentKeyAttributes = emptyMap()
if (metricReferences == null) metricReferences = emptyList()
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy