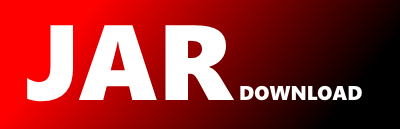
commonMain.aws.sdk.kotlin.services.bedrock.model.CreateModelCustomizationJobRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrock.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateModelCustomizationJobRequest private constructor(builder: Builder) {
/**
* Name of the base model.
*/
public val baseModelIdentifier: kotlin.String? = builder.baseModelIdentifier
/**
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more information, see [Ensuring idempotency](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/Run_Instance_Idempotency.html).
*/
public val clientRequestToken: kotlin.String? = builder.clientRequestToken
/**
* The custom model is encrypted at rest using this key.
*/
public val customModelKmsKeyId: kotlin.String? = builder.customModelKmsKeyId
/**
* A name for the resulting custom model.
*/
public val customModelName: kotlin.String? = builder.customModelName
/**
* Tags to attach to the resulting custom model.
*/
public val customModelTags: List? = builder.customModelTags
/**
* The customization type.
*/
public val customizationType: aws.sdk.kotlin.services.bedrock.model.CustomizationType? = builder.customizationType
/**
* Parameters related to tuning the model. For details on the format for different models, see [Custom model hyperparameters](https://docs.aws.amazon.com/bedrock/latest/userguide/custom-models-hp.html).
*/
public val hyperParameters: Map? = builder.hyperParameters
/**
* A name for the fine-tuning job.
*/
public val jobName: kotlin.String? = builder.jobName
/**
* Tags to attach to the job.
*/
public val jobTags: List? = builder.jobTags
/**
* S3 location for the output data.
*/
public val outputDataConfig: aws.sdk.kotlin.services.bedrock.model.OutputDataConfig? = builder.outputDataConfig
/**
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your behalf. For example, during model training, Amazon Bedrock needs your permission to read input data from an S3 bucket, write model artifacts to an S3 bucket. To pass this role to Amazon Bedrock, the caller of this API must have the `iam:PassRole` permission.
*/
public val roleArn: kotlin.String? = builder.roleArn
/**
* Information about the training dataset.
*/
public val trainingDataConfig: aws.sdk.kotlin.services.bedrock.model.TrainingDataConfig? = builder.trainingDataConfig
/**
* Information about the validation dataset.
*/
public val validationDataConfig: aws.sdk.kotlin.services.bedrock.model.ValidationDataConfig? = builder.validationDataConfig
/**
* The configuration of the Virtual Private Cloud (VPC) that contains the resources that you're using for this job. For more information, see [Protect your model customization jobs using a VPC](https://docs.aws.amazon.com/bedrock/latest/userguide/vpc-model-customization.html).
*/
public val vpcConfig: aws.sdk.kotlin.services.bedrock.model.VpcConfig? = builder.vpcConfig
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.bedrock.model.CreateModelCustomizationJobRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateModelCustomizationJobRequest(")
append("baseModelIdentifier=$baseModelIdentifier,")
append("clientRequestToken=$clientRequestToken,")
append("customModelKmsKeyId=$customModelKmsKeyId,")
append("customModelName=$customModelName,")
append("customModelTags=$customModelTags,")
append("customizationType=$customizationType,")
append("hyperParameters=$hyperParameters,")
append("jobName=$jobName,")
append("jobTags=$jobTags,")
append("outputDataConfig=$outputDataConfig,")
append("roleArn=$roleArn,")
append("trainingDataConfig=$trainingDataConfig,")
append("validationDataConfig=$validationDataConfig,")
append("vpcConfig=$vpcConfig")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = baseModelIdentifier?.hashCode() ?: 0
result = 31 * result + (clientRequestToken?.hashCode() ?: 0)
result = 31 * result + (customModelKmsKeyId?.hashCode() ?: 0)
result = 31 * result + (customModelName?.hashCode() ?: 0)
result = 31 * result + (customModelTags?.hashCode() ?: 0)
result = 31 * result + (customizationType?.hashCode() ?: 0)
result = 31 * result + (hyperParameters?.hashCode() ?: 0)
result = 31 * result + (jobName?.hashCode() ?: 0)
result = 31 * result + (jobTags?.hashCode() ?: 0)
result = 31 * result + (outputDataConfig?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (trainingDataConfig?.hashCode() ?: 0)
result = 31 * result + (validationDataConfig?.hashCode() ?: 0)
result = 31 * result + (vpcConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateModelCustomizationJobRequest
if (baseModelIdentifier != other.baseModelIdentifier) return false
if (clientRequestToken != other.clientRequestToken) return false
if (customModelKmsKeyId != other.customModelKmsKeyId) return false
if (customModelName != other.customModelName) return false
if (customModelTags != other.customModelTags) return false
if (customizationType != other.customizationType) return false
if (hyperParameters != other.hyperParameters) return false
if (jobName != other.jobName) return false
if (jobTags != other.jobTags) return false
if (outputDataConfig != other.outputDataConfig) return false
if (roleArn != other.roleArn) return false
if (trainingDataConfig != other.trainingDataConfig) return false
if (validationDataConfig != other.validationDataConfig) return false
if (vpcConfig != other.vpcConfig) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.bedrock.model.CreateModelCustomizationJobRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Name of the base model.
*/
public var baseModelIdentifier: kotlin.String? = null
/**
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more information, see [Ensuring idempotency](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/Run_Instance_Idempotency.html).
*/
public var clientRequestToken: kotlin.String? = null
/**
* The custom model is encrypted at rest using this key.
*/
public var customModelKmsKeyId: kotlin.String? = null
/**
* A name for the resulting custom model.
*/
public var customModelName: kotlin.String? = null
/**
* Tags to attach to the resulting custom model.
*/
public var customModelTags: List? = null
/**
* The customization type.
*/
public var customizationType: aws.sdk.kotlin.services.bedrock.model.CustomizationType? = null
/**
* Parameters related to tuning the model. For details on the format for different models, see [Custom model hyperparameters](https://docs.aws.amazon.com/bedrock/latest/userguide/custom-models-hp.html).
*/
public var hyperParameters: Map? = null
/**
* A name for the fine-tuning job.
*/
public var jobName: kotlin.String? = null
/**
* Tags to attach to the job.
*/
public var jobTags: List? = null
/**
* S3 location for the output data.
*/
public var outputDataConfig: aws.sdk.kotlin.services.bedrock.model.OutputDataConfig? = null
/**
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your behalf. For example, during model training, Amazon Bedrock needs your permission to read input data from an S3 bucket, write model artifacts to an S3 bucket. To pass this role to Amazon Bedrock, the caller of this API must have the `iam:PassRole` permission.
*/
public var roleArn: kotlin.String? = null
/**
* Information about the training dataset.
*/
public var trainingDataConfig: aws.sdk.kotlin.services.bedrock.model.TrainingDataConfig? = null
/**
* Information about the validation dataset.
*/
public var validationDataConfig: aws.sdk.kotlin.services.bedrock.model.ValidationDataConfig? = null
/**
* The configuration of the Virtual Private Cloud (VPC) that contains the resources that you're using for this job. For more information, see [Protect your model customization jobs using a VPC](https://docs.aws.amazon.com/bedrock/latest/userguide/vpc-model-customization.html).
*/
public var vpcConfig: aws.sdk.kotlin.services.bedrock.model.VpcConfig? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.bedrock.model.CreateModelCustomizationJobRequest) : this() {
this.baseModelIdentifier = x.baseModelIdentifier
this.clientRequestToken = x.clientRequestToken
this.customModelKmsKeyId = x.customModelKmsKeyId
this.customModelName = x.customModelName
this.customModelTags = x.customModelTags
this.customizationType = x.customizationType
this.hyperParameters = x.hyperParameters
this.jobName = x.jobName
this.jobTags = x.jobTags
this.outputDataConfig = x.outputDataConfig
this.roleArn = x.roleArn
this.trainingDataConfig = x.trainingDataConfig
this.validationDataConfig = x.validationDataConfig
this.vpcConfig = x.vpcConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.bedrock.model.CreateModelCustomizationJobRequest = CreateModelCustomizationJobRequest(this)
/**
* construct an [aws.sdk.kotlin.services.bedrock.model.OutputDataConfig] inside the given [block]
*/
public fun outputDataConfig(block: aws.sdk.kotlin.services.bedrock.model.OutputDataConfig.Builder.() -> kotlin.Unit) {
this.outputDataConfig = aws.sdk.kotlin.services.bedrock.model.OutputDataConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.bedrock.model.TrainingDataConfig] inside the given [block]
*/
public fun trainingDataConfig(block: aws.sdk.kotlin.services.bedrock.model.TrainingDataConfig.Builder.() -> kotlin.Unit) {
this.trainingDataConfig = aws.sdk.kotlin.services.bedrock.model.TrainingDataConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.bedrock.model.ValidationDataConfig] inside the given [block]
*/
public fun validationDataConfig(block: aws.sdk.kotlin.services.bedrock.model.ValidationDataConfig.Builder.() -> kotlin.Unit) {
this.validationDataConfig = aws.sdk.kotlin.services.bedrock.model.ValidationDataConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.bedrock.model.VpcConfig] inside the given [block]
*/
public fun vpcConfig(block: aws.sdk.kotlin.services.bedrock.model.VpcConfig.Builder.() -> kotlin.Unit) {
this.vpcConfig = aws.sdk.kotlin.services.bedrock.model.VpcConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy