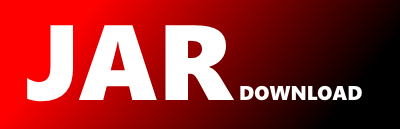
commonMain.aws.sdk.kotlin.services.appstream.model.Application.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream-jvm Show documentation
Show all versions of appstream-jvm Show documentation
The AWS SDK for Kotlin client for AppStream
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Describes an application in the application catalog.
*/
public class Application private constructor(builder: Builder) {
/**
* The app block ARN of the application.
*/
public val appBlockArn: kotlin.String? = builder.appBlockArn
/**
* The ARN of the application.
*/
public val arn: kotlin.String? = builder.arn
/**
* The time at which the application was created within the app block.
*/
public val createdTime: aws.smithy.kotlin.runtime.time.Instant? = builder.createdTime
/**
* The description of the application.
*/
public val description: kotlin.String? = builder.description
/**
* The application name to display.
*/
public val displayName: kotlin.String? = builder.displayName
/**
* If there is a problem, the application can be disabled after image creation.
*/
public val enabled: kotlin.Boolean? = builder.enabled
/**
* The S3 location of the application icon.
*/
public val iconS3Location: aws.sdk.kotlin.services.appstream.model.S3Location? = builder.iconS3Location
/**
* The URL for the application icon. This URL might be time-limited.
*/
public val iconUrl: kotlin.String? = builder.iconUrl
/**
* The instance families for the application.
*/
public val instanceFamilies: List? = builder.instanceFamilies
/**
* The arguments that are passed to the application at launch.
*/
public val launchParameters: kotlin.String? = builder.launchParameters
/**
* The path to the application executable in the instance.
*/
public val launchPath: kotlin.String? = builder.launchPath
/**
* Additional attributes that describe the application.
*/
public val metadata: Map? = builder.metadata
/**
* The name of the application.
*/
public val name: kotlin.String? = builder.name
/**
* The platforms on which the application can run.
*/
public val platforms: List? = builder.platforms
/**
* The working directory for the application.
*/
public val workingDirectory: kotlin.String? = builder.workingDirectory
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appstream.model.Application = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Application(")
append("appBlockArn=$appBlockArn,")
append("arn=$arn,")
append("createdTime=$createdTime,")
append("description=$description,")
append("displayName=$displayName,")
append("enabled=$enabled,")
append("iconS3Location=$iconS3Location,")
append("iconUrl=$iconUrl,")
append("instanceFamilies=$instanceFamilies,")
append("launchParameters=$launchParameters,")
append("launchPath=$launchPath,")
append("metadata=$metadata,")
append("name=$name,")
append("platforms=$platforms,")
append("workingDirectory=$workingDirectory")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = appBlockArn?.hashCode() ?: 0
result = 31 * result + (arn?.hashCode() ?: 0)
result = 31 * result + (createdTime?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (displayName?.hashCode() ?: 0)
result = 31 * result + (enabled?.hashCode() ?: 0)
result = 31 * result + (iconS3Location?.hashCode() ?: 0)
result = 31 * result + (iconUrl?.hashCode() ?: 0)
result = 31 * result + (instanceFamilies?.hashCode() ?: 0)
result = 31 * result + (launchParameters?.hashCode() ?: 0)
result = 31 * result + (launchPath?.hashCode() ?: 0)
result = 31 * result + (metadata?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (platforms?.hashCode() ?: 0)
result = 31 * result + (workingDirectory?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Application
if (appBlockArn != other.appBlockArn) return false
if (arn != other.arn) return false
if (createdTime != other.createdTime) return false
if (description != other.description) return false
if (displayName != other.displayName) return false
if (enabled != other.enabled) return false
if (iconS3Location != other.iconS3Location) return false
if (iconUrl != other.iconUrl) return false
if (instanceFamilies != other.instanceFamilies) return false
if (launchParameters != other.launchParameters) return false
if (launchPath != other.launchPath) return false
if (metadata != other.metadata) return false
if (name != other.name) return false
if (platforms != other.platforms) return false
if (workingDirectory != other.workingDirectory) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appstream.model.Application = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The app block ARN of the application.
*/
public var appBlockArn: kotlin.String? = null
/**
* The ARN of the application.
*/
public var arn: kotlin.String? = null
/**
* The time at which the application was created within the app block.
*/
public var createdTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The description of the application.
*/
public var description: kotlin.String? = null
/**
* The application name to display.
*/
public var displayName: kotlin.String? = null
/**
* If there is a problem, the application can be disabled after image creation.
*/
public var enabled: kotlin.Boolean? = null
/**
* The S3 location of the application icon.
*/
public var iconS3Location: aws.sdk.kotlin.services.appstream.model.S3Location? = null
/**
* The URL for the application icon. This URL might be time-limited.
*/
public var iconUrl: kotlin.String? = null
/**
* The instance families for the application.
*/
public var instanceFamilies: List? = null
/**
* The arguments that are passed to the application at launch.
*/
public var launchParameters: kotlin.String? = null
/**
* The path to the application executable in the instance.
*/
public var launchPath: kotlin.String? = null
/**
* Additional attributes that describe the application.
*/
public var metadata: Map? = null
/**
* The name of the application.
*/
public var name: kotlin.String? = null
/**
* The platforms on which the application can run.
*/
public var platforms: List? = null
/**
* The working directory for the application.
*/
public var workingDirectory: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appstream.model.Application) : this() {
this.appBlockArn = x.appBlockArn
this.arn = x.arn
this.createdTime = x.createdTime
this.description = x.description
this.displayName = x.displayName
this.enabled = x.enabled
this.iconS3Location = x.iconS3Location
this.iconUrl = x.iconUrl
this.instanceFamilies = x.instanceFamilies
this.launchParameters = x.launchParameters
this.launchPath = x.launchPath
this.metadata = x.metadata
this.name = x.name
this.platforms = x.platforms
this.workingDirectory = x.workingDirectory
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appstream.model.Application = Application(this)
/**
* construct an [aws.sdk.kotlin.services.appstream.model.S3Location] inside the given [block]
*/
public fun iconS3Location(block: aws.sdk.kotlin.services.appstream.model.S3Location.Builder.() -> kotlin.Unit) {
this.iconS3Location = aws.sdk.kotlin.services.appstream.model.S3Location.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy