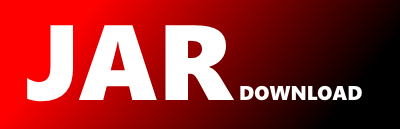
commonMain.aws.sdk.kotlin.services.chatbot.model.SlackUserIdentity.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.chatbot.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Identifes a User level permission for a channel configuration.
*/
public class SlackUserIdentity private constructor(builder: Builder) {
/**
* The AWS user identity ARN used to associate a Slack User Identity with an IAM Role.
*/
public val awsUserIdentity: kotlin.String? = builder.awsUserIdentity
/**
* The ARN of the SlackChannelConfiguration associated with the user identity.
*/
public val chatConfigurationArn: kotlin.String = requireNotNull(builder.chatConfigurationArn) { "A non-null value must be provided for chatConfigurationArn" }
/**
* The ARN of the IAM role that defines the permissions for AWS Chatbot. This is a user-defined role that AWS Chatbot will assume. This is not the service-linked role. For more information, see IAM Policies for AWS Chatbot.
*/
public val iamRoleArn: kotlin.String = requireNotNull(builder.iamRoleArn) { "A non-null value must be provided for iamRoleArn" }
/**
* The ID of the Slack workspace authorized with AWS Chatbot.
*/
public val slackTeamId: kotlin.String = requireNotNull(builder.slackTeamId) { "A non-null value must be provided for slackTeamId" }
/**
* The ID of the user in Slack.
*/
public val slackUserId: kotlin.String = requireNotNull(builder.slackUserId) { "A non-null value must be provided for slackUserId" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.chatbot.model.SlackUserIdentity = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SlackUserIdentity(")
append("awsUserIdentity=$awsUserIdentity,")
append("chatConfigurationArn=$chatConfigurationArn,")
append("iamRoleArn=$iamRoleArn,")
append("slackTeamId=$slackTeamId,")
append("slackUserId=$slackUserId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = awsUserIdentity?.hashCode() ?: 0
result = 31 * result + (chatConfigurationArn.hashCode())
result = 31 * result + (iamRoleArn.hashCode())
result = 31 * result + (slackTeamId.hashCode())
result = 31 * result + (slackUserId.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SlackUserIdentity
if (awsUserIdentity != other.awsUserIdentity) return false
if (chatConfigurationArn != other.chatConfigurationArn) return false
if (iamRoleArn != other.iamRoleArn) return false
if (slackTeamId != other.slackTeamId) return false
if (slackUserId != other.slackUserId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.chatbot.model.SlackUserIdentity = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The AWS user identity ARN used to associate a Slack User Identity with an IAM Role.
*/
public var awsUserIdentity: kotlin.String? = null
/**
* The ARN of the SlackChannelConfiguration associated with the user identity.
*/
public var chatConfigurationArn: kotlin.String? = null
/**
* The ARN of the IAM role that defines the permissions for AWS Chatbot. This is a user-defined role that AWS Chatbot will assume. This is not the service-linked role. For more information, see IAM Policies for AWS Chatbot.
*/
public var iamRoleArn: kotlin.String? = null
/**
* The ID of the Slack workspace authorized with AWS Chatbot.
*/
public var slackTeamId: kotlin.String? = null
/**
* The ID of the user in Slack.
*/
public var slackUserId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.chatbot.model.SlackUserIdentity) : this() {
this.awsUserIdentity = x.awsUserIdentity
this.chatConfigurationArn = x.chatConfigurationArn
this.iamRoleArn = x.iamRoleArn
this.slackTeamId = x.slackTeamId
this.slackUserId = x.slackUserId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.chatbot.model.SlackUserIdentity = SlackUserIdentity(this)
internal fun correctErrors(): Builder {
if (chatConfigurationArn == null) chatConfigurationArn = ""
if (iamRoleArn == null) iamRoleArn = ""
if (slackTeamId == null) slackTeamId = ""
if (slackUserId == null) slackUserId = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy