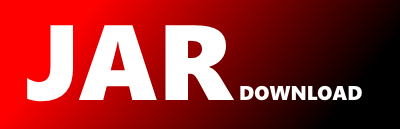
commonMain.aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudsearch-jvm Show documentation
Show all versions of cloudsearch-jvm Show documentation
The AWS SDK for Kotlin client for CloudSearch
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.cloudsearch.model
/**
* Options for a field that contains an array of text strings. Present if `IndexFieldType` specifies the field is of type `text-array`. A `text-array` field is always searchable. All options are enabled by default.
*/
public class TextArrayOptions private constructor(builder: Builder) {
/**
* The name of an analysis scheme for a `text-array` field.
*/
public val analysisScheme: kotlin.String? = builder.analysisScheme
/**
* A value to use for the field if the field isn't specified for a document.
*/
public val defaultValue: kotlin.String? = builder.defaultValue
/**
* Whether highlights can be returned for the field.
*/
public val highlightEnabled: kotlin.Boolean? = builder.highlightEnabled
/**
* Whether the contents of the field can be returned in the search results.
*/
public val returnEnabled: kotlin.Boolean? = builder.returnEnabled
/**
* A list of source fields to map to the field.
*/
public val sourceFields: kotlin.String? = builder.sourceFields
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TextArrayOptions(")
append("analysisScheme=$analysisScheme,")
append("defaultValue=$defaultValue,")
append("highlightEnabled=$highlightEnabled,")
append("returnEnabled=$returnEnabled,")
append("sourceFields=$sourceFields")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = analysisScheme?.hashCode() ?: 0
result = 31 * result + (defaultValue?.hashCode() ?: 0)
result = 31 * result + (highlightEnabled?.hashCode() ?: 0)
result = 31 * result + (returnEnabled?.hashCode() ?: 0)
result = 31 * result + (sourceFields?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TextArrayOptions
if (analysisScheme != other.analysisScheme) return false
if (defaultValue != other.defaultValue) return false
if (highlightEnabled != other.highlightEnabled) return false
if (returnEnabled != other.returnEnabled) return false
if (sourceFields != other.sourceFields) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions = Builder(this).apply(block).build()
public class Builder {
/**
* The name of an analysis scheme for a `text-array` field.
*/
public var analysisScheme: kotlin.String? = null
/**
* A value to use for the field if the field isn't specified for a document.
*/
public var defaultValue: kotlin.String? = null
/**
* Whether highlights can be returned for the field.
*/
public var highlightEnabled: kotlin.Boolean? = null
/**
* Whether the contents of the field can be returned in the search results.
*/
public var returnEnabled: kotlin.Boolean? = null
/**
* A list of source fields to map to the field.
*/
public var sourceFields: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions) : this() {
this.analysisScheme = x.analysisScheme
this.defaultValue = x.defaultValue
this.highlightEnabled = x.highlightEnabled
this.returnEnabled = x.returnEnabled
this.sourceFields = x.sourceFields
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions = TextArrayOptions(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy