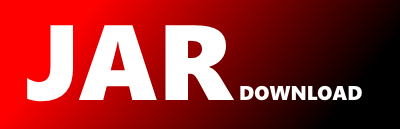
commonMain.aws.sdk.kotlin.services.appstream.AppStreamClient.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.internal.borrow
import aws.sdk.kotlin.runtime.endpoint.AwsEndpointResolver
import aws.sdk.kotlin.runtime.region.resolveRegion
import aws.sdk.kotlin.services.appstream.internal.DefaultEndpointResolver
import aws.sdk.kotlin.services.appstream.model.*
import aws.smithy.kotlin.runtime.SdkClient
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProvider
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigner
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkLogMode
import aws.smithy.kotlin.runtime.config.SdkClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.endpoints.EndpointResolver
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.StandardRetryStrategy
/**
* # Amazon AppStream 2.0
* This is the *Amazon AppStream 2.0 API Reference*. This documentation provides descriptions and syntax for each of the actions and data types in AppStream 2.0. AppStream 2.0 is a fully managed, secure application streaming service that lets you stream desktop applications to users without rewriting applications. AppStream 2.0 manages the AWS resources that are required to host and run your applications, scales automatically, and provides access to your users on demand.
*
* You can call the AppStream 2.0 API operations by using an interface VPC endpoint (interface endpoint). For more information, see [Access AppStream 2.0 API Operations and CLI Commands Through an Interface VPC Endpoint](https://docs.aws.amazon.com/appstream2/latest/developerguide/access-api-cli-through-interface-vpc-endpoint.html) in the *Amazon AppStream 2.0 Administration Guide*.
*
* To learn more about AppStream 2.0, see the following resources:
* + [Amazon AppStream 2.0 product page](http://aws.amazon.com/appstream2)
* + [Amazon AppStream 2.0 documentation](http://aws.amazon.com/documentation/appstream2)
*/
interface AppStreamClient : SdkClient {
override val serviceName: String
get() = "AppStream"
/**
* AppStreamClient's configuration
*/
val config: Config
companion object {
operator fun invoke(block: Config.Builder.() -> Unit): AppStreamClient {
val config = Config.Builder().apply(block).build()
return DefaultAppStreamClient(config)
}
operator fun invoke(config: Config): AppStreamClient = DefaultAppStreamClient(config)
/**
* Construct a [AppStreamClient] by resolving the configuration from the current environment.
*/
suspend fun fromEnvironment(block: (Config.Builder.() -> Unit)? = null): AppStreamClient {
val builder = Config.Builder()
if (block != null) builder.apply(block)
builder.region = builder.region ?: resolveRegion()
return DefaultAppStreamClient(builder.build())
}
}
class Config private constructor(builder: Builder): HttpClientConfig, SdkClientConfig {
val credentialsProvider: CredentialsProvider = builder.credentialsProvider?.borrow() ?: DefaultChainCredentialsProvider()
val endpointResolver: AwsEndpointResolver = builder.endpointResolver ?: DefaultEndpointResolver()
override val httpClientEngine: HttpClientEngine? = builder.httpClientEngine
val region: String = requireNotNull(builder.region) { "region is a required configuration property" }
val retryStrategy: RetryStrategy = StandardRetryStrategy()
override val sdkLogMode: SdkLogMode = builder.sdkLogMode
val signer: AwsSigner = builder.signer ?: DefaultAwsSigner
companion object {
inline operator fun invoke(block: Builder.() -> kotlin.Unit): Config = Builder().apply(block).build()
}
class Builder {
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
* NOTE: The caller is responsible for managing the lifetime of the provider when set. The SDK
* client will not close it when the client is closed.
*/
var credentialsProvider: CredentialsProvider? = null
/**
* Determines the endpoint (hostname) to make requests to. When not provided a default
* resolver is configured automatically. This is an advanced client option.
*/
var endpointResolver: AwsEndpointResolver? = null
/**
* Override the default HTTP client engine used to make SDK requests (e.g. configure proxy behavior, timeouts, concurrency, etc).
* NOTE: The caller is responsible for managing the lifetime of the engine when set. The SDK
* client will not close it when the client is closed.
*/
var httpClientEngine: HttpClientEngine? = null
/**
* AWS region to make requests to
*/
var region: String? = null
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
*
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
*
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
var sdkLogMode: SdkLogMode = SdkLogMode.Default
/**
* The implementation of AWS signer to use for signing requests
*/
var signer: AwsSigner? = null
@PublishedApi
internal fun build(): Config = Config(this)
}
}
/**
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*/
suspend fun associateApplicationFleet(input: AssociateApplicationFleetRequest): AssociateApplicationFleetResponse
/**
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*/
suspend fun associateApplicationFleet(block: AssociateApplicationFleetRequest.Builder.() -> Unit) = associateApplicationFleet(AssociateApplicationFleetRequest.Builder().apply(block).build())
/**
* Associates an application to entitle.
*/
suspend fun associateApplicationToEntitlement(input: AssociateApplicationToEntitlementRequest): AssociateApplicationToEntitlementResponse
/**
* Associates an application to entitle.
*/
suspend fun associateApplicationToEntitlement(block: AssociateApplicationToEntitlementRequest.Builder.() -> Unit) = associateApplicationToEntitlement(AssociateApplicationToEntitlementRequest.Builder().apply(block).build())
/**
* Associates the specified fleet with the specified stack.
*/
suspend fun associateFleet(input: AssociateFleetRequest): AssociateFleetResponse
/**
* Associates the specified fleet with the specified stack.
*/
suspend fun associateFleet(block: AssociateFleetRequest.Builder.() -> Unit) = associateFleet(AssociateFleetRequest.Builder().apply(block).build())
/**
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with fleets that are joined to an Active Directory domain.
*/
suspend fun batchAssociateUserStack(input: BatchAssociateUserStackRequest): BatchAssociateUserStackResponse
/**
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with fleets that are joined to an Active Directory domain.
*/
suspend fun batchAssociateUserStack(block: BatchAssociateUserStackRequest.Builder.() -> Unit) = batchAssociateUserStack(BatchAssociateUserStackRequest.Builder().apply(block).build())
/**
* Disassociates the specified users from the specified stacks.
*/
suspend fun batchDisassociateUserStack(input: BatchDisassociateUserStackRequest): BatchDisassociateUserStackResponse
/**
* Disassociates the specified users from the specified stacks.
*/
suspend fun batchDisassociateUserStack(block: BatchDisassociateUserStackRequest.Builder.() -> Unit) = batchDisassociateUserStack(BatchDisassociateUserStackRequest.Builder().apply(block).build())
/**
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you added to the image will not be copied.
*/
suspend fun copyImage(input: CopyImageRequest): CopyImageResponse
/**
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you added to the image will not be copied.
*/
suspend fun copyImage(block: CopyImageRequest.Builder.() -> Unit) = copyImage(CopyImageRequest.Builder().apply(block).build())
/**
* Creates an app block.
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3 bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard disk includes the application binaries and other files necessary to launch your applications. Multiple applications can be assigned to a single app block.
*
* This is only supported for Elastic fleets.
*/
suspend fun createAppBlock(input: CreateAppBlockRequest): CreateAppBlockResponse
/**
* Creates an app block.
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3 bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard disk includes the application binaries and other files necessary to launch your applications. Multiple applications can be assigned to a single app block.
*
* This is only supported for Elastic fleets.
*/
suspend fun createAppBlock(block: CreateAppBlockRequest.Builder.() -> Unit) = createAppBlock(CreateAppBlockRequest.Builder().apply(block).build())
/**
* Creates an application.
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on Elastic fleet streaming instances. An application consists of the launch details, icon, and display name. Applications are associated with an app block that contains the application binaries and other files. The applications assigned to an Elastic fleet are the applications users can launch.
*
* This is only supported for Elastic fleets.
*/
suspend fun createApplication(input: CreateApplicationRequest): CreateApplicationResponse
/**
* Creates an application.
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on Elastic fleet streaming instances. An application consists of the launch details, icon, and display name. Applications are associated with an app block that contains the application binaries and other files. The applications assigned to an Elastic fleet are the applications users can launch.
*
* This is only supported for Elastic fleets.
*/
suspend fun createApplication(block: CreateApplicationRequest.Builder.() -> Unit) = createApplication(CreateApplicationRequest.Builder().apply(block).build())
/**
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*/
suspend fun createDirectoryConfig(input: CreateDirectoryConfigRequest): CreateDirectoryConfigResponse
/**
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*/
suspend fun createDirectoryConfig(block: CreateDirectoryConfigRequest.Builder.() -> Unit) = createDirectoryConfig(CreateDirectoryConfigRequest.Builder().apply(block).build())
/**
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*/
suspend fun createEntitlement(input: CreateEntitlementRequest): CreateEntitlementResponse
/**
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*/
suspend fun createEntitlement(block: CreateEntitlementRequest.Builder.() -> Unit) = createEntitlement(CreateEntitlementRequest.Builder().apply(block).build())
/**
* Creates a fleet. A fleet consists of streaming instances that run a specified image when using Always-On or On-Demand.
*/
suspend fun createFleet(input: CreateFleetRequest): CreateFleetResponse
/**
* Creates a fleet. A fleet consists of streaming instances that run a specified image when using Always-On or On-Demand.
*/
suspend fun createFleet(block: CreateFleetRequest.Builder.() -> Unit) = createFleet(CreateFleetRequest.Builder().apply(block).build())
/**
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
* The initial state of the builder is `PENDING`. When it is ready, the state is `RUNNING`.
*/
suspend fun createImageBuilder(input: CreateImageBuilderRequest): CreateImageBuilderResponse
/**
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
* The initial state of the builder is `PENDING`. When it is ready, the state is `RUNNING`.
*/
suspend fun createImageBuilder(block: CreateImageBuilderRequest.Builder.() -> Unit) = createImageBuilder(CreateImageBuilderRequest.Builder().apply(block).build())
/**
* Creates a URL to start an image builder streaming session.
*/
suspend fun createImageBuilderStreamingUrl(input: CreateImageBuilderStreamingUrlRequest): CreateImageBuilderStreamingUrlResponse
/**
* Creates a URL to start an image builder streaming session.
*/
suspend fun createImageBuilderStreamingUrl(block: CreateImageBuilderStreamingUrlRequest.Builder.() -> Unit) = createImageBuilderStreamingUrl(CreateImageBuilderStreamingUrlRequest.Builder().apply(block).build())
/**
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access policies, and storage configurations.
*/
suspend fun createStack(input: CreateStackRequest): CreateStackResponse
/**
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access policies, and storage configurations.
*/
suspend fun createStack(block: CreateStackRequest.Builder.() -> Unit) = createStack(CreateStackRequest.Builder().apply(block).build())
/**
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL enables application streaming to be tested without user setup.
*/
suspend fun createStreamingUrl(input: CreateStreamingUrlRequest): CreateStreamingUrlResponse
/**
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL enables application streaming to be tested without user setup.
*/
suspend fun createStreamingUrl(block: CreateStreamingUrlRequest.Builder.() -> Unit) = createStreamingUrl(CreateStreamingUrlRequest.Builder().apply(block).build())
/**
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent software.
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in [Administer Your AppStream 2.0 Images](https://docs.aws.amazon.com/appstream2/latest/developerguide/administer-images.html), in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun createUpdatedImage(input: CreateUpdatedImageRequest): CreateUpdatedImageResponse
/**
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent software.
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in [Administer Your AppStream 2.0 Images](https://docs.aws.amazon.com/appstream2/latest/developerguide/administer-images.html), in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun createUpdatedImage(block: CreateUpdatedImageRequest.Builder.() -> Unit) = createUpdatedImage(CreateUpdatedImageRequest.Builder().apply(block).build())
/**
* Creates a usage report subscription. Usage reports are generated daily.
*/
suspend fun createUsageReportSubscription(input: CreateUsageReportSubscriptionRequest = CreateUsageReportSubscriptionRequest {}): CreateUsageReportSubscriptionResponse
/**
* Creates a usage report subscription. Usage reports are generated daily.
*/
suspend fun createUsageReportSubscription(block: CreateUsageReportSubscriptionRequest.Builder.() -> Unit) = createUsageReportSubscription(CreateUsageReportSubscriptionRequest.Builder().apply(block).build())
/**
* Creates a new user in the user pool.
*/
suspend fun createUser(input: CreateUserRequest): CreateUserResponse
/**
* Creates a new user in the user pool.
*/
suspend fun createUser(block: CreateUserRequest.Builder.() -> Unit) = createUser(CreateUserRequest.Builder().apply(block).build())
/**
* Deletes an app block.
*/
suspend fun deleteAppBlock(input: DeleteAppBlockRequest): DeleteAppBlockResponse
/**
* Deletes an app block.
*/
suspend fun deleteAppBlock(block: DeleteAppBlockRequest.Builder.() -> Unit) = deleteAppBlock(DeleteAppBlockRequest.Builder().apply(block).build())
/**
* Deletes an application.
*/
suspend fun deleteApplication(input: DeleteApplicationRequest): DeleteApplicationResponse
/**
* Deletes an application.
*/
suspend fun deleteApplication(block: DeleteApplicationRequest.Builder.() -> Unit) = deleteApplication(DeleteApplicationRequest.Builder().apply(block).build())
/**
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required to join streaming instances to an Active Directory domain.
*/
suspend fun deleteDirectoryConfig(input: DeleteDirectoryConfigRequest): DeleteDirectoryConfigResponse
/**
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required to join streaming instances to an Active Directory domain.
*/
suspend fun deleteDirectoryConfig(block: DeleteDirectoryConfigRequest.Builder.() -> Unit) = deleteDirectoryConfig(DeleteDirectoryConfigRequest.Builder().apply(block).build())
/**
* Deletes the specified entitlement.
*/
suspend fun deleteEntitlement(input: DeleteEntitlementRequest): DeleteEntitlementResponse
/**
* Deletes the specified entitlement.
*/
suspend fun deleteEntitlement(block: DeleteEntitlementRequest.Builder.() -> Unit) = deleteEntitlement(DeleteEntitlementRequest.Builder().apply(block).build())
/**
* Deletes the specified fleet.
*/
suspend fun deleteFleet(input: DeleteFleetRequest): DeleteFleetResponse
/**
* Deletes the specified fleet.
*/
suspend fun deleteFleet(block: DeleteFleetRequest.Builder.() -> Unit) = deleteFleet(DeleteFleetRequest.Builder().apply(block).build())
/**
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot provision new capacity using the image.
*/
suspend fun deleteImage(input: DeleteImageRequest): DeleteImageResponse
/**
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot provision new capacity using the image.
*/
suspend fun deleteImage(block: DeleteImageRequest.Builder.() -> Unit) = deleteImage(DeleteImageRequest.Builder().apply(block).build())
/**
* Deletes the specified image builder and releases the capacity.
*/
suspend fun deleteImageBuilder(input: DeleteImageBuilderRequest): DeleteImageBuilderResponse
/**
* Deletes the specified image builder and releases the capacity.
*/
suspend fun deleteImageBuilder(block: DeleteImageBuilderRequest.Builder.() -> Unit) = deleteImageBuilder(DeleteImageBuilderRequest.Builder().apply(block).build())
/**
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to which you previously granted these permissions can no longer use the image.
*/
suspend fun deleteImagePermissions(input: DeleteImagePermissionsRequest): DeleteImagePermissionsResponse
/**
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to which you previously granted these permissions can no longer use the image.
*/
suspend fun deleteImagePermissions(block: DeleteImagePermissionsRequest.Builder.() -> Unit) = deleteImagePermissions(DeleteImagePermissionsRequest.Builder().apply(block).build())
/**
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the stack is no longer available to users. Also, any reservations made for application streaming sessions for the stack are released.
*/
suspend fun deleteStack(input: DeleteStackRequest): DeleteStackResponse
/**
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the stack is no longer available to users. Also, any reservations made for application streaming sessions for the stack are released.
*/
suspend fun deleteStack(block: DeleteStackRequest.Builder.() -> Unit) = deleteStack(DeleteStackRequest.Builder().apply(block).build())
/**
* Disables usage report generation.
*/
suspend fun deleteUsageReportSubscription(input: DeleteUsageReportSubscriptionRequest = DeleteUsageReportSubscriptionRequest {}): DeleteUsageReportSubscriptionResponse
/**
* Disables usage report generation.
*/
suspend fun deleteUsageReportSubscription(block: DeleteUsageReportSubscriptionRequest.Builder.() -> Unit) = deleteUsageReportSubscription(DeleteUsageReportSubscriptionRequest.Builder().apply(block).build())
/**
* Deletes a user from the user pool.
*/
suspend fun deleteUser(input: DeleteUserRequest): DeleteUserResponse
/**
* Deletes a user from the user pool.
*/
suspend fun deleteUser(block: DeleteUserRequest.Builder.() -> Unit) = deleteUser(DeleteUserRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more app blocks.
*/
suspend fun describeAppBlocks(input: DescribeAppBlocksRequest = DescribeAppBlocksRequest {}): DescribeAppBlocksResponse
/**
* Retrieves a list that describes one or more app blocks.
*/
suspend fun describeAppBlocks(block: DescribeAppBlocksRequest.Builder.() -> Unit) = describeAppBlocks(DescribeAppBlocksRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName must be specified.
*/
suspend fun describeApplicationFleetAssociations(input: DescribeApplicationFleetAssociationsRequest = DescribeApplicationFleetAssociationsRequest {}): DescribeApplicationFleetAssociationsResponse
/**
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName must be specified.
*/
suspend fun describeApplicationFleetAssociations(block: DescribeApplicationFleetAssociationsRequest.Builder.() -> Unit) = describeApplicationFleetAssociations(DescribeApplicationFleetAssociationsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more applications.
*/
suspend fun describeApplications(input: DescribeApplicationsRequest = DescribeApplicationsRequest {}): DescribeApplicationsResponse
/**
* Retrieves a list that describes one or more applications.
*/
suspend fun describeApplications(block: DescribeApplicationsRequest.Builder.() -> Unit) = describeApplications(DescribeApplicationsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names for these objects are provided. Otherwise, all Directory Config objects in the account are described. These objects include the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*
* Although the response syntax in this topic includes the account password, this password is not returned in the actual response.
*/
suspend fun describeDirectoryConfigs(input: DescribeDirectoryConfigsRequest = DescribeDirectoryConfigsRequest {}): DescribeDirectoryConfigsResponse
/**
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names for these objects are provided. Otherwise, all Directory Config objects in the account are described. These objects include the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*
* Although the response syntax in this topic includes the account password, this password is not returned in the actual response.
*/
suspend fun describeDirectoryConfigs(block: DescribeDirectoryConfigsRequest.Builder.() -> Unit) = describeDirectoryConfigs(DescribeDirectoryConfigsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one of more entitlements.
*/
suspend fun describeEntitlements(input: DescribeEntitlementsRequest): DescribeEntitlementsResponse
/**
* Retrieves a list that describes one of more entitlements.
*/
suspend fun describeEntitlements(block: DescribeEntitlementsRequest.Builder.() -> Unit) = describeEntitlements(DescribeEntitlementsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all fleets in the account are described.
*/
suspend fun describeFleets(input: DescribeFleetsRequest = DescribeFleetsRequest {}): DescribeFleetsResponse
/**
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all fleets in the account are described.
*/
suspend fun describeFleets(block: DescribeFleetsRequest.Builder.() -> Unit) = describeFleets(DescribeFleetsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided. Otherwise, all image builders in the account are described.
*/
suspend fun describeImageBuilders(input: DescribeImageBuildersRequest = DescribeImageBuildersRequest {}): DescribeImageBuildersResponse
/**
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided. Otherwise, all image builders in the account are described.
*/
suspend fun describeImageBuilders(block: DescribeImageBuildersRequest.Builder.() -> Unit) = describeImageBuilders(DescribeImageBuildersRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*/
suspend fun describeImagePermissions(input: DescribeImagePermissionsRequest): DescribeImagePermissionsResponse
/**
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*/
suspend fun describeImagePermissions(block: DescribeImagePermissionsRequest.Builder.() -> Unit) = describeImagePermissions(DescribeImagePermissionsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided. Otherwise, all images in the account are described.
*/
suspend fun describeImages(input: DescribeImagesRequest = DescribeImagesRequest {}): DescribeImagesResponse
/**
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided. Otherwise, all images in the account are described.
*/
suspend fun describeImages(block: DescribeImagesRequest.Builder.() -> Unit) = describeImages(DescribeImagesRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not provided, the default is to authenticate users using a streaming URL.
*/
suspend fun describeSessions(input: DescribeSessionsRequest): DescribeSessionsResponse
/**
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not provided, the default is to authenticate users using a streaming URL.
*/
suspend fun describeSessions(block: DescribeSessionsRequest.Builder.() -> Unit) = describeSessions(DescribeSessionsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all stacks in the account are described.
*/
suspend fun describeStacks(input: DescribeStacksRequest = DescribeStacksRequest {}): DescribeStacksResponse
/**
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all stacks in the account are described.
*/
suspend fun describeStacks(block: DescribeStacksRequest.Builder.() -> Unit) = describeStacks(DescribeStacksRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more usage report subscriptions.
*/
suspend fun describeUsageReportSubscriptions(input: DescribeUsageReportSubscriptionsRequest = DescribeUsageReportSubscriptionsRequest {}): DescribeUsageReportSubscriptionsResponse
/**
* Retrieves a list that describes one or more usage report subscriptions.
*/
suspend fun describeUsageReportSubscriptions(block: DescribeUsageReportSubscriptionsRequest.Builder.() -> Unit) = describeUsageReportSubscriptions(DescribeUsageReportSubscriptionsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the following:
* + The stack name
* + The user name (email address of the user associated with the stack) and the authentication type for the user
*/
suspend fun describeUserStackAssociations(input: DescribeUserStackAssociationsRequest = DescribeUserStackAssociationsRequest {}): DescribeUserStackAssociationsResponse
/**
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the following:
* + The stack name
* + The user name (email address of the user associated with the stack) and the authentication type for the user
*/
suspend fun describeUserStackAssociations(block: DescribeUserStackAssociationsRequest.Builder.() -> Unit) = describeUserStackAssociations(DescribeUserStackAssociationsRequest.Builder().apply(block).build())
/**
* Retrieves a list that describes one or more specified users in the user pool.
*/
suspend fun describeUsers(input: DescribeUsersRequest): DescribeUsersResponse
/**
* Retrieves a list that describes one or more specified users in the user pool.
*/
suspend fun describeUsers(block: DescribeUsersRequest.Builder.() -> Unit) = describeUsers(DescribeUsersRequest.Builder().apply(block).build())
/**
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled. This action does not delete the user.
*/
suspend fun disableUser(input: DisableUserRequest): DisableUserResponse
/**
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled. This action does not delete the user.
*/
suspend fun disableUser(block: DisableUserRequest.Builder.() -> Unit) = disableUser(DisableUserRequest.Builder().apply(block).build())
/**
* Disassociates the specified application from the fleet.
*/
suspend fun disassociateApplicationFleet(input: DisassociateApplicationFleetRequest): DisassociateApplicationFleetResponse
/**
* Disassociates the specified application from the fleet.
*/
suspend fun disassociateApplicationFleet(block: DisassociateApplicationFleetRequest.Builder.() -> Unit) = disassociateApplicationFleet(DisassociateApplicationFleetRequest.Builder().apply(block).build())
/**
* Deletes the specified application from the specified entitlement.
*/
suspend fun disassociateApplicationFromEntitlement(input: DisassociateApplicationFromEntitlementRequest): DisassociateApplicationFromEntitlementResponse
/**
* Deletes the specified application from the specified entitlement.
*/
suspend fun disassociateApplicationFromEntitlement(block: DisassociateApplicationFromEntitlementRequest.Builder.() -> Unit) = disassociateApplicationFromEntitlement(DisassociateApplicationFromEntitlementRequest.Builder().apply(block).build())
/**
* Disassociates the specified fleet from the specified stack.
*/
suspend fun disassociateFleet(input: DisassociateFleetRequest): DisassociateFleetResponse
/**
* Disassociates the specified fleet from the specified stack.
*/
suspend fun disassociateFleet(block: DisassociateFleetRequest.Builder.() -> Unit) = disassociateFleet(DisassociateFleetRequest.Builder().apply(block).build())
/**
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications from the stacks to which they are assigned.
*/
suspend fun enableUser(input: EnableUserRequest): EnableUserResponse
/**
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications from the stacks to which they are assigned.
*/
suspend fun enableUser(block: EnableUserRequest.Builder.() -> Unit) = enableUser(EnableUserRequest.Builder().apply(block).build())
/**
* Immediately stops the specified streaming session.
*/
suspend fun expireSession(input: ExpireSessionRequest): ExpireSessionResponse
/**
* Immediately stops the specified streaming session.
*/
suspend fun expireSession(block: ExpireSessionRequest.Builder.() -> Unit) = expireSession(ExpireSessionRequest.Builder().apply(block).build())
/**
* Retrieves the name of the fleet that is associated with the specified stack.
*/
suspend fun listAssociatedFleets(input: ListAssociatedFleetsRequest): ListAssociatedFleetsResponse
/**
* Retrieves the name of the fleet that is associated with the specified stack.
*/
suspend fun listAssociatedFleets(block: ListAssociatedFleetsRequest.Builder.() -> Unit) = listAssociatedFleets(ListAssociatedFleetsRequest.Builder().apply(block).build())
/**
* Retrieves the name of the stack with which the specified fleet is associated.
*/
suspend fun listAssociatedStacks(input: ListAssociatedStacksRequest): ListAssociatedStacksResponse
/**
* Retrieves the name of the stack with which the specified fleet is associated.
*/
suspend fun listAssociatedStacks(block: ListAssociatedStacksRequest.Builder.() -> Unit) = listAssociatedStacks(ListAssociatedStacksRequest.Builder().apply(block).build())
/**
* Retrieves a list of entitled applications.
*/
suspend fun listEntitledApplications(input: ListEntitledApplicationsRequest): ListEntitledApplicationsResponse
/**
* Retrieves a list of entitled applications.
*/
suspend fun listEntitledApplications(block: ListEntitledApplicationsRequest.Builder.() -> Unit) = listEntitledApplications(ListEntitledApplicationsRequest.Builder().apply(block).build())
/**
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders, images, fleets, and stacks.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse
/**
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders, images, fleets, and stacks.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun listTagsForResource(block: ListTagsForResourceRequest.Builder.() -> Unit) = listTagsForResource(ListTagsForResourceRequest.Builder().apply(block).build())
/**
* Starts the specified fleet.
*/
suspend fun startFleet(input: StartFleetRequest): StartFleetResponse
/**
* Starts the specified fleet.
*/
suspend fun startFleet(block: StartFleetRequest.Builder.() -> Unit) = startFleet(StartFleetRequest.Builder().apply(block).build())
/**
* Starts the specified image builder.
*/
suspend fun startImageBuilder(input: StartImageBuilderRequest): StartImageBuilderResponse
/**
* Starts the specified image builder.
*/
suspend fun startImageBuilder(block: StartImageBuilderRequest.Builder.() -> Unit) = startImageBuilder(StartImageBuilderRequest.Builder().apply(block).build())
/**
* Stops the specified fleet.
*/
suspend fun stopFleet(input: StopFleetRequest): StopFleetResponse
/**
* Stops the specified fleet.
*/
suspend fun stopFleet(block: StopFleetRequest.Builder.() -> Unit) = stopFleet(StopFleetRequest.Builder().apply(block).build())
/**
* Stops the specified image builder.
*/
suspend fun stopImageBuilder(input: StopImageBuilderRequest): StopImageBuilderResponse
/**
* Stops the specified image builder.
*/
suspend fun stopImageBuilder(block: StopImageBuilderRequest.Builder.() -> Unit) = stopImageBuilder(StopImageBuilderRequest.Builder().apply(block).build())
/**
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders, images, fleets, and stacks.
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this operation updates its value.
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your resources, use UntagResource.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun tagResource(input: TagResourceRequest): TagResourceResponse
/**
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders, images, fleets, and stacks.
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this operation updates its value.
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your resources, use UntagResource.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun tagResource(block: TagResourceRequest.Builder.() -> Unit) = tagResource(TagResourceRequest.Builder().apply(block).build())
/**
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
* To list the current tags for your resources, use ListTagsForResource.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse
/**
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
* To list the current tags for your resources, use ListTagsForResource.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
suspend fun untagResource(block: UntagResourceRequest.Builder.() -> Unit) = untagResource(UntagResourceRequest.Builder().apply(block).build())
/**
* Updates the specified application.
*/
suspend fun updateApplication(input: UpdateApplicationRequest): UpdateApplicationResponse
/**
* Updates the specified application.
*/
suspend fun updateApplication(block: UpdateApplicationRequest.Builder.() -> Unit) = updateApplication(UpdateApplicationRequest.Builder().apply(block).build())
/**
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*/
suspend fun updateDirectoryConfig(input: UpdateDirectoryConfigRequest): UpdateDirectoryConfigResponse
/**
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration information required to join fleets and image builders to Microsoft Active Directory domains.
*/
suspend fun updateDirectoryConfig(block: UpdateDirectoryConfigRequest.Builder.() -> Unit) = updateDirectoryConfig(UpdateDirectoryConfigRequest.Builder().apply(block).build())
/**
* Updates the specified entitlement.
*/
suspend fun updateEntitlement(input: UpdateEntitlementRequest): UpdateEntitlementResponse
/**
* Updates the specified entitlement.
*/
suspend fun updateEntitlement(block: UpdateEntitlementRequest.Builder.() -> Unit) = updateEntitlement(UpdateEntitlementRequest.Builder().apply(block).build())
/**
* Updates the specified fleet.
*
* If the fleet is in the `STOPPED` state, you can update any attribute except the fleet name.
*
* If the fleet is in the `RUNNING` state, you can update the following based on the fleet type:
* + Always-On and On-Demand fleet typesYou can update the `DisplayName`, `ComputeCapacity`, `ImageARN`, `ImageName`, `IdleDisconnectTimeoutInSeconds`, and `DisconnectTimeoutInSeconds` attributes.
* + Elastic fleet typeYou can update the `DisplayName`, `IdleDisconnectTimeoutInSeconds`, `DisconnectTimeoutInSeconds`, `MaxConcurrentSessions`, and `UsbDeviceFilterStrings` attributes.
*
* If the fleet is in the `STARTING` or `STOPPED` state, you can't update it.
*/
suspend fun updateFleet(input: UpdateFleetRequest = UpdateFleetRequest {}): UpdateFleetResponse
/**
* Updates the specified fleet.
*
* If the fleet is in the `STOPPED` state, you can update any attribute except the fleet name.
*
* If the fleet is in the `RUNNING` state, you can update the following based on the fleet type:
* + Always-On and On-Demand fleet typesYou can update the `DisplayName`, `ComputeCapacity`, `ImageARN`, `ImageName`, `IdleDisconnectTimeoutInSeconds`, and `DisconnectTimeoutInSeconds` attributes.
* + Elastic fleet typeYou can update the `DisplayName`, `IdleDisconnectTimeoutInSeconds`, `DisconnectTimeoutInSeconds`, `MaxConcurrentSessions`, and `UsbDeviceFilterStrings` attributes.
*
* If the fleet is in the `STARTING` or `STOPPED` state, you can't update it.
*/
suspend fun updateFleet(block: UpdateFleetRequest.Builder.() -> Unit) = updateFleet(UpdateFleetRequest.Builder().apply(block).build())
/**
* Adds or updates permissions for the specified private image.
*/
suspend fun updateImagePermissions(input: UpdateImagePermissionsRequest): UpdateImagePermissionsResponse
/**
* Adds or updates permissions for the specified private image.
*/
suspend fun updateImagePermissions(block: UpdateImagePermissionsRequest.Builder.() -> Unit) = updateImagePermissions(UpdateImagePermissionsRequest.Builder().apply(block).build())
/**
* Updates the specified fields for the specified stack.
*/
suspend fun updateStack(input: UpdateStackRequest): UpdateStackResponse
/**
* Updates the specified fields for the specified stack.
*/
suspend fun updateStack(block: UpdateStackRequest.Builder.() -> Unit) = updateStack(UpdateStackRequest.Builder().apply(block).build())
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy