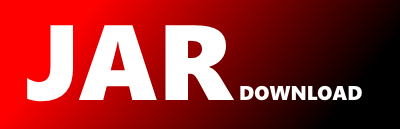
commonMain.aws.sdk.kotlin.services.appstream.model.UpdateAppBlockBuilderRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream-jvm Show documentation
Show all versions of appstream-jvm Show documentation
The AWS SDK for Kotlin client for AppStream
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.appstream.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateAppBlockBuilderRequest private constructor(builder: Builder) {
/**
* The list of interface VPC endpoint (interface endpoint) objects. Administrators can connect to the app block builder only through the specified endpoints.
*/
public val accessEndpoints: List? = builder.accessEndpoints
/**
* The attributes to delete from the app block builder.
*/
public val attributesToDelete: List? = builder.attributesToDelete
/**
* The description of the app block builder.
*/
public val description: kotlin.String? = builder.description
/**
* The display name of the app block builder.
*/
public val displayName: kotlin.String? = builder.displayName
/**
* Enables or disables default internet access for the app block builder.
*/
public val enableDefaultInternetAccess: kotlin.Boolean? = builder.enableDefaultInternetAccess
/**
* The Amazon Resource Name (ARN) of the IAM role to apply to the app block builder. To assume a role, the app block builder calls the AWS Security Token Service (STS) `AssumeRole` API operation and passes the ARN of the role to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and creates the **appstream_machine_role** credential profile on the instance.
*
* For more information, see [Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming Instances](https://docs.aws.amazon.com/appstream2/latest/developerguide/using-iam-roles-to-grant-permissions-to-applications-scripts-streaming-instances.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
public val iamRoleArn: kotlin.String? = builder.iamRoleArn
/**
* The instance type to use when launching the app block builder. The following instance types are available:
* + stream.standard.small
* + stream.standard.medium
* + stream.standard.large
* + stream.standard.xlarge
* + stream.standard.2xlarge
*/
public val instanceType: kotlin.String? = builder.instanceType
/**
* The unique name for the app block builder.
*/
public val name: kotlin.String? = builder.name
/**
* The platform of the app block builder.
*
* `WINDOWS_SERVER_2019` is the only valid value.
*/
public val platform: aws.sdk.kotlin.services.appstream.model.PlatformType? = builder.platform
/**
* The VPC configuration for the app block builder.
*
* App block builders require that you specify at least two subnets in different availability zones.
*/
public val vpcConfig: aws.sdk.kotlin.services.appstream.model.VpcConfig? = builder.vpcConfig
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.appstream.model.UpdateAppBlockBuilderRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateAppBlockBuilderRequest(")
append("accessEndpoints=$accessEndpoints,")
append("attributesToDelete=$attributesToDelete,")
append("description=$description,")
append("displayName=$displayName,")
append("enableDefaultInternetAccess=$enableDefaultInternetAccess,")
append("iamRoleArn=$iamRoleArn,")
append("instanceType=$instanceType,")
append("name=$name,")
append("platform=$platform,")
append("vpcConfig=$vpcConfig")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessEndpoints?.hashCode() ?: 0
result = 31 * result + (attributesToDelete?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (displayName?.hashCode() ?: 0)
result = 31 * result + (enableDefaultInternetAccess?.hashCode() ?: 0)
result = 31 * result + (iamRoleArn?.hashCode() ?: 0)
result = 31 * result + (instanceType?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (platform?.hashCode() ?: 0)
result = 31 * result + (vpcConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateAppBlockBuilderRequest
if (accessEndpoints != other.accessEndpoints) return false
if (attributesToDelete != other.attributesToDelete) return false
if (description != other.description) return false
if (displayName != other.displayName) return false
if (enableDefaultInternetAccess != other.enableDefaultInternetAccess) return false
if (iamRoleArn != other.iamRoleArn) return false
if (instanceType != other.instanceType) return false
if (name != other.name) return false
if (platform != other.platform) return false
if (vpcConfig != other.vpcConfig) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.appstream.model.UpdateAppBlockBuilderRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The list of interface VPC endpoint (interface endpoint) objects. Administrators can connect to the app block builder only through the specified endpoints.
*/
public var accessEndpoints: List? = null
/**
* The attributes to delete from the app block builder.
*/
public var attributesToDelete: List? = null
/**
* The description of the app block builder.
*/
public var description: kotlin.String? = null
/**
* The display name of the app block builder.
*/
public var displayName: kotlin.String? = null
/**
* Enables or disables default internet access for the app block builder.
*/
public var enableDefaultInternetAccess: kotlin.Boolean? = null
/**
* The Amazon Resource Name (ARN) of the IAM role to apply to the app block builder. To assume a role, the app block builder calls the AWS Security Token Service (STS) `AssumeRole` API operation and passes the ARN of the role to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and creates the **appstream_machine_role** credential profile on the instance.
*
* For more information, see [Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming Instances](https://docs.aws.amazon.com/appstream2/latest/developerguide/using-iam-roles-to-grant-permissions-to-applications-scripts-streaming-instances.html) in the *Amazon AppStream 2.0 Administration Guide*.
*/
public var iamRoleArn: kotlin.String? = null
/**
* The instance type to use when launching the app block builder. The following instance types are available:
* + stream.standard.small
* + stream.standard.medium
* + stream.standard.large
* + stream.standard.xlarge
* + stream.standard.2xlarge
*/
public var instanceType: kotlin.String? = null
/**
* The unique name for the app block builder.
*/
public var name: kotlin.String? = null
/**
* The platform of the app block builder.
*
* `WINDOWS_SERVER_2019` is the only valid value.
*/
public var platform: aws.sdk.kotlin.services.appstream.model.PlatformType? = null
/**
* The VPC configuration for the app block builder.
*
* App block builders require that you specify at least two subnets in different availability zones.
*/
public var vpcConfig: aws.sdk.kotlin.services.appstream.model.VpcConfig? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.appstream.model.UpdateAppBlockBuilderRequest) : this() {
this.accessEndpoints = x.accessEndpoints
this.attributesToDelete = x.attributesToDelete
this.description = x.description
this.displayName = x.displayName
this.enableDefaultInternetAccess = x.enableDefaultInternetAccess
this.iamRoleArn = x.iamRoleArn
this.instanceType = x.instanceType
this.name = x.name
this.platform = x.platform
this.vpcConfig = x.vpcConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.appstream.model.UpdateAppBlockBuilderRequest = UpdateAppBlockBuilderRequest(this)
/**
* construct an [aws.sdk.kotlin.services.appstream.model.VpcConfig] inside the given [block]
*/
public fun vpcConfig(block: aws.sdk.kotlin.services.appstream.model.VpcConfig.Builder.() -> kotlin.Unit) {
this.vpcConfig = aws.sdk.kotlin.services.appstream.model.VpcConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy