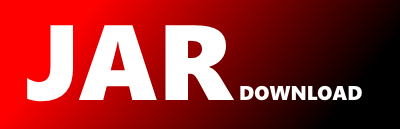
aws.sdk.kotlin.services.codebuild.DefaultCodeBuildClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild
import aws.sdk.kotlin.runtime.auth.signing.AwsSigV4SigningMiddleware
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.execution.AuthAttributes
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.engine.crt.CrtHttpEngine
import aws.sdk.kotlin.runtime.http.middleware.ResolveAwsEndpoint
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.runtime.http.retries.AwsDefaultRetryPolicy
import aws.sdk.kotlin.runtime.protocol.json.AwsJsonProtocol
import aws.sdk.kotlin.services.codebuild.model.*
import aws.sdk.kotlin.services.codebuild.transform.*
import aws.smithy.kotlin.runtime.client.ExecutionContext
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.middleware.Retry
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.sdkHttpClient
import aws.smithy.kotlin.runtime.util.putIfAbsent
const val ServiceId: String = "CodeBuild"
const val ServiceApiVersion: String = "2016-10-06"
const val SdkVersion: String = "0.9.5-beta"
internal class DefaultCodeBuildClient(override val config: CodeBuildClient.Config) : CodeBuildClient {
private val client: SdkHttpClient
init {
val httpClientEngine = config.httpClientEngine ?: CrtHttpEngine()
client = sdkHttpClient(httpClientEngine, manageEngine = config.httpClientEngine == null)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion))
/**
* Deletes one or more builds.
*/
override suspend fun batchDeleteBuilds(input: BatchDeleteBuildsRequest): BatchDeleteBuildsResponse {
val op = SdkHttpOperation.build {
serializer = BatchDeleteBuildsOperationSerializer()
deserializer = BatchDeleteBuildsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchDeleteBuilds"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Retrieves information about one or more batch builds.
*/
override suspend fun batchGetBuildBatches(input: BatchGetBuildBatchesRequest): BatchGetBuildBatchesResponse {
val op = SdkHttpOperation.build {
serializer = BatchGetBuildBatchesOperationSerializer()
deserializer = BatchGetBuildBatchesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchGetBuildBatches"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets information about one or more builds.
*/
override suspend fun batchGetBuilds(input: BatchGetBuildsRequest): BatchGetBuildsResponse {
val op = SdkHttpOperation.build {
serializer = BatchGetBuildsOperationSerializer()
deserializer = BatchGetBuildsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchGetBuilds"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets information about one or more build projects.
*/
override suspend fun batchGetProjects(input: BatchGetProjectsRequest): BatchGetProjectsResponse {
val op = SdkHttpOperation.build {
serializer = BatchGetProjectsOperationSerializer()
deserializer = BatchGetProjectsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchGetProjects"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns an array of report groups.
*/
override suspend fun batchGetReportGroups(input: BatchGetReportGroupsRequest): BatchGetReportGroupsResponse {
val op = SdkHttpOperation.build {
serializer = BatchGetReportGroupsOperationSerializer()
deserializer = BatchGetReportGroupsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchGetReportGroups"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns an array of reports.
*/
override suspend fun batchGetReports(input: BatchGetReportsRequest): BatchGetReportsResponse {
val op = SdkHttpOperation.build {
serializer = BatchGetReportsOperationSerializer()
deserializer = BatchGetReportsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "BatchGetReports"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Creates a build project.
*/
override suspend fun createProject(input: CreateProjectRequest): CreateProjectResponse {
val op = SdkHttpOperation.build {
serializer = CreateProjectOperationSerializer()
deserializer = CreateProjectOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateProject"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Creates a report group. A report group contains a collection of reports.
*/
override suspend fun createReportGroup(input: CreateReportGroupRequest): CreateReportGroupResponse {
val op = SdkHttpOperation.build {
serializer = CreateReportGroupOperationSerializer()
deserializer = CreateReportGroupOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateReportGroup"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or
* Bitbucket repository, enables CodeBuild to start rebuilding the source code every time a
* code change is pushed to the repository.
* If you enable webhooks for an CodeBuild project, and the project is used as a build
* step in CodePipeline, then two identical builds are created for each commit. One build is
* triggered through webhooks, and one through CodePipeline. Because billing is on a per-build
* basis, you are billed for both builds. Therefore, if you are using CodePipeline, we
* recommend that you disable webhooks in CodeBuild. In the CodeBuild console, clear the
* Webhook box. For more information, see step 5 in Change a Build Project's Settings.
*/
override suspend fun createWebhook(input: CreateWebhookRequest): CreateWebhookResponse {
val op = SdkHttpOperation.build {
serializer = CreateWebhookOperationSerializer()
deserializer = CreateWebhookOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateWebhook"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a batch build.
*/
override suspend fun deleteBuildBatch(input: DeleteBuildBatchRequest): DeleteBuildBatchResponse {
val op = SdkHttpOperation.build {
serializer = DeleteBuildBatchOperationSerializer()
deserializer = DeleteBuildBatchOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteBuildBatch"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a build project. When you delete a project, its builds are not deleted.
*/
override suspend fun deleteProject(input: DeleteProjectRequest): DeleteProjectResponse {
val op = SdkHttpOperation.build {
serializer = DeleteProjectOperationSerializer()
deserializer = DeleteProjectOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteProject"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a report.
*/
override suspend fun deleteReport(input: DeleteReportRequest): DeleteReportResponse {
val op = SdkHttpOperation.build {
serializer = DeleteReportOperationSerializer()
deserializer = DeleteReportOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteReport"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a report group. Before you delete a report group, you must delete its reports.
*/
override suspend fun deleteReportGroup(input: DeleteReportGroupRequest): DeleteReportGroupResponse {
val op = SdkHttpOperation.build {
serializer = DeleteReportGroupOperationSerializer()
deserializer = DeleteReportGroupOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteReportGroup"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a resource policy that is identified by its resource ARN.
*/
override suspend fun deleteResourcePolicy(input: DeleteResourcePolicyRequest): DeleteResourcePolicyResponse {
val op = SdkHttpOperation.build {
serializer = DeleteResourcePolicyOperationSerializer()
deserializer = DeleteResourcePolicyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteResourcePolicy"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*/
override suspend fun deleteSourceCredentials(input: DeleteSourceCredentialsRequest): DeleteSourceCredentialsResponse {
val op = SdkHttpOperation.build {
serializer = DeleteSourceCredentialsOperationSerializer()
deserializer = DeleteSourceCredentialsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteSourceCredentials"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* For an existing CodeBuild build project that has its source code stored in a GitHub or
* Bitbucket repository, stops CodeBuild from rebuilding the source code every time a code
* change is pushed to the repository.
*/
override suspend fun deleteWebhook(input: DeleteWebhookRequest): DeleteWebhookResponse {
val op = SdkHttpOperation.build {
serializer = DeleteWebhookOperationSerializer()
deserializer = DeleteWebhookOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteWebhook"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Retrieves one or more code coverage reports.
*/
override suspend fun describeCodeCoverages(input: DescribeCodeCoveragesRequest): DescribeCodeCoveragesResponse {
val op = SdkHttpOperation.build {
serializer = DescribeCodeCoveragesOperationSerializer()
deserializer = DescribeCodeCoveragesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DescribeCodeCoverages"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns a list of details about test cases for a report.
*/
override suspend fun describeTestCases(input: DescribeTestCasesRequest): DescribeTestCasesResponse {
val op = SdkHttpOperation.build {
serializer = DescribeTestCasesOperationSerializer()
deserializer = DescribeTestCasesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DescribeTestCases"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Analyzes and accumulates test report values for the specified test reports.
*/
override suspend fun getReportGroupTrend(input: GetReportGroupTrendRequest): GetReportGroupTrendResponse {
val op = SdkHttpOperation.build {
serializer = GetReportGroupTrendOperationSerializer()
deserializer = GetReportGroupTrendOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetReportGroupTrend"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a resource policy that is identified by its resource ARN.
*/
override suspend fun getResourcePolicy(input: GetResourcePolicyRequest): GetResourcePolicyResponse {
val op = SdkHttpOperation.build {
serializer = GetResourcePolicyOperationSerializer()
deserializer = GetResourcePolicyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetResourcePolicy"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Imports the source repository credentials for an CodeBuild project that has its
* source code stored in a GitHub, GitHub Enterprise, or Bitbucket repository.
*/
override suspend fun importSourceCredentials(input: ImportSourceCredentialsRequest): ImportSourceCredentialsResponse {
val op = SdkHttpOperation.build {
serializer = ImportSourceCredentialsOperationSerializer()
deserializer = ImportSourceCredentialsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ImportSourceCredentials"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Resets the cache for a project.
*/
override suspend fun invalidateProjectCache(input: InvalidateProjectCacheRequest): InvalidateProjectCacheResponse {
val op = SdkHttpOperation.build {
serializer = InvalidateProjectCacheOperationSerializer()
deserializer = InvalidateProjectCacheOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "InvalidateProjectCache"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Retrieves the identifiers of your build batches in the current region.
*/
override suspend fun listBuildBatches(input: ListBuildBatchesRequest): ListBuildBatchesResponse {
val op = SdkHttpOperation.build {
serializer = ListBuildBatchesOperationSerializer()
deserializer = ListBuildBatchesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListBuildBatches"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Retrieves the identifiers of the build batches for a specific project.
*/
override suspend fun listBuildBatchesForProject(input: ListBuildBatchesForProjectRequest): ListBuildBatchesForProjectResponse {
val op = SdkHttpOperation.build {
serializer = ListBuildBatchesForProjectOperationSerializer()
deserializer = ListBuildBatchesForProjectOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListBuildBatchesForProject"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list of build IDs, with each build ID representing a single build.
*/
override suspend fun listBuilds(input: ListBuildsRequest): ListBuildsResponse {
val op = SdkHttpOperation.build {
serializer = ListBuildsOperationSerializer()
deserializer = ListBuildsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListBuilds"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list of build identifiers for the specified build project, with each build
* identifier representing a single build.
*/
override suspend fun listBuildsForProject(input: ListBuildsForProjectRequest): ListBuildsForProjectResponse {
val op = SdkHttpOperation.build {
serializer = ListBuildsForProjectOperationSerializer()
deserializer = ListBuildsForProjectOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListBuildsForProject"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets information about Docker images that are managed by CodeBuild.
*/
override suspend fun listCuratedEnvironmentImages(input: ListCuratedEnvironmentImagesRequest): ListCuratedEnvironmentImagesResponse {
val op = SdkHttpOperation.build {
serializer = ListCuratedEnvironmentImagesOperationSerializer()
deserializer = ListCuratedEnvironmentImagesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListCuratedEnvironmentImages"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list of build project names, with each build project name representing a single
* build project.
*/
override suspend fun listProjects(input: ListProjectsRequest): ListProjectsResponse {
val op = SdkHttpOperation.build {
serializer = ListProjectsOperationSerializer()
deserializer = ListProjectsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListProjects"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list ARNs for the report groups in the current Amazon Web Services account.
*/
override suspend fun listReportGroups(input: ListReportGroupsRequest): ListReportGroupsResponse {
val op = SdkHttpOperation.build {
serializer = ListReportGroupsOperationSerializer()
deserializer = ListReportGroupsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListReportGroups"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns a list of ARNs for the reports in the current Amazon Web Services account.
*/
override suspend fun listReports(input: ListReportsRequest): ListReportsResponse {
val op = SdkHttpOperation.build {
serializer = ListReportsOperationSerializer()
deserializer = ListReportsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListReports"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns a list of ARNs for the reports that belong to a ReportGroup.
*/
override suspend fun listReportsForReportGroup(input: ListReportsForReportGroupRequest): ListReportsForReportGroupResponse {
val op = SdkHttpOperation.build {
serializer = ListReportsForReportGroupOperationSerializer()
deserializer = ListReportsForReportGroupOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListReportsForReportGroup"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list of projects that are shared with other Amazon Web Services accounts or users.
*/
override suspend fun listSharedProjects(input: ListSharedProjectsRequest): ListSharedProjectsResponse {
val op = SdkHttpOperation.build {
serializer = ListSharedProjectsOperationSerializer()
deserializer = ListSharedProjectsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListSharedProjects"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Gets a list of report groups that are shared with other Amazon Web Services accounts or users.
*/
override suspend fun listSharedReportGroups(input: ListSharedReportGroupsRequest): ListSharedReportGroupsResponse {
val op = SdkHttpOperation.build {
serializer = ListSharedReportGroupsOperationSerializer()
deserializer = ListSharedReportGroupsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListSharedReportGroups"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Returns a list of SourceCredentialsInfo objects.
*/
override suspend fun listSourceCredentials(input: ListSourceCredentialsRequest): ListSourceCredentialsResponse {
val op = SdkHttpOperation.build {
serializer = ListSourceCredentialsOperationSerializer()
deserializer = ListSourceCredentialsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListSourceCredentials"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Stores a resource policy for the ARN of a Project or
* ReportGroup object.
*/
override suspend fun putResourcePolicy(input: PutResourcePolicyRequest): PutResourcePolicyResponse {
val op = SdkHttpOperation.build {
serializer = PutResourcePolicyOperationSerializer()
deserializer = PutResourcePolicyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "PutResourcePolicy"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Restarts a build.
*/
override suspend fun retryBuild(input: RetryBuildRequest): RetryBuildResponse {
val op = SdkHttpOperation.build {
serializer = RetryBuildOperationSerializer()
deserializer = RetryBuildOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "RetryBuild"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*/
override suspend fun retryBuildBatch(input: RetryBuildBatchRequest): RetryBuildBatchResponse {
val op = SdkHttpOperation.build {
serializer = RetryBuildBatchOperationSerializer()
deserializer = RetryBuildBatchOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "RetryBuildBatch"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Starts running a build.
*/
override suspend fun startBuild(input: StartBuildRequest): StartBuildResponse {
val op = SdkHttpOperation.build {
serializer = StartBuildOperationSerializer()
deserializer = StartBuildOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StartBuild"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Starts a batch build for a project.
*/
override suspend fun startBuildBatch(input: StartBuildBatchRequest): StartBuildBatchResponse {
val op = SdkHttpOperation.build {
serializer = StartBuildBatchOperationSerializer()
deserializer = StartBuildBatchOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StartBuildBatch"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Attempts to stop running a build.
*/
override suspend fun stopBuild(input: StopBuildRequest): StopBuildResponse {
val op = SdkHttpOperation.build {
serializer = StopBuildOperationSerializer()
deserializer = StopBuildOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StopBuild"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Stops a running batch build.
*/
override suspend fun stopBuildBatch(input: StopBuildBatchRequest): StopBuildBatchResponse {
val op = SdkHttpOperation.build {
serializer = StopBuildBatchOperationSerializer()
deserializer = StopBuildBatchOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StopBuildBatch"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Changes the settings of a build project.
*/
override suspend fun updateProject(input: UpdateProjectRequest): UpdateProjectResponse {
val op = SdkHttpOperation.build {
serializer = UpdateProjectOperationSerializer()
deserializer = UpdateProjectOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateProject"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Changes the public visibility for a project. The project's build results, logs, and
* artifacts are available to the general public. For more information, see Public build
* projects in the CodeBuild User Guide.
* The following should be kept in mind when making your projects public:
* All of a project's build results, logs, and artifacts, including builds that were run
* when the project was private, are available to the general public.
* All build logs and artifacts are available to the public. Environment variables, source
* code, and other sensitive information may have been output to the build logs and artifacts.
* You must be careful about what information is output to the build logs. Some best practice
* are:
* Do not store sensitive values, especially Amazon Web Services access key IDs and secret access
* keys, in environment variables. We recommend that you use an Amazon EC2 Systems Manager Parameter Store
* or Secrets Manager to store sensitive values.
* Follow Best
* practices for using webhooks in the CodeBuild User
* Guide to limit which entities can trigger a build, and do
* not store the buildspec in the project itself, to ensure that your webhooks are as
* secure as possible.
* A malicious user can use public builds to distribute malicious artifacts. We recommend
* that you review all pull requests to verify that the pull request is a legitimate change. We
* also recommend that you validate any artifacts with their checksums to make sure that the
* correct artifacts are being downloaded.
*/
override suspend fun updateProjectVisibility(input: UpdateProjectVisibilityRequest): UpdateProjectVisibilityResponse {
val op = SdkHttpOperation.build {
serializer = UpdateProjectVisibilityOperationSerializer()
deserializer = UpdateProjectVisibilityOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateProjectVisibility"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Updates a report group.
*/
override suspend fun updateReportGroup(input: UpdateReportGroupRequest): UpdateReportGroupResponse {
val op = SdkHttpOperation.build {
serializer = UpdateReportGroupOperationSerializer()
deserializer = UpdateReportGroupOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateReportGroup"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
/**
* Updates the webhook associated with an CodeBuild build project.
* If you use Bitbucket for your repository, rotateSecret is ignored.
*/
override suspend fun updateWebhook(input: UpdateWebhookRequest): UpdateWebhookResponse {
val op = SdkHttpOperation.build {
serializer = UpdateWebhookOperationSerializer()
deserializer = UpdateWebhookOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateWebhook"
}
}
mergeServiceDefaults(op.context)
op.install(ResolveAwsEndpoint(ServiceId, config.endpointResolver))
op.install(Retry(config.retryStrategy, AwsDefaultRetryPolicy))
op.install(AwsJsonProtocol("CodeBuild_20161006", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(
AwsSigV4SigningMiddleware{
this.credentialsProvider = config.credentialsProvider
this.signingService = "codebuild"
}
)
return op.roundTrip(client, input)
}
override fun close() {
client.close()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private suspend fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(AwsClientOption.Region, config.region)
ctx.putIfAbsent(AuthAttributes.SigningRegion, config.region)
ctx.putIfAbsent(SdkClientOption.ServiceName, serviceName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.sdkLogMode)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy