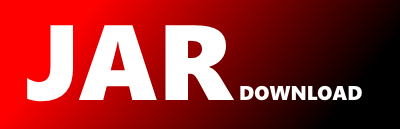
commonMain.aws.sdk.kotlin.services.codebuild.model.BuildSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Contains summary information about a batch build group.
*/
public class BuildSummary private constructor(builder: Builder) {
/**
* The batch build ARN.
*/
public val arn: kotlin.String? = builder.arn
/**
* The status of the build group.
*
* ## FAILED
* The build group failed.
*
* ## FAULT
* The build group faulted.
*
* ## IN_PROGRESS
* The build group is still in progress.
*
* ## STOPPED
* The build group stopped.
*
* ## SUCCEEDED
* The build group succeeded.
*
* ## TIMED_OUT
* The build group timed out.
*/
public val buildStatus: aws.sdk.kotlin.services.codebuild.model.StatusType? = builder.buildStatus
/**
* A `ResolvedArtifact` object that represents the primary build artifacts for the build group.
*/
public val primaryArtifact: aws.sdk.kotlin.services.codebuild.model.ResolvedArtifact? = builder.primaryArtifact
/**
* When the build was started, expressed in Unix time format.
*/
public val requestedOn: aws.smithy.kotlin.runtime.time.Instant? = builder.requestedOn
/**
* An array of `ResolvedArtifact` objects that represents the secondary build artifacts for the build group.
*/
public val secondaryArtifacts: List? = builder.secondaryArtifacts
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codebuild.model.BuildSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("BuildSummary(")
append("arn=$arn,")
append("buildStatus=$buildStatus,")
append("primaryArtifact=$primaryArtifact,")
append("requestedOn=$requestedOn,")
append("secondaryArtifacts=$secondaryArtifacts")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (buildStatus?.hashCode() ?: 0)
result = 31 * result + (primaryArtifact?.hashCode() ?: 0)
result = 31 * result + (requestedOn?.hashCode() ?: 0)
result = 31 * result + (secondaryArtifacts?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as BuildSummary
if (arn != other.arn) return false
if (buildStatus != other.buildStatus) return false
if (primaryArtifact != other.primaryArtifact) return false
if (requestedOn != other.requestedOn) return false
if (secondaryArtifacts != other.secondaryArtifacts) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codebuild.model.BuildSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The batch build ARN.
*/
public var arn: kotlin.String? = null
/**
* The status of the build group.
*
* ## FAILED
* The build group failed.
*
* ## FAULT
* The build group faulted.
*
* ## IN_PROGRESS
* The build group is still in progress.
*
* ## STOPPED
* The build group stopped.
*
* ## SUCCEEDED
* The build group succeeded.
*
* ## TIMED_OUT
* The build group timed out.
*/
public var buildStatus: aws.sdk.kotlin.services.codebuild.model.StatusType? = null
/**
* A `ResolvedArtifact` object that represents the primary build artifacts for the build group.
*/
public var primaryArtifact: aws.sdk.kotlin.services.codebuild.model.ResolvedArtifact? = null
/**
* When the build was started, expressed in Unix time format.
*/
public var requestedOn: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* An array of `ResolvedArtifact` objects that represents the secondary build artifacts for the build group.
*/
public var secondaryArtifacts: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codebuild.model.BuildSummary) : this() {
this.arn = x.arn
this.buildStatus = x.buildStatus
this.primaryArtifact = x.primaryArtifact
this.requestedOn = x.requestedOn
this.secondaryArtifacts = x.secondaryArtifacts
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codebuild.model.BuildSummary = BuildSummary(this)
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.ResolvedArtifact] inside the given [block]
*/
public fun primaryArtifact(block: aws.sdk.kotlin.services.codebuild.model.ResolvedArtifact.Builder.() -> kotlin.Unit) {
this.primaryArtifact = aws.sdk.kotlin.services.codebuild.model.ResolvedArtifact.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy