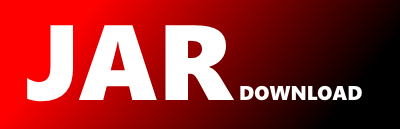
commonMain.aws.sdk.kotlin.services.applicationsignals.model.ServiceSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of applicationsignals-jvm Show documentation
Show all versions of applicationsignals-jvm Show documentation
The AWS SDK for Kotlin client for Application Signals
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.applicationsignals.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* This structure contains information about one of your services that was discoverd by Application Signals
*/
public class ServiceSummary private constructor(builder: Builder) {
/**
* This structure contains one or more string-to-string maps that help identify this service. It can include *platform attributes*, *application attributes*, and *telemetry attributes*.
*
* Platform attributes contain information the service's platform.
* + `PlatformType` defines the hosted-in platform.
* + `EKS.Cluster` is the name of the Amazon EKS cluster.
* + `K8s.Cluster` is the name of the self-hosted Kubernetes cluster.
* + `K8s.Namespace` is the name of the Kubernetes namespace in either Amazon EKS or Kubernetes clusters.
* + `K8s.Workload` is the name of the Kubernetes workload in either Amazon EKS or Kubernetes clusters.
* + `K8s.Node` is the name of the Kubernetes node in either Amazon EKS or Kubernetes clusters.
* + `K8s.Pod` is the name of the Kubernetes pod in either Amazon EKS or Kubernetes clusters.
* + `EC2.AutoScalingGroup` is the name of the Amazon EC2 Auto Scaling group.
* + `EC2.InstanceId` is the ID of the Amazon EC2 instance.
* + `Host` is the name of the host, for all platform types.
*
* Applciation attributes contain information about the application.
* + `AWS.Application` is the application's name in Amazon Web Services Service Catalog AppRegistry.
* + `AWS.Application.ARN` is the application's ARN in Amazon Web Services Service Catalog AppRegistry.
*
* Telemetry attributes contain telemetry information.
* + `Telemetry.SDK` is the fingerprint of the OpenTelemetry SDK version for instrumented services.
* + `Telemetry.Agent` is the fingerprint of the agent used to collect and send telemetry data.
* + `Telemetry.Source` Specifies the point of application where the telemetry was collected or specifies what was used for the source of telemetry data.
*/
public val attributeMaps: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy