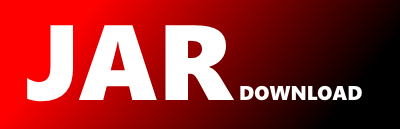
commonMain.aws.sdk.kotlin.services.bedrock.model.ProvisionedModelSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrock.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* A summary of information about a Provisioned Throughput.
*
* This data type is used in the following API operations:
* + [ListProvisionedThroughputs response](https://docs.aws.amazon.com/bedrock/latest/APIReference/API_ListProvisionedModelThroughputs.html#API_ListProvisionedModelThroughputs_ResponseSyntax)
*/
public class ProvisionedModelSummary private constructor(builder: Builder) {
/**
* The duration for which the Provisioned Throughput was committed.
*/
public val commitmentDuration: aws.sdk.kotlin.services.bedrock.model.CommitmentDuration? = builder.commitmentDuration
/**
* The timestamp for when the commitment term of the Provisioned Throughput expires.
*/
public val commitmentExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.commitmentExpirationTime
/**
* The time that the Provisioned Throughput was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.creationTime) { "A non-null value must be provided for creationTime" }
/**
* The Amazon Resource Name (ARN) of the model requested to be associated to this Provisioned Throughput. This value differs from the `modelArn` if updating hasn't completed.
*/
public val desiredModelArn: kotlin.String = requireNotNull(builder.desiredModelArn) { "A non-null value must be provided for desiredModelArn" }
/**
* The number of model units that was requested to be allocated to the Provisioned Throughput.
*/
public val desiredModelUnits: kotlin.Int = requireNotNull(builder.desiredModelUnits) { "A non-null value must be provided for desiredModelUnits" }
/**
* The Amazon Resource Name (ARN) of the base model for which the Provisioned Throughput was created, or of the base model that the custom model for which the Provisioned Throughput was created was customized.
*/
public val foundationModelArn: kotlin.String = requireNotNull(builder.foundationModelArn) { "A non-null value must be provided for foundationModelArn" }
/**
* The time that the Provisioned Throughput was last modified.
*/
public val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastModifiedTime) { "A non-null value must be provided for lastModifiedTime" }
/**
* The Amazon Resource Name (ARN) of the model associated with the Provisioned Throughput.
*/
public val modelArn: kotlin.String = requireNotNull(builder.modelArn) { "A non-null value must be provided for modelArn" }
/**
* The number of model units allocated to the Provisioned Throughput.
*/
public val modelUnits: kotlin.Int = requireNotNull(builder.modelUnits) { "A non-null value must be provided for modelUnits" }
/**
* The Amazon Resource Name (ARN) of the Provisioned Throughput.
*/
public val provisionedModelArn: kotlin.String = requireNotNull(builder.provisionedModelArn) { "A non-null value must be provided for provisionedModelArn" }
/**
* The name of the Provisioned Throughput.
*/
public val provisionedModelName: kotlin.String = requireNotNull(builder.provisionedModelName) { "A non-null value must be provided for provisionedModelName" }
/**
* The status of the Provisioned Throughput.
*/
public val status: aws.sdk.kotlin.services.bedrock.model.ProvisionedModelStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.bedrock.model.ProvisionedModelSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ProvisionedModelSummary(")
append("commitmentDuration=$commitmentDuration,")
append("commitmentExpirationTime=$commitmentExpirationTime,")
append("creationTime=$creationTime,")
append("desiredModelArn=$desiredModelArn,")
append("desiredModelUnits=$desiredModelUnits,")
append("foundationModelArn=$foundationModelArn,")
append("lastModifiedTime=$lastModifiedTime,")
append("modelArn=$modelArn,")
append("modelUnits=$modelUnits,")
append("provisionedModelArn=$provisionedModelArn,")
append("provisionedModelName=$provisionedModelName,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = commitmentDuration?.hashCode() ?: 0
result = 31 * result + (commitmentExpirationTime?.hashCode() ?: 0)
result = 31 * result + (creationTime.hashCode())
result = 31 * result + (desiredModelArn.hashCode())
result = 31 * result + (desiredModelUnits)
result = 31 * result + (foundationModelArn.hashCode())
result = 31 * result + (lastModifiedTime.hashCode())
result = 31 * result + (modelArn.hashCode())
result = 31 * result + (modelUnits)
result = 31 * result + (provisionedModelArn.hashCode())
result = 31 * result + (provisionedModelName.hashCode())
result = 31 * result + (status.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ProvisionedModelSummary
if (commitmentDuration != other.commitmentDuration) return false
if (commitmentExpirationTime != other.commitmentExpirationTime) return false
if (creationTime != other.creationTime) return false
if (desiredModelArn != other.desiredModelArn) return false
if (desiredModelUnits != other.desiredModelUnits) return false
if (foundationModelArn != other.foundationModelArn) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (modelArn != other.modelArn) return false
if (modelUnits != other.modelUnits) return false
if (provisionedModelArn != other.provisionedModelArn) return false
if (provisionedModelName != other.provisionedModelName) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.bedrock.model.ProvisionedModelSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The duration for which the Provisioned Throughput was committed.
*/
public var commitmentDuration: aws.sdk.kotlin.services.bedrock.model.CommitmentDuration? = null
/**
* The timestamp for when the commitment term of the Provisioned Throughput expires.
*/
public var commitmentExpirationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time that the Provisioned Throughput was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the model requested to be associated to this Provisioned Throughput. This value differs from the `modelArn` if updating hasn't completed.
*/
public var desiredModelArn: kotlin.String? = null
/**
* The number of model units that was requested to be allocated to the Provisioned Throughput.
*/
public var desiredModelUnits: kotlin.Int? = null
/**
* The Amazon Resource Name (ARN) of the base model for which the Provisioned Throughput was created, or of the base model that the custom model for which the Provisioned Throughput was created was customized.
*/
public var foundationModelArn: kotlin.String? = null
/**
* The time that the Provisioned Throughput was last modified.
*/
public var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the model associated with the Provisioned Throughput.
*/
public var modelArn: kotlin.String? = null
/**
* The number of model units allocated to the Provisioned Throughput.
*/
public var modelUnits: kotlin.Int? = null
/**
* The Amazon Resource Name (ARN) of the Provisioned Throughput.
*/
public var provisionedModelArn: kotlin.String? = null
/**
* The name of the Provisioned Throughput.
*/
public var provisionedModelName: kotlin.String? = null
/**
* The status of the Provisioned Throughput.
*/
public var status: aws.sdk.kotlin.services.bedrock.model.ProvisionedModelStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.bedrock.model.ProvisionedModelSummary) : this() {
this.commitmentDuration = x.commitmentDuration
this.commitmentExpirationTime = x.commitmentExpirationTime
this.creationTime = x.creationTime
this.desiredModelArn = x.desiredModelArn
this.desiredModelUnits = x.desiredModelUnits
this.foundationModelArn = x.foundationModelArn
this.lastModifiedTime = x.lastModifiedTime
this.modelArn = x.modelArn
this.modelUnits = x.modelUnits
this.provisionedModelArn = x.provisionedModelArn
this.provisionedModelName = x.provisionedModelName
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.bedrock.model.ProvisionedModelSummary = ProvisionedModelSummary(this)
internal fun correctErrors(): Builder {
if (creationTime == null) creationTime = Instant.fromEpochSeconds(0)
if (desiredModelArn == null) desiredModelArn = ""
if (desiredModelUnits == null) desiredModelUnits = 0
if (foundationModelArn == null) foundationModelArn = ""
if (lastModifiedTime == null) lastModifiedTime = Instant.fromEpochSeconds(0)
if (modelArn == null) modelArn = ""
if (modelUnits == null) modelUnits = 0
if (provisionedModelArn == null) provisionedModelArn = ""
if (provisionedModelName == null) provisionedModelName = ""
if (status == null) status = ProvisionedModelStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy