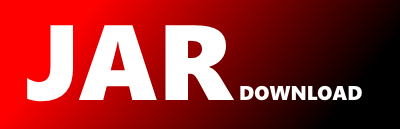
commonMain.aws.sdk.kotlin.services.codebuild.model.TestCase.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information about a test case created using a framework such as NUnit or Cucumber. A test case might be a unit test or a configuration test.
*/
public class TestCase private constructor(builder: Builder) {
/**
* The number of nanoseconds it took to run this test case.
*/
public val durationInNanoSeconds: kotlin.Long? = builder.durationInNanoSeconds
/**
* The date and time a test case expires. A test case expires 30 days after it is created. An expired test case is not available to view in CodeBuild.
*/
public val expired: aws.smithy.kotlin.runtime.time.Instant? = builder.expired
/**
* A message associated with a test case. For example, an error message or stack trace.
*/
public val message: kotlin.String? = builder.message
/**
* The name of the test case.
*/
public val name: kotlin.String? = builder.name
/**
* A string that is applied to a series of related test cases. CodeBuild generates the prefix. The prefix depends on the framework used to generate the tests.
*/
public val prefix: kotlin.String? = builder.prefix
/**
* The ARN of the report to which the test case belongs.
*/
public val reportArn: kotlin.String? = builder.reportArn
/**
* The status returned by the test case after it was run. Valid statuses are `SUCCEEDED`, `FAILED`, `ERROR`, `SKIPPED`, and `UNKNOWN`.
*/
public val status: kotlin.String? = builder.status
/**
* The path to the raw data file that contains the test result.
*/
public val testRawDataPath: kotlin.String? = builder.testRawDataPath
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codebuild.model.TestCase = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TestCase(")
append("durationInNanoSeconds=$durationInNanoSeconds,")
append("expired=$expired,")
append("message=$message,")
append("name=$name,")
append("prefix=$prefix,")
append("reportArn=$reportArn,")
append("status=$status,")
append("testRawDataPath=$testRawDataPath")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = durationInNanoSeconds?.hashCode() ?: 0
result = 31 * result + (expired?.hashCode() ?: 0)
result = 31 * result + (message?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (prefix?.hashCode() ?: 0)
result = 31 * result + (reportArn?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (testRawDataPath?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TestCase
if (durationInNanoSeconds != other.durationInNanoSeconds) return false
if (expired != other.expired) return false
if (message != other.message) return false
if (name != other.name) return false
if (prefix != other.prefix) return false
if (reportArn != other.reportArn) return false
if (status != other.status) return false
if (testRawDataPath != other.testRawDataPath) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codebuild.model.TestCase = Builder(this).apply(block).build()
public class Builder {
/**
* The number of nanoseconds it took to run this test case.
*/
public var durationInNanoSeconds: kotlin.Long? = null
/**
* The date and time a test case expires. A test case expires 30 days after it is created. An expired test case is not available to view in CodeBuild.
*/
public var expired: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A message associated with a test case. For example, an error message or stack trace.
*/
public var message: kotlin.String? = null
/**
* The name of the test case.
*/
public var name: kotlin.String? = null
/**
* A string that is applied to a series of related test cases. CodeBuild generates the prefix. The prefix depends on the framework used to generate the tests.
*/
public var prefix: kotlin.String? = null
/**
* The ARN of the report to which the test case belongs.
*/
public var reportArn: kotlin.String? = null
/**
* The status returned by the test case after it was run. Valid statuses are `SUCCEEDED`, `FAILED`, `ERROR`, `SKIPPED`, and `UNKNOWN`.
*/
public var status: kotlin.String? = null
/**
* The path to the raw data file that contains the test result.
*/
public var testRawDataPath: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codebuild.model.TestCase) : this() {
this.durationInNanoSeconds = x.durationInNanoSeconds
this.expired = x.expired
this.message = x.message
this.name = x.name
this.prefix = x.prefix
this.reportArn = x.reportArn
this.status = x.status
this.testRawDataPath = x.testRawDataPath
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codebuild.model.TestCase = TestCase(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy