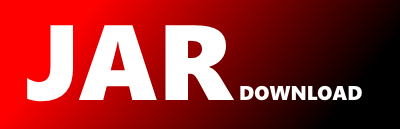
commonMain.aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A structure containing an SLO budget report that you have requested.
*/
public class ServiceLevelObjectiveBudgetReport private constructor(builder: Builder) {
/**
* The ARN of the SLO that this report is for.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's attainment goal, as of the time of the request.
*/
public val attainment: kotlin.Double? = builder.attainment
/**
* The budget amount remaining before the SLO status becomes `BREACHING`, at the time specified in the `Timestemp` parameter of the request. If this value is negative, then the SLO is already in `BREACHING` status.
*/
public val budgetSecondsRemaining: kotlin.Int? = builder.budgetSecondsRemaining
/**
* The status of this SLO, as it relates to the error budget for the entire time interval.
* + `OK` means that the SLO had remaining budget above the warning threshold, as of the time that you specified in `TimeStamp`.
* + `WARNING` means that the SLO's remaining budget was below the warning threshold, as of the time that you specified in `TimeStamp`.
* + `BREACHED` means that the SLO's budget was exhausted, as of the time that you specified in `TimeStamp`.
* + `INSUFFICIENT_DATA` means that the specifed start and end times were before the SLO was created, or that attainment data is missing.
*/
public val budgetStatus: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetStatus = requireNotNull(builder.budgetStatus) { "A non-null value must be provided for budgetStatus" }
/**
* This structure contains the attributes that determine the goal of an SLO. This includes the time period for evaluation and the attainment threshold.
*/
public val goal: aws.sdk.kotlin.services.applicationsignals.model.Goal? = builder.goal
/**
* The name of the SLO that this report is for.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* A structure that contains information about the performance metric that this SLO monitors.
*/
public val sli: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicator? = builder.sli
/**
* The total number of seconds in the error budget for the interval.
*/
public val totalBudgetSeconds: kotlin.Int? = builder.totalBudgetSeconds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ServiceLevelObjectiveBudgetReport(")
append("arn=$arn,")
append("attainment=$attainment,")
append("budgetSecondsRemaining=$budgetSecondsRemaining,")
append("budgetStatus=$budgetStatus,")
append("goal=$goal,")
append("name=$name,")
append("sli=$sli,")
append("totalBudgetSeconds=$totalBudgetSeconds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn.hashCode()
result = 31 * result + (attainment?.hashCode() ?: 0)
result = 31 * result + (budgetSecondsRemaining ?: 0)
result = 31 * result + (budgetStatus.hashCode())
result = 31 * result + (goal?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (sli?.hashCode() ?: 0)
result = 31 * result + (totalBudgetSeconds ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ServiceLevelObjectiveBudgetReport
if (arn != other.arn) return false
if (attainment != other.attainment) return false
if (budgetSecondsRemaining != other.budgetSecondsRemaining) return false
if (budgetStatus != other.budgetStatus) return false
if (goal != other.goal) return false
if (name != other.name) return false
if (sli != other.sli) return false
if (totalBudgetSeconds != other.totalBudgetSeconds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the SLO that this report is for.
*/
public var arn: kotlin.String? = null
/**
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's attainment goal, as of the time of the request.
*/
public var attainment: kotlin.Double? = null
/**
* The budget amount remaining before the SLO status becomes `BREACHING`, at the time specified in the `Timestemp` parameter of the request. If this value is negative, then the SLO is already in `BREACHING` status.
*/
public var budgetSecondsRemaining: kotlin.Int? = null
/**
* The status of this SLO, as it relates to the error budget for the entire time interval.
* + `OK` means that the SLO had remaining budget above the warning threshold, as of the time that you specified in `TimeStamp`.
* + `WARNING` means that the SLO's remaining budget was below the warning threshold, as of the time that you specified in `TimeStamp`.
* + `BREACHED` means that the SLO's budget was exhausted, as of the time that you specified in `TimeStamp`.
* + `INSUFFICIENT_DATA` means that the specifed start and end times were before the SLO was created, or that attainment data is missing.
*/
public var budgetStatus: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetStatus? = null
/**
* This structure contains the attributes that determine the goal of an SLO. This includes the time period for evaluation and the attainment threshold.
*/
public var goal: aws.sdk.kotlin.services.applicationsignals.model.Goal? = null
/**
* The name of the SLO that this report is for.
*/
public var name: kotlin.String? = null
/**
* A structure that contains information about the performance metric that this SLO monitors.
*/
public var sli: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicator? = null
/**
* The total number of seconds in the error budget for the interval.
*/
public var totalBudgetSeconds: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport) : this() {
this.arn = x.arn
this.attainment = x.attainment
this.budgetSecondsRemaining = x.budgetSecondsRemaining
this.budgetStatus = x.budgetStatus
this.goal = x.goal
this.name = x.name
this.sli = x.sli
this.totalBudgetSeconds = x.totalBudgetSeconds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport = ServiceLevelObjectiveBudgetReport(this)
/**
* construct an [aws.sdk.kotlin.services.applicationsignals.model.Goal] inside the given [block]
*/
public fun goal(block: aws.sdk.kotlin.services.applicationsignals.model.Goal.Builder.() -> kotlin.Unit) {
this.goal = aws.sdk.kotlin.services.applicationsignals.model.Goal.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicator] inside the given [block]
*/
public fun sli(block: aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicator.Builder.() -> kotlin.Unit) {
this.sli = aws.sdk.kotlin.services.applicationsignals.model.ServiceLevelIndicator.invoke(block)
}
internal fun correctErrors(): Builder {
if (arn == null) arn = ""
if (budgetStatus == null) budgetStatus = ServiceLevelObjectiveBudgetStatus.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy