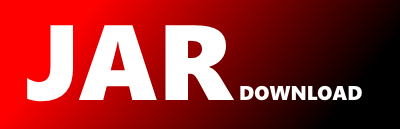
commonMain.aws.sdk.kotlin.services.codebuild.model.CreateWebhookRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild.model
public class CreateWebhookRequest private constructor(builder: Builder) {
/**
* A regular expression used to determine which repository branches are built when a webhook is triggered. If the name of a branch matches the regular expression, then it is built. If `branchFilter` is empty, then all branches are built.
*
* It is recommended that you use `filterGroups` instead of `branchFilter`.
*/
public val branchFilter: kotlin.String? = builder.branchFilter
/**
* Specifies the type of build this webhook will trigger.
*/
public val buildType: aws.sdk.kotlin.services.codebuild.model.WebhookBuildType? = builder.buildType
/**
* An array of arrays of `WebhookFilter` objects used to determine which webhooks are triggered. At least one `WebhookFilter` in the array must specify `EVENT` as its `type`.
*
* For a build to be triggered, at least one filter group in the `filterGroups` array must pass. For a filter group to pass, each of its filters must pass.
*/
public val filterGroups: List>? = builder.filterGroups
/**
* The name of the CodeBuild project.
*/
public val projectName: kotlin.String? = builder.projectName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codebuild.model.CreateWebhookRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateWebhookRequest(")
append("branchFilter=$branchFilter,")
append("buildType=$buildType,")
append("filterGroups=$filterGroups,")
append("projectName=$projectName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = branchFilter?.hashCode() ?: 0
result = 31 * result + (buildType?.hashCode() ?: 0)
result = 31 * result + (filterGroups?.hashCode() ?: 0)
result = 31 * result + (projectName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateWebhookRequest
if (branchFilter != other.branchFilter) return false
if (buildType != other.buildType) return false
if (filterGroups != other.filterGroups) return false
if (projectName != other.projectName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codebuild.model.CreateWebhookRequest = Builder(this).apply(block).build()
public class Builder {
/**
* A regular expression used to determine which repository branches are built when a webhook is triggered. If the name of a branch matches the regular expression, then it is built. If `branchFilter` is empty, then all branches are built.
*
* It is recommended that you use `filterGroups` instead of `branchFilter`.
*/
public var branchFilter: kotlin.String? = null
/**
* Specifies the type of build this webhook will trigger.
*/
public var buildType: aws.sdk.kotlin.services.codebuild.model.WebhookBuildType? = null
/**
* An array of arrays of `WebhookFilter` objects used to determine which webhooks are triggered. At least one `WebhookFilter` in the array must specify `EVENT` as its `type`.
*
* For a build to be triggered, at least one filter group in the `filterGroups` array must pass. For a filter group to pass, each of its filters must pass.
*/
public var filterGroups: List>? = null
/**
* The name of the CodeBuild project.
*/
public var projectName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codebuild.model.CreateWebhookRequest) : this() {
this.branchFilter = x.branchFilter
this.buildType = x.buildType
this.filterGroups = x.filterGroups
this.projectName = x.projectName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codebuild.model.CreateWebhookRequest = CreateWebhookRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy