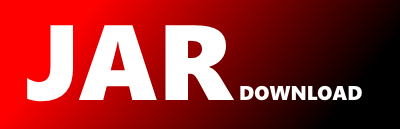
commonMain.aws.sdk.kotlin.services.cloudwatchlogs.model.GetLogEventsRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudwatchlogs Show documentation
Show all versions of cloudwatchlogs Show documentation
The AWS Kotlin client for CloudWatch Logs
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.cloudwatchlogs.model
public class GetLogEventsRequest private constructor(builder: Builder) {
/**
* The end of the time range, expressed as the number of milliseconds after `Jan 1, 1970 00:00:00 UTC`. Events with a timestamp equal to or later than this time are not included.
*/
public val endTime: kotlin.Long? = builder.endTime
/**
* The maximum number of log events returned. If you don't specify a limit, the default is as many log events as can fit in a response size of 1 MB (up to 10,000 log events).
*/
public val limit: kotlin.Int? = builder.limit
/**
* Specify either the name or ARN of the log group to view events from. If the log group is in a source account and you are using a monitoring account, you must use the log group ARN.
*
* You must include either `logGroupIdentifier` or `logGroupName`, but not both.
*/
public val logGroupIdentifier: kotlin.String? = builder.logGroupIdentifier
/**
* The name of the log group.
*
* You must include either `logGroupIdentifier` or `logGroupName`, but not both.
*/
public val logGroupName: kotlin.String? = builder.logGroupName
/**
* The name of the log stream.
*/
public val logStreamName: kotlin.String? = builder.logStreamName
/**
* The token for the next set of items to return. (You received this token from a previous call.)
*/
public val nextToken: kotlin.String? = builder.nextToken
/**
* If the value is true, the earliest log events are returned first. If the value is false, the latest log events are returned first. The default value is false.
*
* If you are using a previous `nextForwardToken` value as the `nextToken` in this operation, you must specify `true` for `startFromHead`.
*/
public val startFromHead: kotlin.Boolean? = builder.startFromHead
/**
* The start of the time range, expressed as the number of milliseconds after `Jan 1, 1970 00:00:00 UTC`. Events with a timestamp equal to this time or later than this time are included. Events with a timestamp earlier than this time are not included.
*/
public val startTime: kotlin.Long? = builder.startTime
/**
* Specify `true` to display the log event fields with all sensitive data unmasked and visible. The default is `false`.
*
* To use this operation with this parameter, you must be signed into an account with the `logs:Unmask` permission.
*/
public val unmask: kotlin.Boolean = builder.unmask
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.cloudwatchlogs.model.GetLogEventsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetLogEventsRequest(")
append("endTime=$endTime,")
append("limit=$limit,")
append("logGroupIdentifier=$logGroupIdentifier,")
append("logGroupName=$logGroupName,")
append("logStreamName=$logStreamName,")
append("nextToken=$nextToken,")
append("startFromHead=$startFromHead,")
append("startTime=$startTime,")
append("unmask=$unmask")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = endTime?.hashCode() ?: 0
result = 31 * result + (limit ?: 0)
result = 31 * result + (logGroupIdentifier?.hashCode() ?: 0)
result = 31 * result + (logGroupName?.hashCode() ?: 0)
result = 31 * result + (logStreamName?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (startFromHead?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (unmask.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetLogEventsRequest
if (endTime != other.endTime) return false
if (limit != other.limit) return false
if (logGroupIdentifier != other.logGroupIdentifier) return false
if (logGroupName != other.logGroupName) return false
if (logStreamName != other.logStreamName) return false
if (nextToken != other.nextToken) return false
if (startFromHead != other.startFromHead) return false
if (startTime != other.startTime) return false
if (unmask != other.unmask) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.cloudwatchlogs.model.GetLogEventsRequest = Builder(this).apply(block).build()
public class Builder {
/**
* The end of the time range, expressed as the number of milliseconds after `Jan 1, 1970 00:00:00 UTC`. Events with a timestamp equal to or later than this time are not included.
*/
public var endTime: kotlin.Long? = null
/**
* The maximum number of log events returned. If you don't specify a limit, the default is as many log events as can fit in a response size of 1 MB (up to 10,000 log events).
*/
public var limit: kotlin.Int? = null
/**
* Specify either the name or ARN of the log group to view events from. If the log group is in a source account and you are using a monitoring account, you must use the log group ARN.
*
* You must include either `logGroupIdentifier` or `logGroupName`, but not both.
*/
public var logGroupIdentifier: kotlin.String? = null
/**
* The name of the log group.
*
* You must include either `logGroupIdentifier` or `logGroupName`, but not both.
*/
public var logGroupName: kotlin.String? = null
/**
* The name of the log stream.
*/
public var logStreamName: kotlin.String? = null
/**
* The token for the next set of items to return. (You received this token from a previous call.)
*/
public var nextToken: kotlin.String? = null
/**
* If the value is true, the earliest log events are returned first. If the value is false, the latest log events are returned first. The default value is false.
*
* If you are using a previous `nextForwardToken` value as the `nextToken` in this operation, you must specify `true` for `startFromHead`.
*/
public var startFromHead: kotlin.Boolean? = null
/**
* The start of the time range, expressed as the number of milliseconds after `Jan 1, 1970 00:00:00 UTC`. Events with a timestamp equal to this time or later than this time are included. Events with a timestamp earlier than this time are not included.
*/
public var startTime: kotlin.Long? = null
/**
* Specify `true` to display the log event fields with all sensitive data unmasked and visible. The default is `false`.
*
* To use this operation with this parameter, you must be signed into an account with the `logs:Unmask` permission.
*/
public var unmask: kotlin.Boolean = false
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.cloudwatchlogs.model.GetLogEventsRequest) : this() {
this.endTime = x.endTime
this.limit = x.limit
this.logGroupIdentifier = x.logGroupIdentifier
this.logGroupName = x.logGroupName
this.logStreamName = x.logStreamName
this.nextToken = x.nextToken
this.startFromHead = x.startFromHead
this.startTime = x.startTime
this.unmask = x.unmask
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.cloudwatchlogs.model.GetLogEventsRequest = GetLogEventsRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy