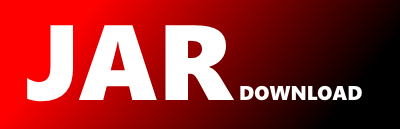
commonMain.aws.sdk.kotlin.services.bedrock.model.FoundationModelSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrock.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Summary information for a foundation model.
*/
public class FoundationModelSummary private constructor(builder: Builder) {
/**
* Whether the model supports fine-tuning or continual pre-training.
*/
public val customizationsSupported: List? = builder.customizationsSupported
/**
* The inference types that the model supports.
*/
public val inferenceTypesSupported: List? = builder.inferenceTypesSupported
/**
* The input modalities that the model supports.
*/
public val inputModalities: List? = builder.inputModalities
/**
* The Amazon Resource Name (ARN) of the foundation model.
*/
public val modelArn: kotlin.String = requireNotNull(builder.modelArn) { "A non-null value must be provided for modelArn" }
/**
* The model ID of the foundation model.
*/
public val modelId: kotlin.String = requireNotNull(builder.modelId) { "A non-null value must be provided for modelId" }
/**
* Contains details about whether a model version is available or deprecated.
*/
public val modelLifecycle: aws.sdk.kotlin.services.bedrock.model.FoundationModelLifecycle? = builder.modelLifecycle
/**
* The name of the model.
*/
public val modelName: kotlin.String? = builder.modelName
/**
* The output modalities that the model supports.
*/
public val outputModalities: List? = builder.outputModalities
/**
* The model's provider name.
*/
public val providerName: kotlin.String? = builder.providerName
/**
* Indicates whether the model supports streaming.
*/
public val responseStreamingSupported: kotlin.Boolean? = builder.responseStreamingSupported
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.bedrock.model.FoundationModelSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("FoundationModelSummary(")
append("customizationsSupported=$customizationsSupported,")
append("inferenceTypesSupported=$inferenceTypesSupported,")
append("inputModalities=$inputModalities,")
append("modelArn=$modelArn,")
append("modelId=$modelId,")
append("modelLifecycle=$modelLifecycle,")
append("modelName=$modelName,")
append("outputModalities=$outputModalities,")
append("providerName=$providerName,")
append("responseStreamingSupported=$responseStreamingSupported")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = customizationsSupported?.hashCode() ?: 0
result = 31 * result + (inferenceTypesSupported?.hashCode() ?: 0)
result = 31 * result + (inputModalities?.hashCode() ?: 0)
result = 31 * result + (modelArn.hashCode())
result = 31 * result + (modelId.hashCode())
result = 31 * result + (modelLifecycle?.hashCode() ?: 0)
result = 31 * result + (modelName?.hashCode() ?: 0)
result = 31 * result + (outputModalities?.hashCode() ?: 0)
result = 31 * result + (providerName?.hashCode() ?: 0)
result = 31 * result + (responseStreamingSupported?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as FoundationModelSummary
if (customizationsSupported != other.customizationsSupported) return false
if (inferenceTypesSupported != other.inferenceTypesSupported) return false
if (inputModalities != other.inputModalities) return false
if (modelArn != other.modelArn) return false
if (modelId != other.modelId) return false
if (modelLifecycle != other.modelLifecycle) return false
if (modelName != other.modelName) return false
if (outputModalities != other.outputModalities) return false
if (providerName != other.providerName) return false
if (responseStreamingSupported != other.responseStreamingSupported) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.bedrock.model.FoundationModelSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Whether the model supports fine-tuning or continual pre-training.
*/
public var customizationsSupported: List? = null
/**
* The inference types that the model supports.
*/
public var inferenceTypesSupported: List? = null
/**
* The input modalities that the model supports.
*/
public var inputModalities: List? = null
/**
* The Amazon Resource Name (ARN) of the foundation model.
*/
public var modelArn: kotlin.String? = null
/**
* The model ID of the foundation model.
*/
public var modelId: kotlin.String? = null
/**
* Contains details about whether a model version is available or deprecated.
*/
public var modelLifecycle: aws.sdk.kotlin.services.bedrock.model.FoundationModelLifecycle? = null
/**
* The name of the model.
*/
public var modelName: kotlin.String? = null
/**
* The output modalities that the model supports.
*/
public var outputModalities: List? = null
/**
* The model's provider name.
*/
public var providerName: kotlin.String? = null
/**
* Indicates whether the model supports streaming.
*/
public var responseStreamingSupported: kotlin.Boolean? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.bedrock.model.FoundationModelSummary) : this() {
this.customizationsSupported = x.customizationsSupported
this.inferenceTypesSupported = x.inferenceTypesSupported
this.inputModalities = x.inputModalities
this.modelArn = x.modelArn
this.modelId = x.modelId
this.modelLifecycle = x.modelLifecycle
this.modelName = x.modelName
this.outputModalities = x.outputModalities
this.providerName = x.providerName
this.responseStreamingSupported = x.responseStreamingSupported
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.bedrock.model.FoundationModelSummary = FoundationModelSummary(this)
/**
* construct an [aws.sdk.kotlin.services.bedrock.model.FoundationModelLifecycle] inside the given [block]
*/
public fun modelLifecycle(block: aws.sdk.kotlin.services.bedrock.model.FoundationModelLifecycle.Builder.() -> kotlin.Unit) {
this.modelLifecycle = aws.sdk.kotlin.services.bedrock.model.FoundationModelLifecycle.invoke(block)
}
internal fun correctErrors(): Builder {
if (modelArn == null) modelArn = ""
if (modelId == null) modelId = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy