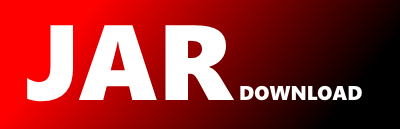
commonMain.aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bedrockruntime-jvm Show documentation
Show all versions of bedrockruntime-jvm Show documentation
The AWS Kotlin client for Bedrock Runtime
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrockruntime.model
/**
* A block of content for a message that you pass to, or receive from, a model with the [Converse](https://docs.aws.amazon.com/bedrock/latest/APIReference/API_runtime_Converse.html) or [ConverseStream](https://docs.aws.amazon.com/bedrock/latest/APIReference/API_runtime_ConverseStream.html) API operations.
*/
public sealed class ContentBlock {
/**
* A document to include in the message.
*/
public data class Document(val value: aws.sdk.kotlin.services.bedrockruntime.model.DocumentBlock) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* Contains the content to assess with the guardrail. If you don't specify `guardContent` in a call to the Converse API, the guardrail (if passed in the Converse API) assesses the entire message.
*
* For more information, see *Use a guardrail with the Converse API* in the *Amazon Bedrock User Guide*.
*/
public data class GuardContent(val value: aws.sdk.kotlin.services.bedrockruntime.model.GuardrailConverseContentBlock) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* Image to include in the message.
*
* This field is only supported by Anthropic Claude 3 models.
*/
public data class Image(val value: aws.sdk.kotlin.services.bedrockruntime.model.ImageBlock) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* Text to include in the message.
*/
public data class Text(val value: kotlin.String) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* The result for a tool request that a model makes.
*/
public data class ToolResult(val value: aws.sdk.kotlin.services.bedrockruntime.model.ToolResultBlock) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* Information about a tool use request from a model.
*/
public data class ToolUse(val value: aws.sdk.kotlin.services.bedrockruntime.model.ToolUseBlock) : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
public object SdkUnknown : aws.sdk.kotlin.services.bedrockruntime.model.ContentBlock() {
}
/**
* Casts this [ContentBlock] as a [Document] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.DocumentBlock] value. Throws an exception if the [ContentBlock] is not a
* [Document].
*/
public fun asDocument(): aws.sdk.kotlin.services.bedrockruntime.model.DocumentBlock = (this as ContentBlock.Document).value
/**
* Casts this [ContentBlock] as a [Document] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.DocumentBlock] value. Returns null if the [ContentBlock] is not a [Document].
*/
public fun asDocumentOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.DocumentBlock? = (this as? ContentBlock.Document)?.value
/**
* Casts this [ContentBlock] as a [GuardContent] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.GuardrailConverseContentBlock] value. Throws an exception if the [ContentBlock] is not a
* [GuardContent].
*/
public fun asGuardContent(): aws.sdk.kotlin.services.bedrockruntime.model.GuardrailConverseContentBlock = (this as ContentBlock.GuardContent).value
/**
* Casts this [ContentBlock] as a [GuardContent] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.GuardrailConverseContentBlock] value. Returns null if the [ContentBlock] is not a [GuardContent].
*/
public fun asGuardContentOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.GuardrailConverseContentBlock? = (this as? ContentBlock.GuardContent)?.value
/**
* Casts this [ContentBlock] as a [Image] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ImageBlock] value. Throws an exception if the [ContentBlock] is not a
* [Image].
*/
public fun asImage(): aws.sdk.kotlin.services.bedrockruntime.model.ImageBlock = (this as ContentBlock.Image).value
/**
* Casts this [ContentBlock] as a [Image] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ImageBlock] value. Returns null if the [ContentBlock] is not a [Image].
*/
public fun asImageOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ImageBlock? = (this as? ContentBlock.Image)?.value
/**
* Casts this [ContentBlock] as a [Text] and retrieves its [kotlin.String] value. Throws an exception if the [ContentBlock] is not a
* [Text].
*/
public fun asText(): kotlin.String = (this as ContentBlock.Text).value
/**
* Casts this [ContentBlock] as a [Text] and retrieves its [kotlin.String] value. Returns null if the [ContentBlock] is not a [Text].
*/
public fun asTextOrNull(): kotlin.String? = (this as? ContentBlock.Text)?.value
/**
* Casts this [ContentBlock] as a [ToolResult] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ToolResultBlock] value. Throws an exception if the [ContentBlock] is not a
* [ToolResult].
*/
public fun asToolResult(): aws.sdk.kotlin.services.bedrockruntime.model.ToolResultBlock = (this as ContentBlock.ToolResult).value
/**
* Casts this [ContentBlock] as a [ToolResult] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ToolResultBlock] value. Returns null if the [ContentBlock] is not a [ToolResult].
*/
public fun asToolResultOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ToolResultBlock? = (this as? ContentBlock.ToolResult)?.value
/**
* Casts this [ContentBlock] as a [ToolUse] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ToolUseBlock] value. Throws an exception if the [ContentBlock] is not a
* [ToolUse].
*/
public fun asToolUse(): aws.sdk.kotlin.services.bedrockruntime.model.ToolUseBlock = (this as ContentBlock.ToolUse).value
/**
* Casts this [ContentBlock] as a [ToolUse] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ToolUseBlock] value. Returns null if the [ContentBlock] is not a [ToolUse].
*/
public fun asToolUseOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ToolUseBlock? = (this as? ContentBlock.ToolUse)?.value
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy