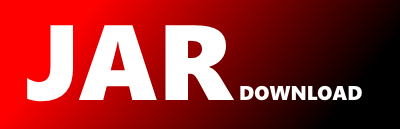
commonMain.aws.sdk.kotlin.services.codebuild.CodeBuildClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild-jvm Show documentation
Show all versions of codebuild-jvm Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.internal.borrow
import aws.sdk.kotlin.runtime.endpoint.AwsEndpointResolver
import aws.sdk.kotlin.runtime.region.resolveRegion
import aws.sdk.kotlin.services.codebuild.internal.DefaultEndpointResolver
import aws.sdk.kotlin.services.codebuild.model.*
import aws.smithy.kotlin.runtime.SdkClient
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProvider
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigner
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkLogMode
import aws.smithy.kotlin.runtime.config.SdkClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.endpoints.EndpointResolver
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.StandardRetryStrategy
/**
* # CodeBuild
* CodeBuild is a fully managed build service in the cloud. CodeBuild compiles your source code, runs unit tests, and produces artifacts that are ready to deploy. CodeBuild eliminates the need to provision, manage, and scale your own build servers. It provides prepackaged build environments for the most popular programming languages and build tools, such as Apache Maven, Gradle, and more. You can also fully customize build environments in CodeBuild to use your own build tools. CodeBuild scales automatically to meet peak build requests. You pay only for the build time you consume. For more information about CodeBuild, see the *[CodeBuild User Guide](https://docs.aws.amazon.com/codebuild/latest/userguide/welcome.html).*
*/
interface CodeBuildClient : SdkClient {
override val serviceName: String
get() = "CodeBuild"
/**
* CodeBuildClient's configuration
*/
val config: Config
companion object {
operator fun invoke(block: Config.Builder.() -> Unit): CodeBuildClient {
val config = Config.Builder().apply(block).build()
return DefaultCodeBuildClient(config)
}
operator fun invoke(config: Config): CodeBuildClient = DefaultCodeBuildClient(config)
/**
* Construct a [CodeBuildClient] by resolving the configuration from the current environment.
*/
suspend fun fromEnvironment(block: (Config.Builder.() -> Unit)? = null): CodeBuildClient {
val builder = Config.Builder()
if (block != null) builder.apply(block)
builder.region = builder.region ?: resolveRegion()
return DefaultCodeBuildClient(builder.build())
}
}
class Config private constructor(builder: Builder): HttpClientConfig, SdkClientConfig {
val credentialsProvider: CredentialsProvider = builder.credentialsProvider?.borrow() ?: DefaultChainCredentialsProvider()
val endpointResolver: AwsEndpointResolver = builder.endpointResolver ?: DefaultEndpointResolver()
override val httpClientEngine: HttpClientEngine? = builder.httpClientEngine
val region: String = requireNotNull(builder.region) { "region is a required configuration property" }
val retryStrategy: RetryStrategy = StandardRetryStrategy()
override val sdkLogMode: SdkLogMode = builder.sdkLogMode
val signer: AwsSigner = builder.signer ?: DefaultAwsSigner
companion object {
inline operator fun invoke(block: Builder.() -> kotlin.Unit): Config = Builder().apply(block).build()
}
class Builder {
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
* NOTE: The caller is responsible for managing the lifetime of the provider when set. The SDK
* client will not close it when the client is closed.
*/
var credentialsProvider: CredentialsProvider? = null
/**
* Determines the endpoint (hostname) to make requests to. When not provided a default
* resolver is configured automatically. This is an advanced client option.
*/
var endpointResolver: AwsEndpointResolver? = null
/**
* Override the default HTTP client engine used to make SDK requests (e.g. configure proxy behavior, timeouts, concurrency, etc).
* NOTE: The caller is responsible for managing the lifetime of the engine when set. The SDK
* client will not close it when the client is closed.
*/
var httpClientEngine: HttpClientEngine? = null
/**
* AWS region to make requests to
*/
var region: String? = null
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
*
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
*
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
var sdkLogMode: SdkLogMode = SdkLogMode.Default
/**
* The implementation of AWS signer to use for signing requests
*/
var signer: AwsSigner? = null
@PublishedApi
internal fun build(): Config = Config(this)
}
}
/**
* Deletes one or more builds.
*/
suspend fun batchDeleteBuilds(input: BatchDeleteBuildsRequest): BatchDeleteBuildsResponse
/**
* Deletes one or more builds.
*/
suspend fun batchDeleteBuilds(block: BatchDeleteBuildsRequest.Builder.() -> Unit) = batchDeleteBuilds(BatchDeleteBuildsRequest.Builder().apply(block).build())
/**
* Retrieves information about one or more batch builds.
*/
suspend fun batchGetBuildBatches(input: BatchGetBuildBatchesRequest): BatchGetBuildBatchesResponse
/**
* Retrieves information about one or more batch builds.
*/
suspend fun batchGetBuildBatches(block: BatchGetBuildBatchesRequest.Builder.() -> Unit) = batchGetBuildBatches(BatchGetBuildBatchesRequest.Builder().apply(block).build())
/**
* Gets information about one or more builds.
*/
suspend fun batchGetBuilds(input: BatchGetBuildsRequest): BatchGetBuildsResponse
/**
* Gets information about one or more builds.
*/
suspend fun batchGetBuilds(block: BatchGetBuildsRequest.Builder.() -> Unit) = batchGetBuilds(BatchGetBuildsRequest.Builder().apply(block).build())
/**
* Gets information about one or more build projects.
*/
suspend fun batchGetProjects(input: BatchGetProjectsRequest): BatchGetProjectsResponse
/**
* Gets information about one or more build projects.
*/
suspend fun batchGetProjects(block: BatchGetProjectsRequest.Builder.() -> Unit) = batchGetProjects(BatchGetProjectsRequest.Builder().apply(block).build())
/**
* Returns an array of report groups.
*/
suspend fun batchGetReportGroups(input: BatchGetReportGroupsRequest): BatchGetReportGroupsResponse
/**
* Returns an array of report groups.
*/
suspend fun batchGetReportGroups(block: BatchGetReportGroupsRequest.Builder.() -> Unit) = batchGetReportGroups(BatchGetReportGroupsRequest.Builder().apply(block).build())
/**
* Returns an array of reports.
*/
suspend fun batchGetReports(input: BatchGetReportsRequest): BatchGetReportsResponse
/**
* Returns an array of reports.
*/
suspend fun batchGetReports(block: BatchGetReportsRequest.Builder.() -> Unit) = batchGetReports(BatchGetReportsRequest.Builder().apply(block).build())
/**
* Creates a build project.
*/
suspend fun createProject(input: CreateProjectRequest): CreateProjectResponse
/**
* Creates a build project.
*/
suspend fun createProject(block: CreateProjectRequest.Builder.() -> Unit) = createProject(CreateProjectRequest.Builder().apply(block).build())
/**
* Creates a report group. A report group contains a collection of reports.
*/
suspend fun createReportGroup(input: CreateReportGroupRequest): CreateReportGroupResponse
/**
* Creates a report group. A report group contains a collection of reports.
*/
suspend fun createReportGroup(block: CreateReportGroupRequest.Builder.() -> Unit) = createReportGroup(CreateReportGroupRequest.Builder().apply(block).build())
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository, enables CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
* If you enable webhooks for an CodeBuild project, and the project is used as a build step in CodePipeline, then two identical builds are created for each commit. One build is triggered through webhooks, and one through CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are using CodePipeline, we recommend that you disable webhooks in CodeBuild. In the CodeBuild console, clear the Webhook box. For more information, see step 5 in [Change a Build Project's Settings](https://docs.aws.amazon.com/codebuild/latest/userguide/change-project.html#change-project-console).
*/
suspend fun createWebhook(input: CreateWebhookRequest): CreateWebhookResponse
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository, enables CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
* If you enable webhooks for an CodeBuild project, and the project is used as a build step in CodePipeline, then two identical builds are created for each commit. One build is triggered through webhooks, and one through CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are using CodePipeline, we recommend that you disable webhooks in CodeBuild. In the CodeBuild console, clear the Webhook box. For more information, see step 5 in [Change a Build Project's Settings](https://docs.aws.amazon.com/codebuild/latest/userguide/change-project.html#change-project-console).
*/
suspend fun createWebhook(block: CreateWebhookRequest.Builder.() -> Unit) = createWebhook(CreateWebhookRequest.Builder().apply(block).build())
/**
* Deletes a batch build.
*/
suspend fun deleteBuildBatch(input: DeleteBuildBatchRequest): DeleteBuildBatchResponse
/**
* Deletes a batch build.
*/
suspend fun deleteBuildBatch(block: DeleteBuildBatchRequest.Builder.() -> Unit) = deleteBuildBatch(DeleteBuildBatchRequest.Builder().apply(block).build())
/**
* Deletes a build project. When you delete a project, its builds are not deleted.
*/
suspend fun deleteProject(input: DeleteProjectRequest): DeleteProjectResponse
/**
* Deletes a build project. When you delete a project, its builds are not deleted.
*/
suspend fun deleteProject(block: DeleteProjectRequest.Builder.() -> Unit) = deleteProject(DeleteProjectRequest.Builder().apply(block).build())
/**
* Deletes a report.
*/
suspend fun deleteReport(input: DeleteReportRequest): DeleteReportResponse
/**
* Deletes a report.
*/
suspend fun deleteReport(block: DeleteReportRequest.Builder.() -> Unit) = deleteReport(DeleteReportRequest.Builder().apply(block).build())
/**
* Deletes a report group. Before you delete a report group, you must delete its reports.
*/
suspend fun deleteReportGroup(input: DeleteReportGroupRequest): DeleteReportGroupResponse
/**
* Deletes a report group. Before you delete a report group, you must delete its reports.
*/
suspend fun deleteReportGroup(block: DeleteReportGroupRequest.Builder.() -> Unit) = deleteReportGroup(DeleteReportGroupRequest.Builder().apply(block).build())
/**
* Deletes a resource policy that is identified by its resource ARN.
*/
suspend fun deleteResourcePolicy(input: DeleteResourcePolicyRequest): DeleteResourcePolicyResponse
/**
* Deletes a resource policy that is identified by its resource ARN.
*/
suspend fun deleteResourcePolicy(block: DeleteResourcePolicyRequest.Builder.() -> Unit) = deleteResourcePolicy(DeleteResourcePolicyRequest.Builder().apply(block).build())
/**
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*/
suspend fun deleteSourceCredentials(input: DeleteSourceCredentialsRequest): DeleteSourceCredentialsResponse
/**
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*/
suspend fun deleteSourceCredentials(block: DeleteSourceCredentialsRequest.Builder.() -> Unit) = deleteSourceCredentials(DeleteSourceCredentialsRequest.Builder().apply(block).build())
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository, stops CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*/
suspend fun deleteWebhook(input: DeleteWebhookRequest): DeleteWebhookResponse
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository, stops CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*/
suspend fun deleteWebhook(block: DeleteWebhookRequest.Builder.() -> Unit) = deleteWebhook(DeleteWebhookRequest.Builder().apply(block).build())
/**
* Retrieves one or more code coverage reports.
*/
suspend fun describeCodeCoverages(input: DescribeCodeCoveragesRequest): DescribeCodeCoveragesResponse
/**
* Retrieves one or more code coverage reports.
*/
suspend fun describeCodeCoverages(block: DescribeCodeCoveragesRequest.Builder.() -> Unit) = describeCodeCoverages(DescribeCodeCoveragesRequest.Builder().apply(block).build())
/**
* Returns a list of details about test cases for a report.
*/
suspend fun describeTestCases(input: DescribeTestCasesRequest): DescribeTestCasesResponse
/**
* Returns a list of details about test cases for a report.
*/
suspend fun describeTestCases(block: DescribeTestCasesRequest.Builder.() -> Unit) = describeTestCases(DescribeTestCasesRequest.Builder().apply(block).build())
/**
* Analyzes and accumulates test report values for the specified test reports.
*/
suspend fun getReportGroupTrend(input: GetReportGroupTrendRequest): GetReportGroupTrendResponse
/**
* Analyzes and accumulates test report values for the specified test reports.
*/
suspend fun getReportGroupTrend(block: GetReportGroupTrendRequest.Builder.() -> Unit) = getReportGroupTrend(GetReportGroupTrendRequest.Builder().apply(block).build())
/**
* Gets a resource policy that is identified by its resource ARN.
*/
suspend fun getResourcePolicy(input: GetResourcePolicyRequest): GetResourcePolicyResponse
/**
* Gets a resource policy that is identified by its resource ARN.
*/
suspend fun getResourcePolicy(block: GetResourcePolicyRequest.Builder.() -> Unit) = getResourcePolicy(GetResourcePolicyRequest.Builder().apply(block).build())
/**
* Imports the source repository credentials for an CodeBuild project that has its source code stored in a GitHub, GitHub Enterprise, or Bitbucket repository.
*/
suspend fun importSourceCredentials(input: ImportSourceCredentialsRequest): ImportSourceCredentialsResponse
/**
* Imports the source repository credentials for an CodeBuild project that has its source code stored in a GitHub, GitHub Enterprise, or Bitbucket repository.
*/
suspend fun importSourceCredentials(block: ImportSourceCredentialsRequest.Builder.() -> Unit) = importSourceCredentials(ImportSourceCredentialsRequest.Builder().apply(block).build())
/**
* Resets the cache for a project.
*/
suspend fun invalidateProjectCache(input: InvalidateProjectCacheRequest): InvalidateProjectCacheResponse
/**
* Resets the cache for a project.
*/
suspend fun invalidateProjectCache(block: InvalidateProjectCacheRequest.Builder.() -> Unit) = invalidateProjectCache(InvalidateProjectCacheRequest.Builder().apply(block).build())
/**
* Retrieves the identifiers of your build batches in the current region.
*/
suspend fun listBuildBatches(input: ListBuildBatchesRequest = ListBuildBatchesRequest {}): ListBuildBatchesResponse
/**
* Retrieves the identifiers of your build batches in the current region.
*/
suspend fun listBuildBatches(block: ListBuildBatchesRequest.Builder.() -> Unit) = listBuildBatches(ListBuildBatchesRequest.Builder().apply(block).build())
/**
* Retrieves the identifiers of the build batches for a specific project.
*/
suspend fun listBuildBatchesForProject(input: ListBuildBatchesForProjectRequest = ListBuildBatchesForProjectRequest {}): ListBuildBatchesForProjectResponse
/**
* Retrieves the identifiers of the build batches for a specific project.
*/
suspend fun listBuildBatchesForProject(block: ListBuildBatchesForProjectRequest.Builder.() -> Unit) = listBuildBatchesForProject(ListBuildBatchesForProjectRequest.Builder().apply(block).build())
/**
* Gets a list of build IDs, with each build ID representing a single build.
*/
suspend fun listBuilds(input: ListBuildsRequest = ListBuildsRequest {}): ListBuildsResponse
/**
* Gets a list of build IDs, with each build ID representing a single build.
*/
suspend fun listBuilds(block: ListBuildsRequest.Builder.() -> Unit) = listBuilds(ListBuildsRequest.Builder().apply(block).build())
/**
* Gets a list of build identifiers for the specified build project, with each build identifier representing a single build.
*/
suspend fun listBuildsForProject(input: ListBuildsForProjectRequest): ListBuildsForProjectResponse
/**
* Gets a list of build identifiers for the specified build project, with each build identifier representing a single build.
*/
suspend fun listBuildsForProject(block: ListBuildsForProjectRequest.Builder.() -> Unit) = listBuildsForProject(ListBuildsForProjectRequest.Builder().apply(block).build())
/**
* Gets information about Docker images that are managed by CodeBuild.
*/
suspend fun listCuratedEnvironmentImages(input: ListCuratedEnvironmentImagesRequest = ListCuratedEnvironmentImagesRequest {}): ListCuratedEnvironmentImagesResponse
/**
* Gets information about Docker images that are managed by CodeBuild.
*/
suspend fun listCuratedEnvironmentImages(block: ListCuratedEnvironmentImagesRequest.Builder.() -> Unit) = listCuratedEnvironmentImages(ListCuratedEnvironmentImagesRequest.Builder().apply(block).build())
/**
* Gets a list of build project names, with each build project name representing a single build project.
*/
suspend fun listProjects(input: ListProjectsRequest = ListProjectsRequest {}): ListProjectsResponse
/**
* Gets a list of build project names, with each build project name representing a single build project.
*/
suspend fun listProjects(block: ListProjectsRequest.Builder.() -> Unit) = listProjects(ListProjectsRequest.Builder().apply(block).build())
/**
* Gets a list ARNs for the report groups in the current Amazon Web Services account.
*/
suspend fun listReportGroups(input: ListReportGroupsRequest = ListReportGroupsRequest {}): ListReportGroupsResponse
/**
* Gets a list ARNs for the report groups in the current Amazon Web Services account.
*/
suspend fun listReportGroups(block: ListReportGroupsRequest.Builder.() -> Unit) = listReportGroups(ListReportGroupsRequest.Builder().apply(block).build())
/**
* Returns a list of ARNs for the reports in the current Amazon Web Services account.
*/
suspend fun listReports(input: ListReportsRequest = ListReportsRequest {}): ListReportsResponse
/**
* Returns a list of ARNs for the reports in the current Amazon Web Services account.
*/
suspend fun listReports(block: ListReportsRequest.Builder.() -> Unit) = listReports(ListReportsRequest.Builder().apply(block).build())
/**
* Returns a list of ARNs for the reports that belong to a `ReportGroup`.
*/
suspend fun listReportsForReportGroup(input: ListReportsForReportGroupRequest): ListReportsForReportGroupResponse
/**
* Returns a list of ARNs for the reports that belong to a `ReportGroup`.
*/
suspend fun listReportsForReportGroup(block: ListReportsForReportGroupRequest.Builder.() -> Unit) = listReportsForReportGroup(ListReportsForReportGroupRequest.Builder().apply(block).build())
/**
* Gets a list of projects that are shared with other Amazon Web Services accounts or users.
*/
suspend fun listSharedProjects(input: ListSharedProjectsRequest = ListSharedProjectsRequest {}): ListSharedProjectsResponse
/**
* Gets a list of projects that are shared with other Amazon Web Services accounts or users.
*/
suspend fun listSharedProjects(block: ListSharedProjectsRequest.Builder.() -> Unit) = listSharedProjects(ListSharedProjectsRequest.Builder().apply(block).build())
/**
* Gets a list of report groups that are shared with other Amazon Web Services accounts or users.
*/
suspend fun listSharedReportGroups(input: ListSharedReportGroupsRequest = ListSharedReportGroupsRequest {}): ListSharedReportGroupsResponse
/**
* Gets a list of report groups that are shared with other Amazon Web Services accounts or users.
*/
suspend fun listSharedReportGroups(block: ListSharedReportGroupsRequest.Builder.() -> Unit) = listSharedReportGroups(ListSharedReportGroupsRequest.Builder().apply(block).build())
/**
* Returns a list of `SourceCredentialsInfo` objects.
*/
suspend fun listSourceCredentials(input: ListSourceCredentialsRequest = ListSourceCredentialsRequest {}): ListSourceCredentialsResponse
/**
* Returns a list of `SourceCredentialsInfo` objects.
*/
suspend fun listSourceCredentials(block: ListSourceCredentialsRequest.Builder.() -> Unit) = listSourceCredentials(ListSourceCredentialsRequest.Builder().apply(block).build())
/**
* Stores a resource policy for the ARN of a `Project` or `ReportGroup` object.
*/
suspend fun putResourcePolicy(input: PutResourcePolicyRequest): PutResourcePolicyResponse
/**
* Stores a resource policy for the ARN of a `Project` or `ReportGroup` object.
*/
suspend fun putResourcePolicy(block: PutResourcePolicyRequest.Builder.() -> Unit) = putResourcePolicy(PutResourcePolicyRequest.Builder().apply(block).build())
/**
* Restarts a build.
*/
suspend fun retryBuild(input: RetryBuildRequest = RetryBuildRequest {}): RetryBuildResponse
/**
* Restarts a build.
*/
suspend fun retryBuild(block: RetryBuildRequest.Builder.() -> Unit) = retryBuild(RetryBuildRequest.Builder().apply(block).build())
/**
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*/
suspend fun retryBuildBatch(input: RetryBuildBatchRequest = RetryBuildBatchRequest {}): RetryBuildBatchResponse
/**
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*/
suspend fun retryBuildBatch(block: RetryBuildBatchRequest.Builder.() -> Unit) = retryBuildBatch(RetryBuildBatchRequest.Builder().apply(block).build())
/**
* Starts running a build.
*/
suspend fun startBuild(input: StartBuildRequest): StartBuildResponse
/**
* Starts running a build.
*/
suspend fun startBuild(block: StartBuildRequest.Builder.() -> Unit) = startBuild(StartBuildRequest.Builder().apply(block).build())
/**
* Starts a batch build for a project.
*/
suspend fun startBuildBatch(input: StartBuildBatchRequest): StartBuildBatchResponse
/**
* Starts a batch build for a project.
*/
suspend fun startBuildBatch(block: StartBuildBatchRequest.Builder.() -> Unit) = startBuildBatch(StartBuildBatchRequest.Builder().apply(block).build())
/**
* Attempts to stop running a build.
*/
suspend fun stopBuild(input: StopBuildRequest): StopBuildResponse
/**
* Attempts to stop running a build.
*/
suspend fun stopBuild(block: StopBuildRequest.Builder.() -> Unit) = stopBuild(StopBuildRequest.Builder().apply(block).build())
/**
* Stops a running batch build.
*/
suspend fun stopBuildBatch(input: StopBuildBatchRequest): StopBuildBatchResponse
/**
* Stops a running batch build.
*/
suspend fun stopBuildBatch(block: StopBuildBatchRequest.Builder.() -> Unit) = stopBuildBatch(StopBuildBatchRequest.Builder().apply(block).build())
/**
* Changes the settings of a build project.
*/
suspend fun updateProject(input: UpdateProjectRequest): UpdateProjectResponse
/**
* Changes the settings of a build project.
*/
suspend fun updateProject(block: UpdateProjectRequest.Builder.() -> Unit) = updateProject(UpdateProjectRequest.Builder().apply(block).build())
/**
* Changes the public visibility for a project. The project's build results, logs, and artifacts are available to the general public. For more information, see [Public build projects](https://docs.aws.amazon.com/codebuild/latest/userguide/public-builds.html) in the *CodeBuild User Guide*.
*
* The following should be kept in mind when making your projects public:
* + All of a project's build results, logs, and artifacts, including builds that were run when the project was private, are available to the general public.
* + All build logs and artifacts are available to the public. Environment variables, source code, and other sensitive information may have been output to the build logs and artifacts. You must be careful about what information is output to the build logs. Some best practice are:
* + Do not store sensitive values, especially Amazon Web Services access key IDs and secret access keys, in environment variables. We recommend that you use an Amazon EC2 Systems Manager Parameter Store or Secrets Manager to store sensitive values.
* + Follow [Best practices for using webhooks](https://docs.aws.amazon.com/codebuild/latest/userguide/webhooks.html#webhook-best-practices) in the *CodeBuild User Guide* to limit which entities can trigger a build, and do not store the buildspec in the project itself, to ensure that your webhooks are as secure as possible.
* + A malicious user can use public builds to distribute malicious artifacts. We recommend that you review all pull requests to verify that the pull request is a legitimate change. We also recommend that you validate any artifacts with their checksums to make sure that the correct artifacts are being downloaded.
*/
suspend fun updateProjectVisibility(input: UpdateProjectVisibilityRequest): UpdateProjectVisibilityResponse
/**
* Changes the public visibility for a project. The project's build results, logs, and artifacts are available to the general public. For more information, see [Public build projects](https://docs.aws.amazon.com/codebuild/latest/userguide/public-builds.html) in the *CodeBuild User Guide*.
*
* The following should be kept in mind when making your projects public:
* + All of a project's build results, logs, and artifacts, including builds that were run when the project was private, are available to the general public.
* + All build logs and artifacts are available to the public. Environment variables, source code, and other sensitive information may have been output to the build logs and artifacts. You must be careful about what information is output to the build logs. Some best practice are:
* + Do not store sensitive values, especially Amazon Web Services access key IDs and secret access keys, in environment variables. We recommend that you use an Amazon EC2 Systems Manager Parameter Store or Secrets Manager to store sensitive values.
* + Follow [Best practices for using webhooks](https://docs.aws.amazon.com/codebuild/latest/userguide/webhooks.html#webhook-best-practices) in the *CodeBuild User Guide* to limit which entities can trigger a build, and do not store the buildspec in the project itself, to ensure that your webhooks are as secure as possible.
* + A malicious user can use public builds to distribute malicious artifacts. We recommend that you review all pull requests to verify that the pull request is a legitimate change. We also recommend that you validate any artifacts with their checksums to make sure that the correct artifacts are being downloaded.
*/
suspend fun updateProjectVisibility(block: UpdateProjectVisibilityRequest.Builder.() -> Unit) = updateProjectVisibility(UpdateProjectVisibilityRequest.Builder().apply(block).build())
/**
* Updates a report group.
*/
suspend fun updateReportGroup(input: UpdateReportGroupRequest): UpdateReportGroupResponse
/**
* Updates a report group.
*/
suspend fun updateReportGroup(block: UpdateReportGroupRequest.Builder.() -> Unit) = updateReportGroup(UpdateReportGroupRequest.Builder().apply(block).build())
/**
* Updates the webhook associated with an CodeBuild build project.
*
* If you use Bitbucket for your repository, `rotateSecret` is ignored.
*/
suspend fun updateWebhook(input: UpdateWebhookRequest): UpdateWebhookResponse
/**
* Updates the webhook associated with an CodeBuild build project.
*
* If you use Bitbucket for your repository, `rotateSecret` is ignored.
*/
suspend fun updateWebhook(block: UpdateWebhookRequest.Builder.() -> Unit) = updateWebhook(UpdateWebhookRequest.Builder().apply(block).build())
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy