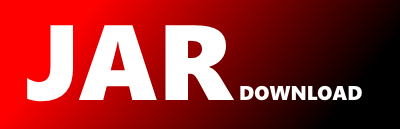
commonMain.aws.sdk.kotlin.services.appstream.model.AppBlock.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream-jvm Show documentation
Show all versions of appstream-jvm Show documentation
The AWS SDK for Kotlin client for AppStream
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Describes an app block.
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3 bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard disk includes the application binaries and other files necessary to launch your applications. Multiple applications can be assigned to a single app block.
*
* This is only supported for Elastic fleets.
*/
public class AppBlock private constructor(builder: Builder) {
/**
* The errors of the app block.
*/
public val appBlockErrors: List? = builder.appBlockErrors
/**
* The ARN of the app block.
*/
public val arn: kotlin.String? = builder.arn
/**
* The created time of the app block.
*/
public val createdTime: aws.smithy.kotlin.runtime.time.Instant? = builder.createdTime
/**
* The description of the app block.
*/
public val description: kotlin.String? = builder.description
/**
* The display name of the app block.
*/
public val displayName: kotlin.String? = builder.displayName
/**
* The name of the app block.
*/
public val name: kotlin.String? = builder.name
/**
* The packaging type of the app block.
*/
public val packagingType: aws.sdk.kotlin.services.appstream.model.PackagingType? = builder.packagingType
/**
* The post setup script details of the app block.
*
* This only applies to app blocks with PackagingType `APPSTREAM2`.
*/
public val postSetupScriptDetails: aws.sdk.kotlin.services.appstream.model.ScriptDetails? = builder.postSetupScriptDetails
/**
* The setup script details of the app block.
*
* This only applies to app blocks with PackagingType `CUSTOM`.
*/
public val setupScriptDetails: aws.sdk.kotlin.services.appstream.model.ScriptDetails? = builder.setupScriptDetails
/**
* The source S3 location of the app block.
*/
public val sourceS3Location: aws.sdk.kotlin.services.appstream.model.S3Location? = builder.sourceS3Location
/**
* The state of the app block.
*
* An app block with AppStream 2.0 packaging will be in the `INACTIVE` state if no application package (VHD) is assigned to it. After an application package (VHD) is created by an app block builder for an app block, it becomes `ACTIVE`.
*
* Custom app blocks are always in the `ACTIVE` state and no action is required to use them.
*/
public val state: aws.sdk.kotlin.services.appstream.model.AppBlockState? = builder.state
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appstream.model.AppBlock = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AppBlock(")
append("appBlockErrors=$appBlockErrors,")
append("arn=$arn,")
append("createdTime=$createdTime,")
append("description=$description,")
append("displayName=$displayName,")
append("name=$name,")
append("packagingType=$packagingType,")
append("postSetupScriptDetails=$postSetupScriptDetails,")
append("setupScriptDetails=$setupScriptDetails,")
append("sourceS3Location=$sourceS3Location,")
append("state=$state")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = appBlockErrors?.hashCode() ?: 0
result = 31 * result + (arn?.hashCode() ?: 0)
result = 31 * result + (createdTime?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (displayName?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (packagingType?.hashCode() ?: 0)
result = 31 * result + (postSetupScriptDetails?.hashCode() ?: 0)
result = 31 * result + (setupScriptDetails?.hashCode() ?: 0)
result = 31 * result + (sourceS3Location?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AppBlock
if (appBlockErrors != other.appBlockErrors) return false
if (arn != other.arn) return false
if (createdTime != other.createdTime) return false
if (description != other.description) return false
if (displayName != other.displayName) return false
if (name != other.name) return false
if (packagingType != other.packagingType) return false
if (postSetupScriptDetails != other.postSetupScriptDetails) return false
if (setupScriptDetails != other.setupScriptDetails) return false
if (sourceS3Location != other.sourceS3Location) return false
if (state != other.state) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appstream.model.AppBlock = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The errors of the app block.
*/
public var appBlockErrors: List? = null
/**
* The ARN of the app block.
*/
public var arn: kotlin.String? = null
/**
* The created time of the app block.
*/
public var createdTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The description of the app block.
*/
public var description: kotlin.String? = null
/**
* The display name of the app block.
*/
public var displayName: kotlin.String? = null
/**
* The name of the app block.
*/
public var name: kotlin.String? = null
/**
* The packaging type of the app block.
*/
public var packagingType: aws.sdk.kotlin.services.appstream.model.PackagingType? = null
/**
* The post setup script details of the app block.
*
* This only applies to app blocks with PackagingType `APPSTREAM2`.
*/
public var postSetupScriptDetails: aws.sdk.kotlin.services.appstream.model.ScriptDetails? = null
/**
* The setup script details of the app block.
*
* This only applies to app blocks with PackagingType `CUSTOM`.
*/
public var setupScriptDetails: aws.sdk.kotlin.services.appstream.model.ScriptDetails? = null
/**
* The source S3 location of the app block.
*/
public var sourceS3Location: aws.sdk.kotlin.services.appstream.model.S3Location? = null
/**
* The state of the app block.
*
* An app block with AppStream 2.0 packaging will be in the `INACTIVE` state if no application package (VHD) is assigned to it. After an application package (VHD) is created by an app block builder for an app block, it becomes `ACTIVE`.
*
* Custom app blocks are always in the `ACTIVE` state and no action is required to use them.
*/
public var state: aws.sdk.kotlin.services.appstream.model.AppBlockState? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appstream.model.AppBlock) : this() {
this.appBlockErrors = x.appBlockErrors
this.arn = x.arn
this.createdTime = x.createdTime
this.description = x.description
this.displayName = x.displayName
this.name = x.name
this.packagingType = x.packagingType
this.postSetupScriptDetails = x.postSetupScriptDetails
this.setupScriptDetails = x.setupScriptDetails
this.sourceS3Location = x.sourceS3Location
this.state = x.state
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appstream.model.AppBlock = AppBlock(this)
/**
* construct an [aws.sdk.kotlin.services.appstream.model.ScriptDetails] inside the given [block]
*/
public fun postSetupScriptDetails(block: aws.sdk.kotlin.services.appstream.model.ScriptDetails.Builder.() -> kotlin.Unit) {
this.postSetupScriptDetails = aws.sdk.kotlin.services.appstream.model.ScriptDetails.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.appstream.model.ScriptDetails] inside the given [block]
*/
public fun setupScriptDetails(block: aws.sdk.kotlin.services.appstream.model.ScriptDetails.Builder.() -> kotlin.Unit) {
this.setupScriptDetails = aws.sdk.kotlin.services.appstream.model.ScriptDetails.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.appstream.model.S3Location] inside the given [block]
*/
public fun sourceS3Location(block: aws.sdk.kotlin.services.appstream.model.S3Location.Builder.() -> kotlin.Unit) {
this.sourceS3Location = aws.sdk.kotlin.services.appstream.model.S3Location.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy