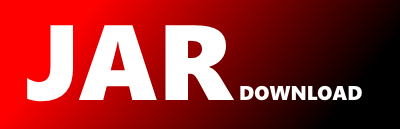
commonMain.aws.sdk.kotlin.services.bedrock.model.GetProvisionedModelThroughputResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrock.model
import aws.smithy.kotlin.runtime.time.Instant
public class GetProvisionedModelThroughputResponse private constructor(builder: Builder) {
/**
* Commitment duration of the provisioned throughput.
*/
public val commitmentDuration: aws.sdk.kotlin.services.bedrock.model.CommitmentDuration? = builder.commitmentDuration
/**
* Commitment expiration time for the provisioned throughput.
*/
public val commitmentExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.commitmentExpirationTime
/**
* The timestamp of the creation time for this provisioned throughput.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.creationTime) { "A non-null value must be provided for creationTime" }
/**
* The ARN of the new model to asssociate with this provisioned throughput.
*/
public val desiredModelArn: kotlin.String = requireNotNull(builder.desiredModelArn) { "A non-null value must be provided for desiredModelArn" }
/**
* The desired number of model units that was requested to be available for this provisioned throughput.
*/
public val desiredModelUnits: kotlin.Int = requireNotNull(builder.desiredModelUnits) { "A non-null value must be provided for desiredModelUnits" }
/**
* Failure message for any issues that the create operation encounters.
*/
public val failureMessage: kotlin.String? = builder.failureMessage
/**
* ARN of the foundation model.
*/
public val foundationModelArn: kotlin.String = requireNotNull(builder.foundationModelArn) { "A non-null value must be provided for foundationModelArn" }
/**
* The timestamp of the last modified time of this provisioned throughput.
*/
public val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastModifiedTime) { "A non-null value must be provided for lastModifiedTime" }
/**
* The ARN or name of the model associated with this provisioned throughput.
*/
public val modelArn: kotlin.String = requireNotNull(builder.modelArn) { "A non-null value must be provided for modelArn" }
/**
* The current number of model units requested to be available for this provisioned throughput.
*/
public val modelUnits: kotlin.Int = requireNotNull(builder.modelUnits) { "A non-null value must be provided for modelUnits" }
/**
* The ARN of the provisioned throughput.
*/
public val provisionedModelArn: kotlin.String = requireNotNull(builder.provisionedModelArn) { "A non-null value must be provided for provisionedModelArn" }
/**
* The name of the provisioned throughput.
*/
public val provisionedModelName: kotlin.String = requireNotNull(builder.provisionedModelName) { "A non-null value must be provided for provisionedModelName" }
/**
* Status of the provisioned throughput.
*/
public val status: aws.sdk.kotlin.services.bedrock.model.ProvisionedModelStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.bedrock.model.GetProvisionedModelThroughputResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetProvisionedModelThroughputResponse(")
append("commitmentDuration=$commitmentDuration,")
append("commitmentExpirationTime=$commitmentExpirationTime,")
append("creationTime=$creationTime,")
append("desiredModelArn=$desiredModelArn,")
append("desiredModelUnits=$desiredModelUnits,")
append("failureMessage=$failureMessage,")
append("foundationModelArn=$foundationModelArn,")
append("lastModifiedTime=$lastModifiedTime,")
append("modelArn=$modelArn,")
append("modelUnits=$modelUnits,")
append("provisionedModelArn=$provisionedModelArn,")
append("provisionedModelName=$provisionedModelName,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = commitmentDuration?.hashCode() ?: 0
result = 31 * result + (commitmentExpirationTime?.hashCode() ?: 0)
result = 31 * result + (creationTime.hashCode())
result = 31 * result + (desiredModelArn.hashCode())
result = 31 * result + (desiredModelUnits)
result = 31 * result + (failureMessage?.hashCode() ?: 0)
result = 31 * result + (foundationModelArn.hashCode())
result = 31 * result + (lastModifiedTime.hashCode())
result = 31 * result + (modelArn.hashCode())
result = 31 * result + (modelUnits)
result = 31 * result + (provisionedModelArn.hashCode())
result = 31 * result + (provisionedModelName.hashCode())
result = 31 * result + (status.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetProvisionedModelThroughputResponse
if (commitmentDuration != other.commitmentDuration) return false
if (commitmentExpirationTime != other.commitmentExpirationTime) return false
if (creationTime != other.creationTime) return false
if (desiredModelArn != other.desiredModelArn) return false
if (desiredModelUnits != other.desiredModelUnits) return false
if (failureMessage != other.failureMessage) return false
if (foundationModelArn != other.foundationModelArn) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (modelArn != other.modelArn) return false
if (modelUnits != other.modelUnits) return false
if (provisionedModelArn != other.provisionedModelArn) return false
if (provisionedModelName != other.provisionedModelName) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.bedrock.model.GetProvisionedModelThroughputResponse = Builder(this).apply(block).build()
public class Builder {
/**
* Commitment duration of the provisioned throughput.
*/
public var commitmentDuration: aws.sdk.kotlin.services.bedrock.model.CommitmentDuration? = null
/**
* Commitment expiration time for the provisioned throughput.
*/
public var commitmentExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The timestamp of the creation time for this provisioned throughput.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of the new model to asssociate with this provisioned throughput.
*/
public var desiredModelArn: kotlin.String? = null
/**
* The desired number of model units that was requested to be available for this provisioned throughput.
*/
public var desiredModelUnits: kotlin.Int? = null
/**
* Failure message for any issues that the create operation encounters.
*/
public var failureMessage: kotlin.String? = null
/**
* ARN of the foundation model.
*/
public var foundationModelArn: kotlin.String? = null
/**
* The timestamp of the last modified time of this provisioned throughput.
*/
public var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN or name of the model associated with this provisioned throughput.
*/
public var modelArn: kotlin.String? = null
/**
* The current number of model units requested to be available for this provisioned throughput.
*/
public var modelUnits: kotlin.Int? = null
/**
* The ARN of the provisioned throughput.
*/
public var provisionedModelArn: kotlin.String? = null
/**
* The name of the provisioned throughput.
*/
public var provisionedModelName: kotlin.String? = null
/**
* Status of the provisioned throughput.
*/
public var status: aws.sdk.kotlin.services.bedrock.model.ProvisionedModelStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.bedrock.model.GetProvisionedModelThroughputResponse) : this() {
this.commitmentDuration = x.commitmentDuration
this.commitmentExpirationTime = x.commitmentExpirationTime
this.creationTime = x.creationTime
this.desiredModelArn = x.desiredModelArn
this.desiredModelUnits = x.desiredModelUnits
this.failureMessage = x.failureMessage
this.foundationModelArn = x.foundationModelArn
this.lastModifiedTime = x.lastModifiedTime
this.modelArn = x.modelArn
this.modelUnits = x.modelUnits
this.provisionedModelArn = x.provisionedModelArn
this.provisionedModelName = x.provisionedModelName
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.bedrock.model.GetProvisionedModelThroughputResponse = GetProvisionedModelThroughputResponse(this)
internal fun correctErrors(): Builder {
if (creationTime == null) creationTime = Instant.fromEpochSeconds(0)
if (desiredModelArn == null) desiredModelArn = ""
if (desiredModelUnits == null) desiredModelUnits = 0
if (foundationModelArn == null) foundationModelArn = ""
if (lastModifiedTime == null) lastModifiedTime = Instant.fromEpochSeconds(0)
if (modelArn == null) modelArn = ""
if (modelUnits == null) modelUnits = 0
if (provisionedModelArn == null) provisionedModelArn = ""
if (provisionedModelName == null) provisionedModelName = ""
if (status == null) status = ProvisionedModelStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy