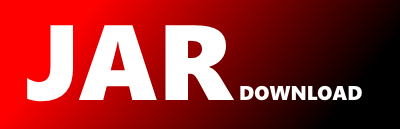
commonMain.aws.sdk.kotlin.services.cloudsearch.model.IndexField.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudsearch-jvm Show documentation
Show all versions of cloudsearch-jvm Show documentation
The AWS SDK for Kotlin client for CloudSearch
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.cloudsearch.model
/**
* Configuration information for a field in the index, including its name, type, and options. The supported options depend on the `IndexFieldType`.
*/
public class IndexField private constructor(builder: Builder) {
/**
* Options for a field that contains an array of dates. Present if `IndexFieldType` specifies the field is of type `date-array`. All options are enabled by default.
*/
public val dateArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.DateArrayOptions? = builder.dateArrayOptions
/**
* Options for a date field. Dates and times are specified in UTC (Coordinated Universal Time) according to IETF RFC3339: yyyy-mm-ddT00:00:00Z. Present if `IndexFieldType` specifies the field is of type `date`. All options are enabled by default.
*/
public val dateOptions: aws.sdk.kotlin.services.cloudsearch.model.DateOptions? = builder.dateOptions
/**
* Options for a field that contains an array of double-precision 64-bit floating point values. Present if `IndexFieldType` specifies the field is of type `double-array`. All options are enabled by default.
*/
public val doubleArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.DoubleArrayOptions? = builder.doubleArrayOptions
/**
* Options for a double-precision 64-bit floating point field. Present if `IndexFieldType` specifies the field is of type `double`. All options are enabled by default.
*/
public val doubleOptions: aws.sdk.kotlin.services.cloudsearch.model.DoubleOptions? = builder.doubleOptions
/**
* A string that represents the name of an index field. CloudSearch supports regular index fields as well as dynamic fields. A dynamic field's name defines a pattern that begins or ends with a wildcard. Any document fields that don't map to a regular index field but do match a dynamic field's pattern are configured with the dynamic field's indexing options.
*
* Regular field names begin with a letter and can contain the following characters: a-z (lowercase), 0-9, and _ (underscore). Dynamic field names must begin or end with a wildcard (*). The wildcard can also be the only character in a dynamic field name. Multiple wildcards, and wildcards embedded within a string are not supported.
*
* The name `score` is reserved and cannot be used as a field name. To reference a document's ID, you can use the name `_id`.
*/
public val indexFieldName: kotlin.String = requireNotNull(builder.indexFieldName) { "A non-null value must be provided for indexFieldName" }
/**
* The type of field. The valid options for a field depend on the field type. For more information about the supported field types, see [Configuring Index Fields](http://docs.aws.amazon.com/cloudsearch/latest/developerguide/configuring-index-fields.html) in the *Amazon CloudSearch Developer Guide*.
*/
public val indexFieldType: aws.sdk.kotlin.services.cloudsearch.model.IndexFieldType = requireNotNull(builder.indexFieldType) { "A non-null value must be provided for indexFieldType" }
/**
* Options for a field that contains an array of 64-bit signed integers. Present if `IndexFieldType` specifies the field is of type `int-array`. All options are enabled by default.
*/
public val intArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.IntArrayOptions? = builder.intArrayOptions
/**
* Options for a 64-bit signed integer field. Present if `IndexFieldType` specifies the field is of type `int`. All options are enabled by default.
*/
public val intOptions: aws.sdk.kotlin.services.cloudsearch.model.IntOptions? = builder.intOptions
/**
* Options for a latlon field. A latlon field contains a location stored as a latitude and longitude value pair. Present if `IndexFieldType` specifies the field is of type `latlon`. All options are enabled by default.
*/
public val latLonOptions: aws.sdk.kotlin.services.cloudsearch.model.LatLonOptions? = builder.latLonOptions
/**
* Options for a field that contains an array of literal strings. Present if `IndexFieldType` specifies the field is of type `literal-array`. All options are enabled by default.
*/
public val literalArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.LiteralArrayOptions? = builder.literalArrayOptions
/**
* Options for literal field. Present if `IndexFieldType` specifies the field is of type `literal`. All options are enabled by default.
*/
public val literalOptions: aws.sdk.kotlin.services.cloudsearch.model.LiteralOptions? = builder.literalOptions
/**
* Options for a field that contains an array of text strings. Present if `IndexFieldType` specifies the field is of type `text-array`. A `text-array` field is always searchable. All options are enabled by default.
*/
public val textArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions? = builder.textArrayOptions
/**
* Options for text field. Present if `IndexFieldType` specifies the field is of type `text`. A `text` field is always searchable. All options are enabled by default.
*/
public val textOptions: aws.sdk.kotlin.services.cloudsearch.model.TextOptions? = builder.textOptions
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.cloudsearch.model.IndexField = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("IndexField(")
append("dateArrayOptions=$dateArrayOptions,")
append("dateOptions=$dateOptions,")
append("doubleArrayOptions=$doubleArrayOptions,")
append("doubleOptions=$doubleOptions,")
append("indexFieldName=$indexFieldName,")
append("indexFieldType=$indexFieldType,")
append("intArrayOptions=$intArrayOptions,")
append("intOptions=$intOptions,")
append("latLonOptions=$latLonOptions,")
append("literalArrayOptions=$literalArrayOptions,")
append("literalOptions=$literalOptions,")
append("textArrayOptions=$textArrayOptions,")
append("textOptions=$textOptions")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dateArrayOptions?.hashCode() ?: 0
result = 31 * result + (dateOptions?.hashCode() ?: 0)
result = 31 * result + (doubleArrayOptions?.hashCode() ?: 0)
result = 31 * result + (doubleOptions?.hashCode() ?: 0)
result = 31 * result + (indexFieldName.hashCode())
result = 31 * result + (indexFieldType.hashCode())
result = 31 * result + (intArrayOptions?.hashCode() ?: 0)
result = 31 * result + (intOptions?.hashCode() ?: 0)
result = 31 * result + (latLonOptions?.hashCode() ?: 0)
result = 31 * result + (literalArrayOptions?.hashCode() ?: 0)
result = 31 * result + (literalOptions?.hashCode() ?: 0)
result = 31 * result + (textArrayOptions?.hashCode() ?: 0)
result = 31 * result + (textOptions?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as IndexField
if (dateArrayOptions != other.dateArrayOptions) return false
if (dateOptions != other.dateOptions) return false
if (doubleArrayOptions != other.doubleArrayOptions) return false
if (doubleOptions != other.doubleOptions) return false
if (indexFieldName != other.indexFieldName) return false
if (indexFieldType != other.indexFieldType) return false
if (intArrayOptions != other.intArrayOptions) return false
if (intOptions != other.intOptions) return false
if (latLonOptions != other.latLonOptions) return false
if (literalArrayOptions != other.literalArrayOptions) return false
if (literalOptions != other.literalOptions) return false
if (textArrayOptions != other.textArrayOptions) return false
if (textOptions != other.textOptions) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.cloudsearch.model.IndexField = Builder(this).apply(block).build()
public class Builder {
/**
* Options for a field that contains an array of dates. Present if `IndexFieldType` specifies the field is of type `date-array`. All options are enabled by default.
*/
public var dateArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.DateArrayOptions? = null
/**
* Options for a date field. Dates and times are specified in UTC (Coordinated Universal Time) according to IETF RFC3339: yyyy-mm-ddT00:00:00Z. Present if `IndexFieldType` specifies the field is of type `date`. All options are enabled by default.
*/
public var dateOptions: aws.sdk.kotlin.services.cloudsearch.model.DateOptions? = null
/**
* Options for a field that contains an array of double-precision 64-bit floating point values. Present if `IndexFieldType` specifies the field is of type `double-array`. All options are enabled by default.
*/
public var doubleArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.DoubleArrayOptions? = null
/**
* Options for a double-precision 64-bit floating point field. Present if `IndexFieldType` specifies the field is of type `double`. All options are enabled by default.
*/
public var doubleOptions: aws.sdk.kotlin.services.cloudsearch.model.DoubleOptions? = null
/**
* A string that represents the name of an index field. CloudSearch supports regular index fields as well as dynamic fields. A dynamic field's name defines a pattern that begins or ends with a wildcard. Any document fields that don't map to a regular index field but do match a dynamic field's pattern are configured with the dynamic field's indexing options.
*
* Regular field names begin with a letter and can contain the following characters: a-z (lowercase), 0-9, and _ (underscore). Dynamic field names must begin or end with a wildcard (*). The wildcard can also be the only character in a dynamic field name. Multiple wildcards, and wildcards embedded within a string are not supported.
*
* The name `score` is reserved and cannot be used as a field name. To reference a document's ID, you can use the name `_id`.
*/
public var indexFieldName: kotlin.String? = null
/**
* The type of field. The valid options for a field depend on the field type. For more information about the supported field types, see [Configuring Index Fields](http://docs.aws.amazon.com/cloudsearch/latest/developerguide/configuring-index-fields.html) in the *Amazon CloudSearch Developer Guide*.
*/
public var indexFieldType: aws.sdk.kotlin.services.cloudsearch.model.IndexFieldType? = null
/**
* Options for a field that contains an array of 64-bit signed integers. Present if `IndexFieldType` specifies the field is of type `int-array`. All options are enabled by default.
*/
public var intArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.IntArrayOptions? = null
/**
* Options for a 64-bit signed integer field. Present if `IndexFieldType` specifies the field is of type `int`. All options are enabled by default.
*/
public var intOptions: aws.sdk.kotlin.services.cloudsearch.model.IntOptions? = null
/**
* Options for a latlon field. A latlon field contains a location stored as a latitude and longitude value pair. Present if `IndexFieldType` specifies the field is of type `latlon`. All options are enabled by default.
*/
public var latLonOptions: aws.sdk.kotlin.services.cloudsearch.model.LatLonOptions? = null
/**
* Options for a field that contains an array of literal strings. Present if `IndexFieldType` specifies the field is of type `literal-array`. All options are enabled by default.
*/
public var literalArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.LiteralArrayOptions? = null
/**
* Options for literal field. Present if `IndexFieldType` specifies the field is of type `literal`. All options are enabled by default.
*/
public var literalOptions: aws.sdk.kotlin.services.cloudsearch.model.LiteralOptions? = null
/**
* Options for a field that contains an array of text strings. Present if `IndexFieldType` specifies the field is of type `text-array`. A `text-array` field is always searchable. All options are enabled by default.
*/
public var textArrayOptions: aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions? = null
/**
* Options for text field. Present if `IndexFieldType` specifies the field is of type `text`. A `text` field is always searchable. All options are enabled by default.
*/
public var textOptions: aws.sdk.kotlin.services.cloudsearch.model.TextOptions? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.cloudsearch.model.IndexField) : this() {
this.dateArrayOptions = x.dateArrayOptions
this.dateOptions = x.dateOptions
this.doubleArrayOptions = x.doubleArrayOptions
this.doubleOptions = x.doubleOptions
this.indexFieldName = x.indexFieldName
this.indexFieldType = x.indexFieldType
this.intArrayOptions = x.intArrayOptions
this.intOptions = x.intOptions
this.latLonOptions = x.latLonOptions
this.literalArrayOptions = x.literalArrayOptions
this.literalOptions = x.literalOptions
this.textArrayOptions = x.textArrayOptions
this.textOptions = x.textOptions
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.cloudsearch.model.IndexField = IndexField(this)
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.DateArrayOptions] inside the given [block]
*/
public fun dateArrayOptions(block: aws.sdk.kotlin.services.cloudsearch.model.DateArrayOptions.Builder.() -> kotlin.Unit) {
this.dateArrayOptions = aws.sdk.kotlin.services.cloudsearch.model.DateArrayOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.DateOptions] inside the given [block]
*/
public fun dateOptions(block: aws.sdk.kotlin.services.cloudsearch.model.DateOptions.Builder.() -> kotlin.Unit) {
this.dateOptions = aws.sdk.kotlin.services.cloudsearch.model.DateOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.DoubleArrayOptions] inside the given [block]
*/
public fun doubleArrayOptions(block: aws.sdk.kotlin.services.cloudsearch.model.DoubleArrayOptions.Builder.() -> kotlin.Unit) {
this.doubleArrayOptions = aws.sdk.kotlin.services.cloudsearch.model.DoubleArrayOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.DoubleOptions] inside the given [block]
*/
public fun doubleOptions(block: aws.sdk.kotlin.services.cloudsearch.model.DoubleOptions.Builder.() -> kotlin.Unit) {
this.doubleOptions = aws.sdk.kotlin.services.cloudsearch.model.DoubleOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.IntArrayOptions] inside the given [block]
*/
public fun intArrayOptions(block: aws.sdk.kotlin.services.cloudsearch.model.IntArrayOptions.Builder.() -> kotlin.Unit) {
this.intArrayOptions = aws.sdk.kotlin.services.cloudsearch.model.IntArrayOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.IntOptions] inside the given [block]
*/
public fun intOptions(block: aws.sdk.kotlin.services.cloudsearch.model.IntOptions.Builder.() -> kotlin.Unit) {
this.intOptions = aws.sdk.kotlin.services.cloudsearch.model.IntOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.LatLonOptions] inside the given [block]
*/
public fun latLonOptions(block: aws.sdk.kotlin.services.cloudsearch.model.LatLonOptions.Builder.() -> kotlin.Unit) {
this.latLonOptions = aws.sdk.kotlin.services.cloudsearch.model.LatLonOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.LiteralArrayOptions] inside the given [block]
*/
public fun literalArrayOptions(block: aws.sdk.kotlin.services.cloudsearch.model.LiteralArrayOptions.Builder.() -> kotlin.Unit) {
this.literalArrayOptions = aws.sdk.kotlin.services.cloudsearch.model.LiteralArrayOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.LiteralOptions] inside the given [block]
*/
public fun literalOptions(block: aws.sdk.kotlin.services.cloudsearch.model.LiteralOptions.Builder.() -> kotlin.Unit) {
this.literalOptions = aws.sdk.kotlin.services.cloudsearch.model.LiteralOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions] inside the given [block]
*/
public fun textArrayOptions(block: aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions.Builder.() -> kotlin.Unit) {
this.textArrayOptions = aws.sdk.kotlin.services.cloudsearch.model.TextArrayOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.cloudsearch.model.TextOptions] inside the given [block]
*/
public fun textOptions(block: aws.sdk.kotlin.services.cloudsearch.model.TextOptions.Builder.() -> kotlin.Unit) {
this.textOptions = aws.sdk.kotlin.services.cloudsearch.model.TextOptions.invoke(block)
}
internal fun correctErrors(): Builder {
if (indexFieldName == null) indexFieldName = ""
if (indexFieldType == null) indexFieldType = IndexFieldType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy