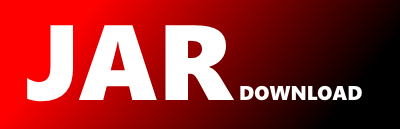
commonMain.aws.sdk.kotlin.services.codebuild.model.ImportSourceCredentialsRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild-jvm Show documentation
Show all versions of codebuild-jvm Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild.model
import aws.smithy.kotlin.runtime.SdkDsl
public class ImportSourceCredentialsRequest private constructor(builder: Builder) {
/**
* The type of authentication used to connect to a GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket repository. An OAUTH connection is not supported by the API and must be created using the CodeBuild console. Note that CODECONNECTIONS is only valid for GitLab and GitLab Self Managed.
*/
public val authType: aws.sdk.kotlin.services.codebuild.model.AuthType? = builder.authType
/**
* The source provider used for this project.
*/
public val serverType: aws.sdk.kotlin.services.codebuild.model.ServerType? = builder.serverType
/**
* Set to `false` to prevent overwriting the repository source credentials. Set to `true` to overwrite the repository source credentials. The default value is `true`.
*/
public val shouldOverwrite: kotlin.Boolean? = builder.shouldOverwrite
/**
* For GitHub or GitHub Enterprise, this is the personal access token. For Bitbucket, this is either the access token or the app password. For the `authType` CODECONNECTIONS, this is the `connectionArn`.
*/
public val token: kotlin.String? = builder.token
/**
* The Bitbucket username when the `authType` is BASIC_AUTH. This parameter is not valid for other types of source providers or connections.
*/
public val username: kotlin.String? = builder.username
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codebuild.model.ImportSourceCredentialsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ImportSourceCredentialsRequest(")
append("authType=$authType,")
append("serverType=$serverType,")
append("shouldOverwrite=$shouldOverwrite,")
append("token=*** Sensitive Data Redacted ***,")
append("username=$username")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = authType?.hashCode() ?: 0
result = 31 * result + (serverType?.hashCode() ?: 0)
result = 31 * result + (shouldOverwrite?.hashCode() ?: 0)
result = 31 * result + (token?.hashCode() ?: 0)
result = 31 * result + (username?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ImportSourceCredentialsRequest
if (authType != other.authType) return false
if (serverType != other.serverType) return false
if (shouldOverwrite != other.shouldOverwrite) return false
if (token != other.token) return false
if (username != other.username) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codebuild.model.ImportSourceCredentialsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The type of authentication used to connect to a GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket repository. An OAUTH connection is not supported by the API and must be created using the CodeBuild console. Note that CODECONNECTIONS is only valid for GitLab and GitLab Self Managed.
*/
public var authType: aws.sdk.kotlin.services.codebuild.model.AuthType? = null
/**
* The source provider used for this project.
*/
public var serverType: aws.sdk.kotlin.services.codebuild.model.ServerType? = null
/**
* Set to `false` to prevent overwriting the repository source credentials. Set to `true` to overwrite the repository source credentials. The default value is `true`.
*/
public var shouldOverwrite: kotlin.Boolean? = null
/**
* For GitHub or GitHub Enterprise, this is the personal access token. For Bitbucket, this is either the access token or the app password. For the `authType` CODECONNECTIONS, this is the `connectionArn`.
*/
public var token: kotlin.String? = null
/**
* The Bitbucket username when the `authType` is BASIC_AUTH. This parameter is not valid for other types of source providers or connections.
*/
public var username: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codebuild.model.ImportSourceCredentialsRequest) : this() {
this.authType = x.authType
this.serverType = x.serverType
this.shouldOverwrite = x.shouldOverwrite
this.token = x.token
this.username = x.username
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codebuild.model.ImportSourceCredentialsRequest = ImportSourceCredentialsRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy