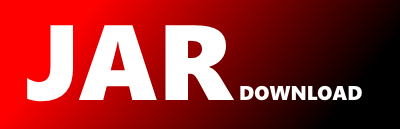
commonMain.aws.sdk.kotlin.services.appstream.model.Session.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream-jvm Show documentation
Show all versions of appstream-jvm Show documentation
The AWS SDK for Kotlin client for AppStream
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Describes a streaming session.
*/
class Session private constructor(builder: Builder) {
/**
* The authentication method. The user is authenticated using a streaming URL (`API`) or SAML 2.0 federation (`SAML`).
*/
val authenticationType: aws.sdk.kotlin.services.appstream.model.AuthenticationType? = builder.authenticationType
/**
* Specifies whether a user is connected to the streaming session.
*/
val connectionState: aws.sdk.kotlin.services.appstream.model.SessionConnectionState? = builder.connectionState
/**
* The name of the fleet for the streaming session.
*/
val fleetName: kotlin.String? = builder.fleetName
/**
* The identifier of the streaming session.
*/
val id: kotlin.String? = builder.id
/**
* The time when the streaming session is set to expire. This time is based on the `MaxUserDurationinSeconds` value, which determines the maximum length of time that a streaming session can run. A streaming session might end earlier than the time specified in `SessionMaxExpirationTime`, when the `DisconnectTimeOutInSeconds` elapses or the user chooses to end his or her session. If the `DisconnectTimeOutInSeconds` elapses, or the user chooses to end his or her session, the streaming instance is terminated and the streaming session ends.
*/
val maxExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.maxExpirationTime
/**
* The network details for the streaming session.
*/
val networkAccessConfiguration: aws.sdk.kotlin.services.appstream.model.NetworkAccessConfiguration? = builder.networkAccessConfiguration
/**
* The name of the stack for the streaming session.
*/
val stackName: kotlin.String? = builder.stackName
/**
* The time when a streaming instance is dedicated for the user.
*/
val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The current state of the streaming session.
*/
val state: aws.sdk.kotlin.services.appstream.model.SessionState? = builder.state
/**
* The identifier of the user for whom the session was created.
*/
val userId: kotlin.String? = builder.userId
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appstream.model.Session = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Session(")
append("authenticationType=$authenticationType,")
append("connectionState=$connectionState,")
append("fleetName=$fleetName,")
append("id=$id,")
append("maxExpirationTime=$maxExpirationTime,")
append("networkAccessConfiguration=$networkAccessConfiguration,")
append("stackName=$stackName,")
append("startTime=$startTime,")
append("state=$state,")
append("userId=$userId)")
}
override fun hashCode(): kotlin.Int {
var result = authenticationType?.hashCode() ?: 0
result = 31 * result + (connectionState?.hashCode() ?: 0)
result = 31 * result + (fleetName?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (maxExpirationTime?.hashCode() ?: 0)
result = 31 * result + (networkAccessConfiguration?.hashCode() ?: 0)
result = 31 * result + (stackName?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (userId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Session
if (authenticationType != other.authenticationType) return false
if (connectionState != other.connectionState) return false
if (fleetName != other.fleetName) return false
if (id != other.id) return false
if (maxExpirationTime != other.maxExpirationTime) return false
if (networkAccessConfiguration != other.networkAccessConfiguration) return false
if (stackName != other.stackName) return false
if (startTime != other.startTime) return false
if (state != other.state) return false
if (userId != other.userId) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appstream.model.Session = Builder(this).apply(block).build()
class Builder {
/**
* The authentication method. The user is authenticated using a streaming URL (`API`) or SAML 2.0 federation (`SAML`).
*/
var authenticationType: aws.sdk.kotlin.services.appstream.model.AuthenticationType? = null
/**
* Specifies whether a user is connected to the streaming session.
*/
var connectionState: aws.sdk.kotlin.services.appstream.model.SessionConnectionState? = null
/**
* The name of the fleet for the streaming session.
*/
var fleetName: kotlin.String? = null
/**
* The identifier of the streaming session.
*/
var id: kotlin.String? = null
/**
* The time when the streaming session is set to expire. This time is based on the `MaxUserDurationinSeconds` value, which determines the maximum length of time that a streaming session can run. A streaming session might end earlier than the time specified in `SessionMaxExpirationTime`, when the `DisconnectTimeOutInSeconds` elapses or the user chooses to end his or her session. If the `DisconnectTimeOutInSeconds` elapses, or the user chooses to end his or her session, the streaming instance is terminated and the streaming session ends.
*/
var maxExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The network details for the streaming session.
*/
var networkAccessConfiguration: aws.sdk.kotlin.services.appstream.model.NetworkAccessConfiguration? = null
/**
* The name of the stack for the streaming session.
*/
var stackName: kotlin.String? = null
/**
* The time when a streaming instance is dedicated for the user.
*/
var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The current state of the streaming session.
*/
var state: aws.sdk.kotlin.services.appstream.model.SessionState? = null
/**
* The identifier of the user for whom the session was created.
*/
var userId: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appstream.model.Session) : this() {
this.authenticationType = x.authenticationType
this.connectionState = x.connectionState
this.fleetName = x.fleetName
this.id = x.id
this.maxExpirationTime = x.maxExpirationTime
this.networkAccessConfiguration = x.networkAccessConfiguration
this.stackName = x.stackName
this.startTime = x.startTime
this.state = x.state
this.userId = x.userId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appstream.model.Session = Session(this)
/**
* construct an [aws.sdk.kotlin.services.appstream.model.NetworkAccessConfiguration] inside the given [block]
*/
fun networkAccessConfiguration(block: aws.sdk.kotlin.services.appstream.model.NetworkAccessConfiguration.Builder.() -> kotlin.Unit) {
this.networkAccessConfiguration = aws.sdk.kotlin.services.appstream.model.NetworkAccessConfiguration.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy