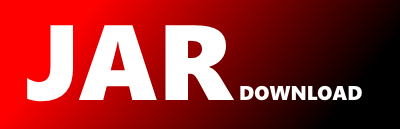
commonMain.aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Use this structure to specify the information for the metric that the SLO will monitor.
*/
public class ServiceLevelIndicatorMetricConfig private constructor(builder: Builder) {
/**
* If this SLO is related to a metric collected by Application Signals, you must use this field to specify which service the SLO metric is related to. To do so, you must specify at least the `Type`, `Name`, and `Environment` attributes.
*
* This is a string-to-string map. It can include the following fields.
* + `Type` designates the type of object this is.
* + `ResourceType` specifies the type of the resource. This field is used only when the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Name` specifies the name of the object. This is used only if the value of the `Type` field is `Service`, `RemoteService`, or `AWS::Service`.
* + `Identifier` identifies the resource objects of this resource. This is used only if the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Environment` specifies the location where this object is hosted, or what it belongs to.
*/
public val keyAttributes: Map? = builder.keyAttributes
/**
* If this SLO monitors a CloudWatch metric or the result of a CloudWatch metric math expression, use this structure to specify that metric or expression.
*/
public val metricDataQueries: List? = builder.metricDataQueries
/**
* If the SLO is to monitor either the `LATENCY` or `AVAILABILITY` metric that Application Signals collects, use this field to specify which of those metrics is used.
*/
public val metricType: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricType? = builder.metricType
/**
* If the SLO is to monitor a specific operation of the service, use this field to specify the name of that operation.
*/
public val operationName: kotlin.String? = builder.operationName
/**
* The number of seconds to use as the period for SLO evaluation. Your application's performance is compared to the SLI during each period. For each period, the application is determined to have either achieved or not achieved the necessary performance.
*/
public val periodSeconds: kotlin.Int? = builder.periodSeconds
/**
* The statistic to use for comparison to the threshold. It can be any CloudWatch statistic or extended statistic. For more information about statistics, see [CloudWatch statistics definitions](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/Statistics-definitions.html).
*/
public val statistic: kotlin.String? = builder.statistic
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ServiceLevelIndicatorMetricConfig(")
append("keyAttributes=$keyAttributes,")
append("metricDataQueries=$metricDataQueries,")
append("metricType=$metricType,")
append("operationName=$operationName,")
append("periodSeconds=$periodSeconds,")
append("statistic=$statistic")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = keyAttributes?.hashCode() ?: 0
result = 31 * result + (metricDataQueries?.hashCode() ?: 0)
result = 31 * result + (metricType?.hashCode() ?: 0)
result = 31 * result + (operationName?.hashCode() ?: 0)
result = 31 * result + (periodSeconds ?: 0)
result = 31 * result + (statistic?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ServiceLevelIndicatorMetricConfig
if (keyAttributes != other.keyAttributes) return false
if (metricDataQueries != other.metricDataQueries) return false
if (metricType != other.metricType) return false
if (operationName != other.operationName) return false
if (periodSeconds != other.periodSeconds) return false
if (statistic != other.statistic) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricConfig = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* If this SLO is related to a metric collected by Application Signals, you must use this field to specify which service the SLO metric is related to. To do so, you must specify at least the `Type`, `Name`, and `Environment` attributes.
*
* This is a string-to-string map. It can include the following fields.
* + `Type` designates the type of object this is.
* + `ResourceType` specifies the type of the resource. This field is used only when the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Name` specifies the name of the object. This is used only if the value of the `Type` field is `Service`, `RemoteService`, or `AWS::Service`.
* + `Identifier` identifies the resource objects of this resource. This is used only if the value of the `Type` field is `Resource` or `AWS::Resource`.
* + `Environment` specifies the location where this object is hosted, or what it belongs to.
*/
public var keyAttributes: Map? = null
/**
* If this SLO monitors a CloudWatch metric or the result of a CloudWatch metric math expression, use this structure to specify that metric or expression.
*/
public var metricDataQueries: List? = null
/**
* If the SLO is to monitor either the `LATENCY` or `AVAILABILITY` metric that Application Signals collects, use this field to specify which of those metrics is used.
*/
public var metricType: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricType? = null
/**
* If the SLO is to monitor a specific operation of the service, use this field to specify the name of that operation.
*/
public var operationName: kotlin.String? = null
/**
* The number of seconds to use as the period for SLO evaluation. Your application's performance is compared to the SLI during each period. For each period, the application is determined to have either achieved or not achieved the necessary performance.
*/
public var periodSeconds: kotlin.Int? = null
/**
* The statistic to use for comparison to the threshold. It can be any CloudWatch statistic or extended statistic. For more information about statistics, see [CloudWatch statistics definitions](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/Statistics-definitions.html).
*/
public var statistic: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricConfig) : this() {
this.keyAttributes = x.keyAttributes
this.metricDataQueries = x.metricDataQueries
this.metricType = x.metricType
this.operationName = x.operationName
this.periodSeconds = x.periodSeconds
this.statistic = x.statistic
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicatorMetricConfig = ServiceLevelIndicatorMetricConfig(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy