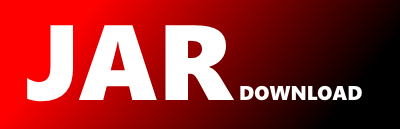
commonMain.aws.sdk.kotlin.services.appstream.model.CreateUpdatedImageRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream-jvm Show documentation
Show all versions of appstream-jvm Show documentation
The AWS SDK for Kotlin client for AppStream
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream.model
public class CreateUpdatedImageRequest private constructor(builder: Builder) {
/**
* Indicates whether to display the status of image update availability before AppStream 2.0 initiates the process of creating a new updated image. If this value is set to `true`, AppStream 2.0 displays whether image updates are available. If this value is set to `false`, AppStream 2.0 initiates the process of creating a new updated image without displaying whether image updates are available.
*/
public val dryRun: kotlin.Boolean = builder.dryRun
/**
* The name of the image to update.
*/
public val existingImageName: kotlin.String? = builder.existingImageName
/**
* The description to display for the new image.
*/
public val newImageDescription: kotlin.String? = builder.newImageDescription
/**
* The name to display for the new image.
*/
public val newImageDisplayName: kotlin.String? = builder.newImageDisplayName
/**
* The name of the new image. The name must be unique within the AWS account and Region.
*/
public val newImageName: kotlin.String? = builder.newImageName
/**
* The tags to associate with the new image. A tag is a key-value pair, and the value is optional. For example, Environment=Test. If you do not specify a value, Environment=.
*
* Generally allowed characters are: letters, numbers, and spaces representable in UTF-8, and the following special characters:
*
* _ . : / = + \ - @
*
* If you do not specify a value, the value is set to an empty string.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
public val newImageTags: Map? = builder.newImageTags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appstream.model.CreateUpdatedImageRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateUpdatedImageRequest(")
append("dryRun=$dryRun,")
append("existingImageName=$existingImageName,")
append("newImageDescription=$newImageDescription,")
append("newImageDisplayName=$newImageDisplayName,")
append("newImageName=$newImageName,")
append("newImageTags=$newImageTags)")
}
override fun hashCode(): kotlin.Int {
var result = dryRun.hashCode()
result = 31 * result + (existingImageName?.hashCode() ?: 0)
result = 31 * result + (newImageDescription?.hashCode() ?: 0)
result = 31 * result + (newImageDisplayName?.hashCode() ?: 0)
result = 31 * result + (newImageName?.hashCode() ?: 0)
result = 31 * result + (newImageTags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateUpdatedImageRequest
if (dryRun != other.dryRun) return false
if (existingImageName != other.existingImageName) return false
if (newImageDescription != other.newImageDescription) return false
if (newImageDisplayName != other.newImageDisplayName) return false
if (newImageName != other.newImageName) return false
if (newImageTags != other.newImageTags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appstream.model.CreateUpdatedImageRequest = Builder(this).apply(block).build()
public class Builder {
/**
* Indicates whether to display the status of image update availability before AppStream 2.0 initiates the process of creating a new updated image. If this value is set to `true`, AppStream 2.0 displays whether image updates are available. If this value is set to `false`, AppStream 2.0 initiates the process of creating a new updated image without displaying whether image updates are available.
*/
public var dryRun: kotlin.Boolean = false
/**
* The name of the image to update.
*/
public var existingImageName: kotlin.String? = null
/**
* The description to display for the new image.
*/
public var newImageDescription: kotlin.String? = null
/**
* The name to display for the new image.
*/
public var newImageDisplayName: kotlin.String? = null
/**
* The name of the new image. The name must be unique within the AWS account and Region.
*/
public var newImageName: kotlin.String? = null
/**
* The tags to associate with the new image. A tag is a key-value pair, and the value is optional. For example, Environment=Test. If you do not specify a value, Environment=.
*
* Generally allowed characters are: letters, numbers, and spaces representable in UTF-8, and the following special characters:
*
* _ . : / = + \ - @
*
* If you do not specify a value, the value is set to an empty string.
*
* For more information about tags, see [Tagging Your Resources](https://docs.aws.amazon.com/appstream2/latest/developerguide/tagging-basic.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
public var newImageTags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appstream.model.CreateUpdatedImageRequest) : this() {
this.dryRun = x.dryRun
this.existingImageName = x.existingImageName
this.newImageDescription = x.newImageDescription
this.newImageDisplayName = x.newImageDisplayName
this.newImageName = x.newImageName
this.newImageTags = x.newImageTags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appstream.model.CreateUpdatedImageRequest = CreateUpdatedImageRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy