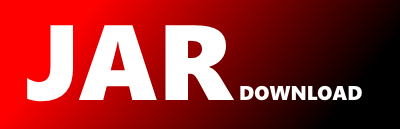
commonMain.aws.sdk.kotlin.services.chatbot.model.UpdateMicrosoftTeamsChannelConfigurationRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.chatbot.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateMicrosoftTeamsChannelConfigurationRequest private constructor(builder: Builder) {
/**
* The ID of the Microsoft Teams channel.
*/
public val channelId: kotlin.String? = builder.channelId
/**
* The name of the Microsoft Teams channel.
*/
public val channelName: kotlin.String? = builder.channelName
/**
* The ARN of the MicrosoftTeamsChannelConfiguration to update.
*/
public val chatConfigurationArn: kotlin.String? = builder.chatConfigurationArn
/**
* The list of IAM policy ARNs that are applied as channel guardrails. The AWS managed 'AdministratorAccess' policy is applied by default if this is not set.
*/
public val guardrailPolicyArns: List? = builder.guardrailPolicyArns
/**
* The ARN of the IAM role that defines the permissions for AWS Chatbot. This is a user-defined role that AWS Chatbot will assume. This is not the service-linked role. For more information, see IAM Policies for AWS Chatbot.
*/
public val iamRoleArn: kotlin.String? = builder.iamRoleArn
/**
* Logging levels include ERROR, INFO, or NONE.
*/
public val loggingLevel: kotlin.String? = builder.loggingLevel
/**
* The ARNs of the SNS topics that deliver notifications to AWS Chatbot.
*/
public val snsTopicArns: List? = builder.snsTopicArns
/**
* Enables use of a user role requirement in your chat configuration.
*/
public val userAuthorizationRequired: kotlin.Boolean? = builder.userAuthorizationRequired
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.chatbot.model.UpdateMicrosoftTeamsChannelConfigurationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateMicrosoftTeamsChannelConfigurationRequest(")
append("channelId=$channelId,")
append("channelName=$channelName,")
append("chatConfigurationArn=$chatConfigurationArn,")
append("guardrailPolicyArns=$guardrailPolicyArns,")
append("iamRoleArn=$iamRoleArn,")
append("loggingLevel=$loggingLevel,")
append("snsTopicArns=$snsTopicArns,")
append("userAuthorizationRequired=$userAuthorizationRequired")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = channelId?.hashCode() ?: 0
result = 31 * result + (channelName?.hashCode() ?: 0)
result = 31 * result + (chatConfigurationArn?.hashCode() ?: 0)
result = 31 * result + (guardrailPolicyArns?.hashCode() ?: 0)
result = 31 * result + (iamRoleArn?.hashCode() ?: 0)
result = 31 * result + (loggingLevel?.hashCode() ?: 0)
result = 31 * result + (snsTopicArns?.hashCode() ?: 0)
result = 31 * result + (userAuthorizationRequired?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateMicrosoftTeamsChannelConfigurationRequest
if (channelId != other.channelId) return false
if (channelName != other.channelName) return false
if (chatConfigurationArn != other.chatConfigurationArn) return false
if (guardrailPolicyArns != other.guardrailPolicyArns) return false
if (iamRoleArn != other.iamRoleArn) return false
if (loggingLevel != other.loggingLevel) return false
if (snsTopicArns != other.snsTopicArns) return false
if (userAuthorizationRequired != other.userAuthorizationRequired) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.chatbot.model.UpdateMicrosoftTeamsChannelConfigurationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the Microsoft Teams channel.
*/
public var channelId: kotlin.String? = null
/**
* The name of the Microsoft Teams channel.
*/
public var channelName: kotlin.String? = null
/**
* The ARN of the MicrosoftTeamsChannelConfiguration to update.
*/
public var chatConfigurationArn: kotlin.String? = null
/**
* The list of IAM policy ARNs that are applied as channel guardrails. The AWS managed 'AdministratorAccess' policy is applied by default if this is not set.
*/
public var guardrailPolicyArns: List? = null
/**
* The ARN of the IAM role that defines the permissions for AWS Chatbot. This is a user-defined role that AWS Chatbot will assume. This is not the service-linked role. For more information, see IAM Policies for AWS Chatbot.
*/
public var iamRoleArn: kotlin.String? = null
/**
* Logging levels include ERROR, INFO, or NONE.
*/
public var loggingLevel: kotlin.String? = null
/**
* The ARNs of the SNS topics that deliver notifications to AWS Chatbot.
*/
public var snsTopicArns: List? = null
/**
* Enables use of a user role requirement in your chat configuration.
*/
public var userAuthorizationRequired: kotlin.Boolean? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.chatbot.model.UpdateMicrosoftTeamsChannelConfigurationRequest) : this() {
this.channelId = x.channelId
this.channelName = x.channelName
this.chatConfigurationArn = x.chatConfigurationArn
this.guardrailPolicyArns = x.guardrailPolicyArns
this.iamRoleArn = x.iamRoleArn
this.loggingLevel = x.loggingLevel
this.snsTopicArns = x.snsTopicArns
this.userAuthorizationRequired = x.userAuthorizationRequired
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.chatbot.model.UpdateMicrosoftTeamsChannelConfigurationRequest = UpdateMicrosoftTeamsChannelConfigurationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy