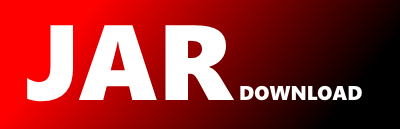
commonMain.aws.sdk.kotlin.services.bedrockruntime.model.InvokeModelRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.bedrockruntime.model
import aws.smithy.kotlin.runtime.SdkDsl
public class InvokeModelRequest private constructor(builder: Builder) {
/**
* The desired MIME type of the inference body in the response. The default value is `application/json`.
*/
public val accept: kotlin.String? = builder.accept
/**
* The prompt and inference parameters in the format specified in the `contentType` in the header. You must provide the body in JSON format. To see the format and content of the request and response bodies for different models, refer to [Inference parameters](https://docs.aws.amazon.com/bedrock/latest/userguide/model-parameters.html). For more information, see [Run inference](https://docs.aws.amazon.com/bedrock/latest/userguide/api-methods-run.html) in the Bedrock User Guide.
*/
public val body: kotlin.ByteArray? = builder.body
/**
* The MIME type of the input data in the request. You must specify `application/json`.
*/
public val contentType: kotlin.String? = builder.contentType
/**
* The unique identifier of the guardrail that you want to use. If you don't provide a value, no guardrail is applied to the invocation.
*
* An error will be thrown in the following situations.
* + You don't provide a guardrail identifier but you specify the `amazon-bedrock-guardrailConfig` field in the request body.
* + You enable the guardrail but the `contentType` isn't `application/json`.
* + You provide a guardrail identifier, but `guardrailVersion` isn't specified.
*/
public val guardrailIdentifier: kotlin.String? = builder.guardrailIdentifier
/**
* The version number for the guardrail. The value can also be `DRAFT`.
*/
public val guardrailVersion: kotlin.String? = builder.guardrailVersion
/**
* The unique identifier of the model to invoke to run inference.
*
* The `modelId` to provide depends on the type of model or throughput that you use:
* + If you use a base model, specify the model ID or its ARN. For a list of model IDs for base models, see [Amazon Bedrock base model IDs (on-demand throughput)](https://docs.aws.amazon.com/bedrock/latest/userguide/model-ids.html#model-ids-arns) in the Amazon Bedrock User Guide.
* + If you use an inference profile, specify the inference profile ID or its ARN. For a list of inference profile IDs, see [Supported Regions and models for cross-region inference](https://docs.aws.amazon.com/bedrock/latest/userguide/cross-region-inference-support.html) in the Amazon Bedrock User Guide.
* + If you use a provisioned model, specify the ARN of the Provisioned Throughput. For more information, see [Run inference using a Provisioned Throughput](https://docs.aws.amazon.com/bedrock/latest/userguide/prov-thru-use.html) in the Amazon Bedrock User Guide.
* + If you use a custom model, first purchase Provisioned Throughput for it. Then specify the ARN of the resulting provisioned model. For more information, see [Use a custom model in Amazon Bedrock](https://docs.aws.amazon.com/bedrock/latest/userguide/model-customization-use.html) in the Amazon Bedrock User Guide.
* + If you use an [imported model](https://docs.aws.amazon.com/bedrock/latest/userguide/model-customization-import-model.html), specify the ARN of the imported model. You can get the model ARN from a successful call to [CreateModelImportJob](https://docs.aws.amazon.com/bedrock/latest/APIReference/API_CreateModelImportJob.html) or from the Imported models page in the Amazon Bedrock console.
*/
public val modelId: kotlin.String? = builder.modelId
/**
* Model performance settings for the request.
*/
public val performanceConfigLatency: aws.sdk.kotlin.services.bedrockruntime.model.PerformanceConfigLatency? = builder.performanceConfigLatency
/**
* Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
*/
public val trace: aws.sdk.kotlin.services.bedrockruntime.model.Trace? = builder.trace
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.bedrockruntime.model.InvokeModelRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("InvokeModelRequest(")
append("accept=$accept,")
append("body=*** Sensitive Data Redacted ***,")
append("contentType=$contentType,")
append("guardrailIdentifier=$guardrailIdentifier,")
append("guardrailVersion=$guardrailVersion,")
append("modelId=$modelId,")
append("performanceConfigLatency=$performanceConfigLatency,")
append("trace=$trace")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accept?.hashCode() ?: 0
result = 31 * result + (body?.contentHashCode() ?: 0)
result = 31 * result + (contentType?.hashCode() ?: 0)
result = 31 * result + (guardrailIdentifier?.hashCode() ?: 0)
result = 31 * result + (guardrailVersion?.hashCode() ?: 0)
result = 31 * result + (modelId?.hashCode() ?: 0)
result = 31 * result + (performanceConfigLatency?.hashCode() ?: 0)
result = 31 * result + (trace?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as InvokeModelRequest
if (accept != other.accept) return false
if (body != null) {
if (other.body == null) return false
if (!body.contentEquals(other.body)) return false
} else if (other.body != null) return false
if (contentType != other.contentType) return false
if (guardrailIdentifier != other.guardrailIdentifier) return false
if (guardrailVersion != other.guardrailVersion) return false
if (modelId != other.modelId) return false
if (performanceConfigLatency != other.performanceConfigLatency) return false
if (trace != other.trace) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.bedrockruntime.model.InvokeModelRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The desired MIME type of the inference body in the response. The default value is `application/json`.
*/
public var accept: kotlin.String? = null
/**
* The prompt and inference parameters in the format specified in the `contentType` in the header. You must provide the body in JSON format. To see the format and content of the request and response bodies for different models, refer to [Inference parameters](https://docs.aws.amazon.com/bedrock/latest/userguide/model-parameters.html). For more information, see [Run inference](https://docs.aws.amazon.com/bedrock/latest/userguide/api-methods-run.html) in the Bedrock User Guide.
*/
public var body: kotlin.ByteArray? = null
/**
* The MIME type of the input data in the request. You must specify `application/json`.
*/
public var contentType: kotlin.String? = null
/**
* The unique identifier of the guardrail that you want to use. If you don't provide a value, no guardrail is applied to the invocation.
*
* An error will be thrown in the following situations.
* + You don't provide a guardrail identifier but you specify the `amazon-bedrock-guardrailConfig` field in the request body.
* + You enable the guardrail but the `contentType` isn't `application/json`.
* + You provide a guardrail identifier, but `guardrailVersion` isn't specified.
*/
public var guardrailIdentifier: kotlin.String? = null
/**
* The version number for the guardrail. The value can also be `DRAFT`.
*/
public var guardrailVersion: kotlin.String? = null
/**
* The unique identifier of the model to invoke to run inference.
*
* The `modelId` to provide depends on the type of model or throughput that you use:
* + If you use a base model, specify the model ID or its ARN. For a list of model IDs for base models, see [Amazon Bedrock base model IDs (on-demand throughput)](https://docs.aws.amazon.com/bedrock/latest/userguide/model-ids.html#model-ids-arns) in the Amazon Bedrock User Guide.
* + If you use an inference profile, specify the inference profile ID or its ARN. For a list of inference profile IDs, see [Supported Regions and models for cross-region inference](https://docs.aws.amazon.com/bedrock/latest/userguide/cross-region-inference-support.html) in the Amazon Bedrock User Guide.
* + If you use a provisioned model, specify the ARN of the Provisioned Throughput. For more information, see [Run inference using a Provisioned Throughput](https://docs.aws.amazon.com/bedrock/latest/userguide/prov-thru-use.html) in the Amazon Bedrock User Guide.
* + If you use a custom model, first purchase Provisioned Throughput for it. Then specify the ARN of the resulting provisioned model. For more information, see [Use a custom model in Amazon Bedrock](https://docs.aws.amazon.com/bedrock/latest/userguide/model-customization-use.html) in the Amazon Bedrock User Guide.
* + If you use an [imported model](https://docs.aws.amazon.com/bedrock/latest/userguide/model-customization-import-model.html), specify the ARN of the imported model. You can get the model ARN from a successful call to [CreateModelImportJob](https://docs.aws.amazon.com/bedrock/latest/APIReference/API_CreateModelImportJob.html) or from the Imported models page in the Amazon Bedrock console.
*/
public var modelId: kotlin.String? = null
/**
* Model performance settings for the request.
*/
public var performanceConfigLatency: aws.sdk.kotlin.services.bedrockruntime.model.PerformanceConfigLatency? = null
/**
* Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
*/
public var trace: aws.sdk.kotlin.services.bedrockruntime.model.Trace? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.bedrockruntime.model.InvokeModelRequest) : this() {
this.accept = x.accept
this.body = x.body
this.contentType = x.contentType
this.guardrailIdentifier = x.guardrailIdentifier
this.guardrailVersion = x.guardrailVersion
this.modelId = x.modelId
this.performanceConfigLatency = x.performanceConfigLatency
this.trace = x.trace
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.bedrockruntime.model.InvokeModelRequest = InvokeModelRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy