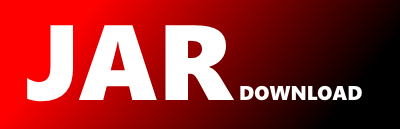
commonMain.aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bedrockruntime-jvm Show documentation
Show all versions of bedrockruntime-jvm Show documentation
The AWS Kotlin client for Bedrock Runtime
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrockruntime.model
/**
* The messages output stream
*/
public sealed class ConverseStreamOutput {
/**
* The messages output content block delta.
*/
public data class ContentBlockDelta(val value: aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockDeltaEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Start information for a content block.
*/
public data class ContentBlockStart(val value: aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStartEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Stop information for a content block.
*/
public data class ContentBlockStop(val value: aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStopEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Message start information.
*/
public data class MessageStart(val value: aws.sdk.kotlin.services.bedrockruntime.model.MessageStartEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Message stop information.
*/
public data class MessageStop(val value: aws.sdk.kotlin.services.bedrockruntime.model.MessageStopEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Metadata for the converse output stream.
*/
public data class Metadata(val value: aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamMetadataEvent) : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
public object SdkUnknown : aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamOutput() {
}
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockDelta] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockDeltaEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [ContentBlockDelta].
*/
public fun asContentBlockDelta(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockDeltaEvent = (this as ConverseStreamOutput.ContentBlockDelta).value
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockDelta] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockDeltaEvent] value. Returns null if the [ConverseStreamOutput] is not a [ContentBlockDelta].
*/
public fun asContentBlockDeltaOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockDeltaEvent? = (this as? ConverseStreamOutput.ContentBlockDelta)?.value
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockStart] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStartEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [ContentBlockStart].
*/
public fun asContentBlockStart(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStartEvent = (this as ConverseStreamOutput.ContentBlockStart).value
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockStart] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStartEvent] value. Returns null if the [ConverseStreamOutput] is not a [ContentBlockStart].
*/
public fun asContentBlockStartOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStartEvent? = (this as? ConverseStreamOutput.ContentBlockStart)?.value
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockStop] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStopEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [ContentBlockStop].
*/
public fun asContentBlockStop(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStopEvent = (this as ConverseStreamOutput.ContentBlockStop).value
/**
* Casts this [ConverseStreamOutput] as a [ContentBlockStop] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStopEvent] value. Returns null if the [ConverseStreamOutput] is not a [ContentBlockStop].
*/
public fun asContentBlockStopOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ContentBlockStopEvent? = (this as? ConverseStreamOutput.ContentBlockStop)?.value
/**
* Casts this [ConverseStreamOutput] as a [MessageStart] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.MessageStartEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [MessageStart].
*/
public fun asMessageStart(): aws.sdk.kotlin.services.bedrockruntime.model.MessageStartEvent = (this as ConverseStreamOutput.MessageStart).value
/**
* Casts this [ConverseStreamOutput] as a [MessageStart] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.MessageStartEvent] value. Returns null if the [ConverseStreamOutput] is not a [MessageStart].
*/
public fun asMessageStartOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.MessageStartEvent? = (this as? ConverseStreamOutput.MessageStart)?.value
/**
* Casts this [ConverseStreamOutput] as a [MessageStop] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.MessageStopEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [MessageStop].
*/
public fun asMessageStop(): aws.sdk.kotlin.services.bedrockruntime.model.MessageStopEvent = (this as ConverseStreamOutput.MessageStop).value
/**
* Casts this [ConverseStreamOutput] as a [MessageStop] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.MessageStopEvent] value. Returns null if the [ConverseStreamOutput] is not a [MessageStop].
*/
public fun asMessageStopOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.MessageStopEvent? = (this as? ConverseStreamOutput.MessageStop)?.value
/**
* Casts this [ConverseStreamOutput] as a [Metadata] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamMetadataEvent] value. Throws an exception if the [ConverseStreamOutput] is not a
* [Metadata].
*/
public fun asMetadata(): aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamMetadataEvent = (this as ConverseStreamOutput.Metadata).value
/**
* Casts this [ConverseStreamOutput] as a [Metadata] and retrieves its [aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamMetadataEvent] value. Returns null if the [ConverseStreamOutput] is not a [Metadata].
*/
public fun asMetadataOrNull(): aws.sdk.kotlin.services.bedrockruntime.model.ConverseStreamMetadataEvent? = (this as? ConverseStreamOutput.Metadata)?.value
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy